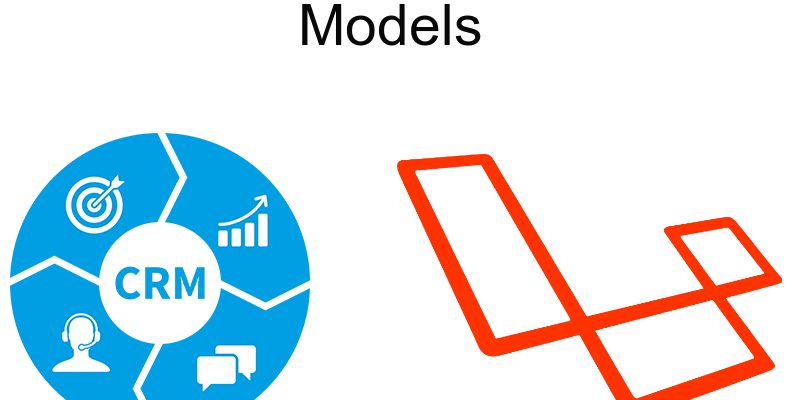
In this part of the series (Implementing CRM with laravel) we will generate the eloquent models and setup up relations between tables.
Series Topics:
- Part 1: Preparation
- Part 2: Database
- Part 3: Models & Relations
- Part 4: Preparing Login Page
- Part 5: Users Module
- Part 6: Roles & Permissions
- Part 7: Documents Module
- Part 8: Contacts Module
- Part 9: Tasks Module
- Part 10: Mailbox Module
- Part 11: Mailbox Module Complete
- Part 12: Calendar Module
- Part 13: Finishing
Let’s generate the eloquent models using the laravel artisan commands, at first create a new directory inside the app/ directory called “Models“. This directory will contains our models, next run these commands one after the other:
php artisan make:model Models/Contact php artisan make:model Models/ContactDocument php artisan make:model Models/ContactEmail php artisan make:model Models/ContactPhone php artisan make:model Models/ContactStatus php artisan make:model Models/Document php artisan make:model Models/DocumentType php artisan make:model Models/Setting php artisan make:model Models/Task php artisan make:model Models/TaskDocument php artisan make:model Models/TaskStatus php artisan make:model Models/TaskType
Now after we generated the models we will list all the model code below along with the relations according to the Database Diagram that we created in the previous part2.
app/Models/Contact.php
<?php namespace App\Models; use App\User; use Illuminate\Database\Eloquent\Model; use Illuminate\Database\Eloquent\SoftDeletes; class Contact extends Model { use SoftDeletes; public $timestamps = true; protected $table = "contact"; protected $fillable = ["first_name", "middle_name", "last_name", "status", "referral_source", "position_title", "industry", "project_type", "company", "project_description", "description", "budget", "website", "linkedin", "address_street", "address_city", "address_state", "address_country", "address_zipcode", "created_by_id", "modified_by_id", "assigned_user_id"]; /** * get created by user object * * @return \Illuminate\Database\Eloquent\Relations\BelongsTo */ public function createdBy() { return $this->belongsTo(User::class, 'created_by_id'); } /** * get modified by user object * * @return \Illuminate\Database\Eloquent\Relations\BelongsTo */ public function modifiedBy() { return $this->belongsTo(User::class, 'modified_by_id'); } /** * get assigned to user object * * @return \Illuminate\Database\Eloquent\Relations\BelongsTo */ public function assignedTo() { return $this->belongsTo(User::class, 'assigned_user_id'); } /** * get status object of this contact i.e lead, opportunity, etc. * * @return \Illuminate\Database\Eloquent\Relations\BelongsTo */ public function getStatus() { return $this->belongsTo(ContactStatus::class, 'status'); } /** * get all documents for this contact (this relation is a many to many) * * @return \Illuminate\Database\Eloquent\Relations\BelongsToMany */ public function documents() { return $this->belongsToMany(Document::class, 'contact_document', 'contact_id', 'document_id'); } /** * get all emails for this contact * * @return \Illuminate\Database\Eloquent\Relations\HasMany */ public function emails() { return $this->hasMany(ContactEmail::class, 'contact_id'); } /** * get all phones for this contact * * @return \Illuminate\Database\Eloquent\Relations\HasMany */ public function phones() { return $this->hasMany(ContactPhone::class, 'contact_id'); } /** * get all tasks related to this contact * * @return \Illuminate\Database\Eloquent\Relations\HasMany */ public function tasks() { return $this->hasMany(Task::class, 'contact_id'); } public function getName() { return $this->first_name . (!empty($this->middle_name)?" " . $this->middle_name . " ":"") . (!empty($this->last_name)?" " . $this->last_name:""); } }
app/Models/ContactDocument.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Model; class ContactDocument extends Model { protected $table = "contact_document"; protected $fillable = ["contact_id", "document_id"]; }
app/Models/ContactEmail.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Model; class ContactEmail extends Model { protected $table = "contact_email"; protected $fillable = ["email", "contact_id"]; }
app/Models/ContactPhone.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Model; class ContactPhone extends Model { protected $table = "contact_phone"; protected $fillable = ["phone", "contact_id"]; }
app/Models/ContactStatus.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Model; class ContactStatus extends Model { protected $table = "contact_status"; protected $fillable = ["name"]; }
app/Models/Document.php
<?php namespace App\Models; use App\User; use Illuminate\Database\Eloquent\Model; use Illuminate\Database\Eloquent\SoftDeletes; class Document extends Model { use SoftDeletes; protected $table = "document"; public $timestamps = true; protected $fillable = ["name", "file", "status", "type", "publish_date", "expiration_date", "created_by_id", "modified_by_id", "assigned_user_id"]; /** * get created by user object * * @return \Illuminate\Database\Eloquent\Relations\BelongsTo */ public function createdBy() { return $this->belongsTo(User::class, 'created_by_id'); } /** * get modified by user object * * @return \Illuminate\Database\Eloquent\Relations\BelongsTo */ public function modifiedBy() { return $this->belongsTo(User::class, 'modified_by_id'); } /** * get assigned to user object * * @return \Illuminate\Database\Eloquent\Relations\BelongsTo */ public function assignedTo() { return $this->belongsTo(User::class, 'assigned_user_id'); } /** * get type object for this document * * @return \Illuminate\Database\Eloquent\Relations\BelongsTo */ public function getType() { return $this->belongsTo(DocumentType::class, 'type'); } }
app/Models/DocumentType.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Model; class DocumentType extends Model { protected $table = "document_type"; protected $fillable = ["name"]; }
app/Models/Setting.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Model; class Setting extends Model { protected $table = "setting"; protected $fillable = ["setting_key", "setting_value"]; }
app/Models/Task.php
<?php namespace App\Models; use App\User; use Illuminate\Database\Eloquent\Model; use Illuminate\Database\Eloquent\SoftDeletes; class Task extends Model { use SoftDeletes; public $timestamps = true; protected $table = "task"; protected $fillable = ["name", "priority", "status", "type_id", "start_date", "end_date", "complete_date", "contact_type", "contact_id", "description", "created_by_id", "modified_by_id", "assigned_user_id"]; /** * get created by user object * * @return \Illuminate\Database\Eloquent\Relations\BelongsTo */ public function createdBy() { return $this->belongsTo(User::class, 'created_by_id'); } /** * get modified by user object * * @return \Illuminate\Database\Eloquent\Relations\BelongsTo */ public function modifiedBy() { return $this->belongsTo(User::class, 'modified_by_id'); } /** * get assigned to user object * * @return \Illuminate\Database\Eloquent\Relations\BelongsTo */ public function assignedTo() { return $this->belongsTo(User::class, 'assigned_user_id'); } /** * get status object for this task i.e completed, started, etc. * * @return \Illuminate\Database\Eloquent\Relations\BelongsTo */ public function getStatus() { return $this->belongsTo(TaskStatus::class, 'status'); } /** * get type object for this task * * @return \Illuminate\Database\Eloquent\Relations\BelongsTo */ public function type() { return $this->belongsTo(TaskType::class, 'type_id'); } /** * get contact object attached with this task * * @return \Illuminate\Database\Eloquent\Relations\BelongsTo */ public function contact() { return $this->belongsTo(Contact::class, 'contact_id'); } /** * get all documents for this task (this is a many to many relation) * * @return \Illuminate\Database\Eloquent\Relations\BelongsToMany */ public function documents() { return $this->belongsToMany(Document::class, 'task_document', 'task_id', 'document_id'); } }
app/Models/TaskDocument.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Model; class TaskDocument extends Model { protected $table = "task_document"; protected $fillable = ["task_id", "document_id"]; }
app/Models/TaskStatus.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Model; class TaskStatus extends Model { protected $table = "task_status"; protected $fillable = ["name"]; }
app/Models/TaskType.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Model; class TaskType extends Model { protected $table = "task_type"; protected $fillable = ["name"]; }
app/User.php
<?php namespace App; use App\Models\Contact; use App\Models\ContactStatus; use App\Models\Document; use App\Models\Task; use App\Models\TaskStatus; use Illuminate\Notifications\Notifiable; use Illuminate\Foundation\Auth\User as Authenticatable; class User extends Authenticatable { use Notifiable; /** * The attributes that are mass assignable. * * @var array */ protected $fillable = [ 'name', 'email', 'password', 'position_title', 'phone', 'image', 'is_admin', 'is_active' ]; /** * The attributes that should be hidden for arrays. * * @var array */ protected $hidden = [ 'password', 'remember_token', ]; /** * get all contacts assigned to user * * * @return \Illuminate\Database\Eloquent\Relations\HasMany */ public function contacts() { return $this->hasMany(Contact::class, 'assigned_user_id'); } /** * get all leads assigned to user */ public function leads() { return $this->hasMany(Contact::class, 'assigned_user_id')->where('status', ContactStatus::where('name', config('seed_data.contact_status')[0])->first()->id); } /** * get all opportunities assigned to user */ public function opportunities() { return $this->hasMany(Contact::class, 'assigned_user_id')->where('status', ContactStatus::where('name', config('seed_data.contact_status')[1])->first()->id); } /** * get all customers assigned to user */ public function customers() { return $this->hasMany(Contact::class, 'assigned_user_id')->where('status', ContactStatus::where('name', config('seed_data.contact_status')[2])->first()->id); } /** * get all closed/archives customers assigned to user */ public function archives() { return $this->hasMany(Contact::class, 'assigned_user_id')->where('status', ContactStatus::where('name', config('seed_data.contact_status')[3])->first()->id); } /** * get all documents assigned to user */ public function documents() { return $this->hasMany(Document::class, 'assigned_user_id'); } /** * get all tasks assigned to user */ public function tasks() { return $this->hasMany(Task::class, 'assigned_user_id'); } /** * get all completed tasks assigned to user */ public function completedTasks() { return $this->hasMany(Task::class, 'assigned_user_id')->where('status', TaskStatus::where('name', config('seed_data.task_statuses')[2])->first()->id); } /** * get all pending tasks assigned to user */ public function pendingTasks() { return $this->hasMany(Task::class, 'assigned_user_id')->where('status', TaskStatus::whereIn('name', [config('seed_data.task_statuses')[0], config('seed_data.task_statuses')[1]])->first()->id); } }
As shown the code the relations is self explanatory as most of the relations is a one to many relationships like createdBy(), modifiedBy(), assignedTo() and there are some of them which is a many to many like Contact::documents() and Task::documents().
The most important part is the relations in the user model, as a sales person i must have the capability to view my contacts, leads and opportunities also view my tasks whether completed or pending, for this reasons we add relations like User::contacts(), User::leads(), User::tasks, etc.
Now let’s move on to the next part to prepare the authentication.
Continue to Part 4: Preparing login page>>>