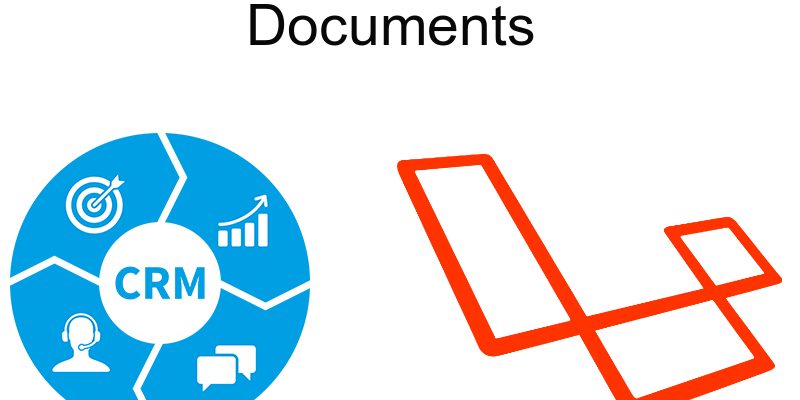
In this part we will implement the documents module that be can selected with contacts or tasks, then we will set the module roles and permissions to allow or disallow access to this module.
Series Topics:
- Part 1: Preparation
- Part 2: Database
- Part 3: Models & Relations
- Part 4: Preparing Login Page
- Part 5: Users Module
- Part 6: Roles & Permissions
- Part 7: Documents Module
- Part 8: Contacts Module
- Part 9: Tasks Module
- Part 10: Mailbox Module
- Part 11: Mailbox Module Complete
- Part 12: Calendar Module
- Part 13: Finishing
The documents in a CRM system represent images, papers, contracts, or licenses. For example when you create a task, that task may have documents or papers attached to it or when you create a lead, that lead also may have some sort of documents. In the documents module we create the documents first then when create a task or lead we just select those documents.
Generate the views:
php artisan crud:view documents --fields="name#string;file#string;status#integer;type#integer;publish_date#string;expiration_date#string;assigned_user_id#integer" --view-path="pages" --route-group=admin --form-helper=html --validations="name#required;file#required"
Generate the controller:
php artisan crud:controller DocumentsController --crud-name=documents --model-name=Document --model-namespace="Models\\" --view-path="pages" --route-group=admin
Next let’s revamp the controller and views to work properly, in the below code i have adjusted the view layout, permissions and others.
Documents Controller
Open app/Http/Controllers/DocumentsController.php and update it as below:
<?php namespace App\Http\Controllers; use App\Helpers\MailerFactory; use App\Models\Document; use App\Models\DocumentType; use App\User; use Illuminate\Http\Request; use Illuminate\Support\Facades\Auth; class DocumentsController extends Controller { protected $mailer; public function __construct(MailerFactory $mailer) { $this->middleware('admin:index-list_documents|create-create_document|show-view_document|edit-edit_document|destroy-delete_document', ['except' => ['store', 'update']]); $this->mailer = $mailer; } /** * Display a listing of the resource. * * @return \Illuminate\View\View */ public function index(Request $request) { $keyword = $request->get('search'); $perPage = 25; if (!empty($keyword)) { $query = Document::where('name', 'like', "%$keyword%"); } else { $query = Document::latest(); } // if not is admin user if(Auth::user()->is_admin == 0) { $query->where('assigned_user_id', Auth::user()->id) ->orWhere('created_by_id', Auth::user()->id); } $documents = $query->paginate($perPage); return view('pages.documents.index', compact('documents')); } /** * Show the form for creating a new resource. * * @return \Illuminate\View\View */ public function create() { $document_types = DocumentType::all(); $users = User::all(); return view('pages.documents.create', compact('document_types', 'users')); } /** * Store a newly created resource in storage. * * @param \Illuminate\Http\Request $request * * @return \Illuminate\Http\RedirectResponse|\Illuminate\Routing\Redirector */ public function store(Request $request) { $this->do_validate($request); $requestData = $request->except(['_token']); checkDirectory("documents"); $requestData['file'] = uploadFile($request, 'file', public_path('uploads/documents')); $requestData['created_by_id'] = Auth::user()->id; $document = Document::create($requestData); if(isset($requestData['assigned_user_id']) && getSetting("enable_email_notification") == 1) { $this->mailer->sendAssignDocumentEmail("Document assigned to you", User::find($requestData['assigned_user_id']), $document); } return redirect('admin/documents')->with('flash_message', 'Document added!'); } /** * Display the specified resource. * * @param int $id * * @return \Illuminate\View\View */ public function show($id) { $document = Document::findOrFail($id); return view('pages.documents.show', compact('document')); } /** * Show the form for editing the specified resource. * * @param int $id * * @return \Illuminate\View\View */ public function edit($id) { $document = Document::findOrFail($id); $document_types = DocumentType::all(); $users = User::all(); return view('pages.documents.edit', compact('document', 'document_types', 'users')); } /** * Update the specified resource in storage. * * @param \Illuminate\Http\Request $request * @param int $id * * @return \Illuminate\Http\RedirectResponse|\Illuminate\Routing\Redirector */ public function update(Request $request, $id) { $this->do_validate($request, 0); $requestData = $request->except(['_token']); if ($request->hasFile('file')) { checkDirectory("documents"); $requestData['file'] = uploadFile($request, 'file', public_path('uploads/documents')); } $requestData['modified_by_id'] = Auth::user()->id; $document = Document::findOrFail($id); $old_assign_user_id = $document->assigned_user_id; $document->update($requestData); if(isset($requestData['assigned_user_id']) && $old_assign_user_id != $requestData['assigned_user_id'] && getSetting("enable_email_notification") == 1) { $this->mailer->sendAssignDocumentEmail("Document assigned to you", User::find($requestData['assigned_user_id']), $document); } return redirect('admin/documents')->with('flash_message', 'Document updated!'); } /** * Remove the specified resource from storage. * * @param int $id * * @return \Illuminate\Http\RedirectResponse|\Illuminate\Routing\Redirector */ public function destroy($id) { Document::destroy($id); return redirect('admin/documents')->with('flash_message', 'Document deleted!'); } public function getAssignDocument($id) { $document = Document::find($id); $users = User::where('id', '!=', $document->assigned_user_id)->get(); return view('pages.documents.assign', compact('users', 'document')); } public function postAssignDocument(Request $request, $id) { $this->validate($request, [ 'assigned_user_id' => 'required' ]); $document = Document::find($id); $document->update(['assigned_user_id' => $request->assigned_user_id]); if(getSetting("enable_email_notification") == 1) { $this->mailer->sendAssignDocumentEmail("Document assigned to you", User::find($request->assigned_user_id), $document); } return redirect('admin/documents')->with('flash_message', 'Document assigned!'); } protected function do_validate($request, $is_create = 1) { $mimes = 'mimes:jpg,jpeg,png,gif,pdf,doc,docx,txt,xls,xlsx,odt,dot,html,htm,rtf,ods,xlt,csv,bmp,odp,pptx,ppsx,ppt,potm'; $this->validate($request, [ 'name' => 'required', 'file' => ($is_create == 0? $mimes:"required|" . $mimes) ]); } }
In the above controller this is a modified version of the controller, first i have added the middleware “admin” in the constructorthat we created in previous parts and i passed the permissions as a string in the format “action-permission” separated by “|”.
Next in the index() method i checked to see if the current user is the admin user or not. If this is the admin user then we retrieve and display all documents otherwise we retrieve only the documents assigned to or created by the current user.
In the store() and update() methods i send an email to the user that this document is assigned to.
Documents Views
resources/views/pages/documents/create.blade.php
@extends('layout.app') @section('title', ' | Create document') @section('content') <section class="content-header"> <h1> Create New document </h1> <ol class="breadcrumb"> <li><a href="{{ url('/admin/') }}"><i class="fa fa-dashboard"></i> Dashboard</a></li> <li><a href="{{ url('/admin/documents') }}">Documents</a></li> <li class="active">Create</li> </ol> </section> <section class="content"> <div class="row"> <div class="col-md-12"> <div class="card"> <div class="card-body"> <a href="{{ url('/admin/documents') }}" title="Back"><button class="btn btn-warning btn-sm"><i class="fa fa-arrow-left" aria-hidden="true"></i> Back</button></a> <br /> <br /> @if ($errors->any()) <ul class="alert alert-danger"> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> @endif <form method="POST" action="{{ url('/admin/documents') }}" accept-charset="UTF-8" enctype="multipart/form-data"> {{ csrf_field() }} @include ('pages.documents.form', ['formMode' => 'create']) </form> </div> </div> </div> </div> </section> @endsection @section('scripts') <script> $(document).ready(function (e) { $("#publish_date, #expiration_date").datepicker(); }); </script> @endsection
resources/views/pages/documents/edit.blade.php
@extends('layout.app') @section('title', ' | Edit document') @section('content') <section class="content-header"> <h1> Edit document #{{ $document->id }} </h1> <ol class="breadcrumb"> <li><a href="{{ url('/admin/') }}"><i class="fa fa-dashboard"></i> Dashboard</a></li> <li><a href="{{ url('/admin/documents') }}">Documents</a></li> <li class="active">Edit</li> </ol> </section> <section class="content"> <div class="row"> <div class="col-md-12"> <div class="card"> <div class="card-body"> <a href="{{ url('/admin/documents') }}" title="Back"><button class="btn btn-warning btn-sm"><i class="fa fa-arrow-left" aria-hidden="true"></i> Back</button></a> <br /> <br /> @if ($errors->any()) <ul class="alert alert-danger"> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> @endif <form method="POST" action="{{ url('/admin/documents/' . $document->id) }}" accept-charset="UTF-8" enctype="multipart/form-data"> {{ method_field('PATCH') }} {{ csrf_field() }} @include ('pages.documents.form', ['formMode' => 'edit']) </form> </div> </div> </div> </div> </section> @endsection @section('scripts') <script> $(document).ready(function (e) { $("#publish_date, #expiration_date").datepicker(); }); </script> @endsection
resources/views/pages/documents/form.blade.php
<div class="row"> <div class="col-md-6"> <div class="form-group {{ $errors->has('name') ? 'has-error' : ''}}"> <label for="name" class="control-label">{{ 'Name' }}</label> <input class="form-control" name="name" type="text" id="name" value="{{ isset($document->name) ? $document->name : ''}}"> {!! $errors->first('name', '<p class="help-block">:message</p>') !!} </div> </div> <div class="col-md-6"> @if(isset($document->file) && !empty($document->file)) <a href="{{ url('uploads/documents/' . $document->file) }}"><i class="fa fa-download"></i> {{$document->file}}</a> @endif <div class="form-group {{ $errors->has('file') ? 'has-error' : ''}}"> <label for="file" class="control-label">{{ 'File' }}</label> <input class="form-control" name="file" type="file" id="file"> {!! $errors->first('file', '<p class="help-block">:message</p>') !!} </div> </div> </div> <div class="row"> <div class="col-md-6"> <div class="form-group {{ $errors->has('status') ? 'has-error' : ''}}"> <label for="status" class="control-label">{{ 'Status' }}</label> <select name="status" id="status" class="form-control"> @foreach(array(1 => "active", 2 => "not active") as $key => $value) <option value="{{ $key }}" {{ isset($document->status) && $document->status == $key?"selected":"" }}>{{ $value }}</option> @endforeach </select> {!! $errors->first('status', '<p class="help-block">:message</p>') !!} </div> </div> <div class="col-md-6"> <div class="form-group {{ $errors->has('type') ? 'has-error' : ''}}"> <label for="type" class="control-label">{{ 'Type' }}</label> <select name="type" id="type" class="form-control"> @foreach($document_types as $document_type) <option value="{{ $document_type->id }}" {{ isset($document->typ) && $document->type == $document_type->id?"selected":"" }}>{{ $document_type->name }}</option> @endforeach </select> {!! $errors->first('type', '<p class="help-block">:message</p>') !!} </div> </div> </div> <div class="row"> <div class="col-md-6"> <div class="form-group {{ $errors->has('publish_date') ? 'has-error' : ''}}"> <label for="publish_date" class="control-label">{{ 'Publish Date' }}</label> <input class="form-control" name="publish_date" type="text" id="publish_date" value="{{ isset($document->publish_date) ? $document->publish_date : ''}}" > {!! $errors->first('publish_date', '<p class="help-block">:message</p>') !!} </div> </div> <div class="col-md-6"> <div class="form-group {{ $errors->has('expiration_date') ? 'has-error' : ''}}"> <label for="expiration_date" class="control-label">{{ 'Expiration Date' }}</label> <input class="form-control" name="expiration_date" type="text" id="expiration_date" value="{{ isset($document->expiration_date) ? $document->expiration_date : ''}}" > {!! $errors->first('expiration_date', '<p class="help-block">:message</p>') !!} </div> </div> </div> @if(\Auth::user()->is_admin == 1) <div class="form-group {{ $errors->has('assigned_user_id') ? 'has-error' : ''}}"> <label for="assigned_user_id" class="control-label">{{ 'Assigned User' }}</label> <select name="assigned_user_id" id="assigned_user_id" class="form-control"> @foreach($users as $user) <option value="{{ $user->id }}" {{ isset($document->assigned_user_id) && $document->assigned_user_id == $user->id?"selected":"" }}>{{ $user->name }}</option> @endforeach </select> {!! $errors->first('assigned_user_id', '<p class="help-block">:message</p>') !!} </div> @endif <div class="form-group"> <input class="btn btn-primary" type="submit" value="{{ $formMode === 'edit' ? 'Update' : 'Create' }}"> </div>
resources/views/pages/documents/index.blade.php
@extends('layout.app') @section('title', ' | List Documents') @section('content') <section class="content-header"> <h1> Documents </h1> <ol class="breadcrumb"> <li><a href="{{ url('/admin') }}"><i class="fa fa-dashboard"></i> Dashboard</a></li> <li class="active">Documents</li> </ol> </section> <section class="content"> <div class="row"> <div class="col-md-12"> <div class="card"> <div class="card-body"> @include('includes.flash_message') @if(user_can("create_document")) <a href="{{ url('/admin/documents/create') }}" class="btn btn-success btn-sm pull-right" title="Add New document"> <i class="fa fa-plus" aria-hidden="true"></i> Add New </a> @endif <form method="GET" action="{{ url('/admin/documents') }}" accept-charset="UTF-8" class="form-inline my-2 my-lg-0" role="search"> <div class="input-group"> <input type="text" class="form-control" name="search" placeholder="Search..." value="{{ request('search') }}"> <span class="input-group-btn"> <button class="btn btn-secondary" type="submit"> <i class="fa fa-search"></i> </button> </span> </div> </form> <br/> <br/> <div class="table-responsive"> <table class="table"> <thead> <tr> @if(\Auth::user()->is_admin == 1) <th>#</th> @endif <th>Name</th> <th>File</th> <th>Status</th> <th>Created at</th> @if(\Auth::user()->is_admin == 1) <th>Created by</th> @endif <th>Assigned to</th> <th>Actions</th> </tr> </thead> <tbody> @foreach($documents as $item) <tr> @if(\Auth::user()->is_admin == 1) <td>{{ $item->id }}</td> @endif <td>{{ $item->name }}</td> <td>@if(!empty($item->file)) <a href="{{ url('uploads/documents/' . $item->file) }}"> <i class="fa fa-download"></i> {{$item->file}}</a> @endif</td> <td>{!! $item->status == 1?"<i class='label label-success'>Active</i>":"<i class='label label-danger'>Not active</i>" !!}</td> <td>{{ $item->created_at }}</td> @if(\Auth::user()->is_admin == 1) <td>{{ $item->createdBy->name }}</td> @endif <td>{{ $item->assignedTo != null ? $item->assignedTo->name : "not set" }}</td> <td> @if(user_can('view_document')) <a href="{{ url('/admin/documents/' . $item->id) }}" title="View document"><button class="btn btn-info btn-sm"><i class="fa fa-eye" aria-hidden="true"></i> View</button></a> @endif @if(user_can('edit_document')) <a href="{{ url('/admin/documents/' . $item->id . '/edit') }}" title="Edit document"><button class="btn btn-primary btn-sm"><i class="fa fa-pencil-square-o" aria-hidden="true"></i> Edit</button></a> @endif @if(user_can('assign_document')) <a href="{{ url('/admin/documents/' . $item->id . '/assign') }}" title="Assign document"><button class="btn btn-primary btn-sm"><i class="fa fa-envelope-o" aria-hidden="true"></i> Assign</button></a> @endif @if(user_can('delete_document')) <form method="POST" action="{{ url('/admin/documents' . '/' . $item->id) }}" accept-charset="UTF-8" style="display:inline"> {{ method_field('DELETE') }} {{ csrf_field() }} <button type="submit" class="btn btn-danger btn-sm" title="Delete document" onclick="return confirm('Confirm delete?')"><i class="fa fa-trash-o" aria-hidden="true"></i> Delete</button> </form> @endif </td> </tr> @endforeach </tbody> </table> <div class="pagination-wrapper"> {!! $documents->appends(['search' => Request::get('search')])->render() !!} </div> </div> </div> </div> </div> </div> </section> @endsection
resources/views/pages/documents/show.blade.php
@extends('layout.app') @section('title', ' | Show document') @section('content') <section class="content-header"> <h1> document #{{ $document->id }} </h1> <ol class="breadcrumb"> <li><a href="{{ url('/admin/') }}"><i class="fa fa-dashboard"></i> Dashboard</a></li> <li><a href="{{ url('/admin/documents') }}">Documents</a></li> <li class="active">Show</li> </ol> </section> <section class="content"> <div class="row"> <div class="col-md-12"> <div class="card"> <div class="card-body"> <a href="{{ url('/admin/documents') }}" title="Back"><button class="btn btn-warning btn-sm"><i class="fa fa-arrow-left" aria-hidden="true"></i> Back</button></a> @if(user_can('edit_document')) <a href="{{ url('/admin/documents/' . $document->id . '/edit') }}" title="Edit document"><button class="btn btn-primary btn-sm"><i class="fa fa-pencil-square-o" aria-hidden="true"></i> Edit</button></a> @endif @if(user_can('delete_document')) <form method="POST" action="{{ url('admin/documents' . '/' . $document->id) }}" accept-charset="UTF-8" style="display:inline"> {{ method_field('DELETE') }} {{ csrf_field() }} <button type="submit" class="btn btn-danger btn-sm" title="Delete document" onclick="return confirm('Confirm delete?')"><i class="fa fa-trash-o" aria-hidden="true"></i> Delete</button> </form> @endif <br/> <br/> <div class="table-responsive"> <table class="table"> <tbody> @if(\Auth::user()->is_admin == 1) <tr> <th>ID</th><td>{{ $document->id }}</td> </tr> @endif <tr><th> Name </th><td> {{ $document->name }} </td></tr> <tr><th> File </th><td> @if(!empty($document->file)) <a href="{{ url('uploads/documents/' . $document->file) }}"> <i class="fa fa-download"></i> {{$document->file}}</a> @endif </td></tr> <tr><th> Status </th><td> {!! $document->status == 1?"<i class='label label-success'>Active</i>":"<i class='label label-danger'>Not active</i>" !!} </td></tr> <tr><th> Type </th><td>{{ $document->getType->name }}</td></tr> <tr><th> Publish date </th><td>{{ $document->publish_date }}</td></tr> <tr><th> Expiration date </th><td>{{ $document->expiration_date }}</td></tr> @if(\Auth::user()->is_admin == 1) <tr><th> Created by </th><td>{{ $document->createdBy->name }}</td></tr> <tr><th> Modified by </th><td>{{ isset($document->modifiedBy->name)?$document->modifiedBy->name:"" }}</td></tr> @endif <tr><th> Assigned to </th><td>{{ $document->assignedTo != null ?$document->assignedTo->name : "not set" }}</td></tr> <tr><th> Created at </th><td>{{ $document->created_at }}</td></tr> <tr><th> Modified at </th><td>{{ $document->updated_at }}</td></tr> </tbody> </table> </div> </div> </div> </div> </div> </section> @endsection
resources/views/pages/documents/assign.blade.php
@extends('layout.app') @section('title', ' | Assign contact') @section('content') <section class="content-header"> <h1> Assign Document </h1> <ol class="breadcrumb"> <li><a href="{{ url('/admin/') }}"><i class="fa fa-dashboard"></i> Dashboard</a></li> <li><a href="{{ url('/admin/documents') }}">Documents</a></li> <li class="active">Assign</li> </ol> </section> <section class="content"> <div class="row"> <div class="col-md-12"> <div class="card"> <div class="card-body"> <a href="{{ url('/admin/documents') }}" title="Back"><button class="btn btn-warning btn-sm"><i class="fa fa-arrow-left" aria-hidden="true"></i> Back</button></a> <br /> <br /> @if ($errors->any()) <ul class="alert alert-danger"> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> @endif <form method="POST" action="{{ url('/admin/documents/' . $document->id . '/assign') }}" accept-charset="UTF-8" enctype="multipart/form-data"> {{ csrf_field() }} {{ method_field("put") }} <div class="form-group {{ $errors->has('assigned_user_id') ? 'has-error' : ''}}"> <label for="assigned_user_id" class="control-label">{{ 'Assigned User' }}</label> <select name="assigned_user_id" id="assigned_user_id" class="form-control"> @foreach($users as $user) <option value="{{ $user->id }}">{{ $user->name }}</option> @endforeach </select> {!! $errors->first('assigned_user_id', '<p class="help-block">:message</p>') !!} </div> <div class="form-group"> <input class="btn btn-primary" type="submit" value="Assign"> </div> </form> </div> </div> </div> </div> </section> @endsection
Update the routes
routes/web.php
<?php /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::group(['prefix' => 'admin', 'middleware' => 'auth'], function () { Route::get('/', function () { return view('pages.home.index'); }); Route::resource('/users', 'UsersController'); Route::get('/my-profile', 'UsersController@getProfile'); Route::get('/my-profile/edit', 'UsersController@getEditProfile'); Route::patch('/my-profile/edit', 'UsersController@postEditProfile'); Route::resource('/permissions', 'PermissionsController'); Route::resource('/roles', 'RolesController'); Route::get('/users/role/{id}', 'UsersController@getRole'); Route::put('/users/role/{id}', 'UsersController@updateRole'); Route::resource('/documents', 'DocumentsController'); Route::get('/documents/{id}/assign', 'DocumentsController@getAssignDocument'); Route::put('/documents/{id}/assign', 'DocumentsController@postAssignDocument'); Route::get('/forbidden', function () { return view('pages.forbidden.forbidden_area'); }); }); Route::get('/', function () { return redirect()->to('/admin'); }); Auth::routes();
Update the sidebar
resources/views/layout/sidebar.blade.php
<!-- Left side column. contains the logo and sidebar --> <aside class="main-sidebar"> <!-- sidebar: style can be found in sidebar.less --> <section class="sidebar"> <!-- Sidebar user panel --> <div class="user-panel"> <div class="pull-left image"> @if(\Auth::user()->image != null) <img src="{{ url('uploads/users/' . \Auth::user()->image) }}" class="img-circle" alt="User Image"> @else <img src="{{ url('theme/dist/img/image_placeholder.png') }}" class="img-circle" alt="User Image"> @endif </div> <div class="pull-left info"> <p>{{ \Auth::user()->name }}</p> </div> </div> <!-- sidebar menu: : style can be found in sidebar.less --> <ul class="sidebar-menu" data-widget="tree"> <li class="header">MAIN NAVIGATION</li> <li class="{{ Request::segment(2) == ""?"active":"" }}"> <a href="{{ url('/admin') }}"> <i class="fa fa-dashboard"></i> <span>Dashboard</span> </a> </li> @if(user_can('list_documents')) <li class="{{ Request::segment(2) == "documents"?"active":"" }}"> <a href="{{ url('/admin/documents') }}"> <i class="fa fa-file-word-o"></i> <span>Documents</span> </a> </li> @endif @if(\Auth::user()->is_admin == 1) <li class="{{ in_array(Request::segment(2), ['users', 'permissions', 'roles'])?"active":"" }} treeview"> <a href="#"> <i class="fa fa-users"></i> <span>User Managment</span> <span class="pull-right-container"> <i class="fa fa-angle-left pull-right"></i> </span> </a> <ul class="treeview-menu"> <li class="{{ Request::segment(2) == "users"?"active":"" }}"> <a href="{{ url('/admin/users') }}"><i class="fa fa-user-o"></i> Users</a> </li> <li class="{{ Request::segment(2) == "permissions"?"active":"" }}"> <a href="{{ url('/admin/permissions') }}"><i class="fa fa-ban"></i> Permissions</a> </li> <li class="{{ Request::segment(2) == "roles"?"active":"" }}"> <a href="{{ url('/admin/roles') }}"><i class="fa fa-list"></i> Roles</a> </li> </ul> </li> @endif </ul> </section> <!-- /.sidebar --> </aside>
In the above code i have added a new route and added new item in the sidebar to enable the navigation to the documents module, also i checked for the permission to allow or disallow the user to view this module.
Also i have modified the views a little bit by adjusting some fields such as the file upload field and select dropdown for the assigned_user_id field.
Modify app/Helpers/MailerFactory.php add this method at the end of the class:
public function sendAssignDocumentEmail($subject, $user, $document) { try { $this->mailer->send("emails.assign_document", ['user' => $user, 'document' => $document, 'subject' => $subject], function($message) use ($subject, $user) { $message->from($this->fromAddress, $this->fromName) ->to($user->email)->subject($subject); }); } catch (\Exception $ex) { die("Mailer Factory error: " . $ex->getMessage()); } }
resources/views/emails/assign_document.blade.php
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>{{ $subject }}</title> </head> <body> <p> Hello {{ $user->name }}, </p> <p> You have been assigned a document, go and check them here <a href="{{ url('admin/documents/' . $document->id) }}"> {{ url('admin/documents/' . $document->id) }} </a> </p> </body> </html>
Now navigate to http://localhost/crm/admin/documents and login check your permissions, add a new user with different permissions and try to add, edit and delete documents.
Updating User Profile
Let’s update the user profile page to show the documents assigned to the currently logged in user
resources/views/pages/users/profile/view.blade.php
@extends('layout.app') @section('title', ' | My Profile') @section('content') <section class="content-header"> <h1> My Profile </h1> </section> <section class="content"> <div class="row"> <div class="col-md-12"> <div class="card"> <div class="card-body"> @include('includes.flash_message') @if(user_can('edit_profile')) <a href="{{ url('/admin/my-profile/edit') }}" title="Edit profile"><button class="btn btn-primary btn-sm"><i class="fa fa-pencil-square-o" aria-hidden="true"></i> Edit</button></a> @endif <br/> <br/> <div class="table-responsive"> <table class="table"> <tbody> @if(!empty($user->image)) <tr> <td> <img src="{{ url('uploads/users/' . $user->image) }}" class="pull-right" width="200" height="200" /> </td> </tr> @endif <tr><th> Name </th><td> {{ $user->name }} </td> </tr><tr><th> Email </th><td> {{ $user->email }} </td></tr> <tr><th> Position Title </th><td> {{ $user->position_title }} </td></tr> <tr><th> Phone </th><td> {{ $user->phone }} </td></tr> </tbody> </table> <hr/> @if(user_can('list_documents')) <h3>Documents assigned</h3> @if($user->documents->count() > 0) <table class="table"> <tr> <th>Name</th> <th>View</th> </tr> <tbody> @foreach($user->documents as $document) <tr> <td>{{ $document->name }}</td> <td> @if(user_can("view_document")) <a href="{{ url('/admin/documents/' . $document->id) }}"><i class="fa fa-camera"></i></a> @endif </td> </tr> @endforeach </tbody> </table> @else <p>No documents assigned</p> @endif @endif </div> </div> </div> </div> </div> </section> @endsection
Continue to Part 8: contacts module>>>