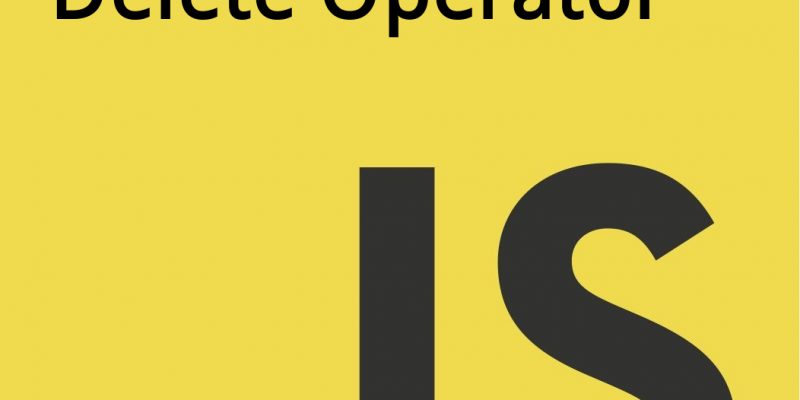
The JavaScript delete
operator removes a property from an object; if no more references to the same property are held, it is eventually released automatically.
When using the delete
operator on arrays take caution as delete will delete the specified item but the array length not get affected.
Let’s take an example
var Employee = { firstname: "Jon", lastname: "Doe" } console.log(Employee.firstname); // expected output: "Jon" delete Employee.firstname; console.log(Employee.firstname); // expected output: undefined
In the code above we defined an object with two items and then displayed the “firstname” property in the console. Next i delete the “firstname” property as shown above after that when displaying the property again it shows “undefined”.
delete
operator will return true
for all cases except when the property is an own non-configurable property, in which case, false
is returned in non-strict mode.
delete operator Exceptions
The delete
operator removes a given property from an object. On successful deletion, it will return true
, else false
will be returned. However, it is important to consider the following scenarios:
- If the property which you are trying to delete does not exist,
delete
will not have any effect and will returntrue
- If a property with the same name exists on the object’s prototype chain, then, after deletion, the object will use the property from the prototype chain (in other words,
delete
only has an effect on own properties). - Any property declared with
var
cannot be deleted from the global scope or from a function’s scope. - Any property declared with
let
orconst
cannot be deleted from the scope within which they were defined. - Non-configurable properties cannot be removed. This includes properties of built-in objects like
Math
,Array
,Object
and properties that are created as non-configurable with methods likeObject.defineProperty()
.
The following snippet gives a simple example:
var Employee = { age: 28, name: 'abc', designation: 'developer' } console.log(delete Employee.name); // returns true console.log(delete Employee.age); // returns true // When trying to delete a property that does // not exist, true is returned console.log(delete Employee.salary); // returns true
Â
Non-configurable properties
When a property is marked as non-configurable, delete
won’t have any effect, and will return false
. In strict mode this will raise a SyntaxError
.
var Employee = {}; Object.defineProperty(Employee, 'name', {configurable: false}); console.log(delete Employee.name); // returns false
var
, let
and const
create non-configurable properties that cannot be deleted with the delete
operator:
var nameOther = 'XYZ'; delete nameOther; // return false
// creates the property adminName on the global scope adminName = 'xyz'; // creates the property empCount on the global scope // Since we are using var, this is marked as non-configurable. The same is true of let and const. var empCount = 43; EmployeeDetails = { name: 'xyz', age: 5, designation: 'Developer' }; // adminName is a property of the global scope. // It can be deleted since it is created without var. // Therefore, it is configurable. delete adminName; // returns true // On the contrary, empCount is not configurable, // since var was used. delete empCount; // returns false // delete can be used to remove properties from objects delete EmployeeDetails.name; // returns true // Even when the property does not exists, it returns "true" delete EmployeeDetails.salary; // returns true // delete does not affect built-in static properties delete Math.PI; // returns false // EmployeeDetails is a property of the global scope. // Since it defined without "var", it is marked configurable delete EmployeeDetails; // returns true function f() { var z = 44; // delete doesn't affect local variable names delete z; // returns false }