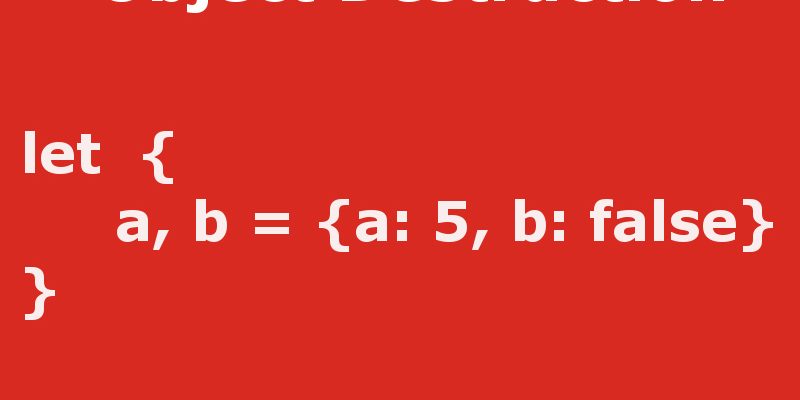
In this post i will show you a very powerful feature in Ecma script 2015 which is the Object Destruction feature and how to extract variables from array and object keys.
Object Destruction means tear a part an object or array into it’s original items which is the inverse of Construction where you build up some sort of collection using keys and values, so the destruction is the process of extracting an object or array items into separate variables where the name of the variable is something you define and the value is the corresponding on that array or object. This feature already exist in a lot of language such as PHP and Python.
Terminology
// for arrays [variable1, variable2, variable3] = array() for objects {variable1, variable2, variable3} = {}
As you see in the above terminology we assign the right hand side which can be an array or object to the left hand side which also can be and array or variables or object of variables.
Destructing Arrays
Let’s imagine we have this array
var months = ['January', 'February', 'March', 'April'];
Now if we want to extract this 4 element array to 4 variables like this:
var [first_day, second_day, third_day, fourth_day] = months; console.log(first_day, second_day, third_day, fourth_day); // output January February March April
As you see from the output the values assigned in the order which they defined in the array.
You can also declare the variables first:
var first_day, second_day, third_day, fourth_day; [first_day, second_day, third_day, fourth_day] = months; console.log(first_day, second_day, third_day, fourth_day);
Â
Using default values
In cases where the array contains just little values than the defined variables on the left side you can assign those variables default values which will be replaced by undefined for example:
[first_day='jan', second_day='feb', third_day='march', fourth_day='april'] = ['January']; console.log(first_day); console.log(second_day); console.log(third_day); console.log(fourth_day); // output January feb // the default value march // the default value april // the default value
Ignoring specific values
If you need to ignore specific values in the array you can replace it by empty variable like this:
var [first_day, , third_day, ] = months; console.log(first_day); console.log(third_day); // output January March
What if you want to assign the rest of the array to a variable, you can accomplish this using the rest expression like shown below:
[first_day, ...other_days ] = months; console.log(first_day); console.log(other_days); // output January Array [ "February", "March", "April" ]
But don’t use a trailing comma after the rest expression like this:
[first_day, ...other_days, ] = months; // Error: rest element may not have a trailing comma
Destructing Objects
Destruction in objects works in the same fashion as arrays, you specify an object of the right side to be assigned to any object of variables on the left side
let months = {first_day: 'January', second_day: 'February', third_day: 'March'};
let {first_day, second_day, third_day} = months; console.log(first_day); console.log(second_day); console.log(third_day);
As you see above we specified the variables in object expression instead of array expression, you can also declare the variables first:
let first_day, second_day, third_day; ({first_day, second_day, third_day} = months);
Note here the parentheses is needed in case of object destruction if you omit them you will get a syntax error.
Using new variable names as object values
You can use new variable names as the object values instead of the object keys when extracting like this:
({first_day: first, second_day: second, third_day: third} = months); console.log(first); console.log(second); console.log(third);
Object destructing default values
Default values works in the same manner as arrays, you pass the object keys in the left side a default value like this example:
({first_day, second_day = 'feb', third_day = 'march'} = {first_day: 'January'}); console.log(first_day); console.log(second_day); console.log(third_day); // output January feb march
As you see above we passed default values to the second and third items in the object so that those values be used in case no actual value exist.
You can use also new naming with the default values as in this example:
const {width: W = 10, height: H = 5} = {width: 3}; console.log(W); // 3 console.log(H); // 5
Â
Function parameter default values
Object destruction can very helpful when setting function parameters default values easily, remember in the old days to set default values for the function you may have done something like this:
function do_something(options) { options = options === undefined ? {} : options; var param1 = options.param1 === undefined ? '' : options.param1; var param2 = options.param2 === undefined ? '' : options.param2; }
But using the object destruction syntax this example can be simplified as shown here:
function do_something({param1 = 5, param2 = true} = {}) { console.log(param1, param2); } do_something(); do_something({ param1: 20 }); do_something({ param2: false }); do_something({ param1: 45, param2: false });
Note how the object destruction syntax makes it easy to use the default values without going through the nasty checks as in the first function, now you can call the function without parameters , with only one parameter or with the two parameters.
Nested Objects
The power of object destruction comes in handy when you need to capture nested object expressions without having to navigate all the object deep to get a certain value, consider this example from google maps autocomplete api response:
let autocompleteResponse = { address_components: [ { long_name: "58", short_name: "58", types: [] }, { long_name: "Helsinki", short_name: "HKI", types: [] }, { long_name: "Finland", short_name: "FI", types: [] }, { long_name: "00500", short_name: "00500", types: [] } ], adr_address: "Hämeentie 58 Helsinki Finland", formatted_address: "Hameentie 58, 00500 Helsinki, Finland", geometry: { location: { lat: "34.68484", lng: "67.43333" } }, icon: "https://maps.gstatic.com/mapfiles/place_api/icons/geocode-71.png", id: "1e60bcce0153a978681bd4caf8504c3438f91555", name: "Hämeentie 58", place_id: "ChIJnRTUNHkJkkYRttAbgCFYfPw", plus_code: { compound_code: "5XQ7+88 Helsinki, Finland", global_code: "9GG65XQ7+88" }, zip_code: "00500" };
Now if for example you need to access some nested keys like lat or lng you may have to do something like this:
let lat = autocompleteResponse.geometry.location.lat; let lng = autocompleteResponse.geometry.location.lng;
Using object expressions this can simplified like shown below:
let { lat, lng } = autocompleteResponse.geometry.location; console.log(lat); console.log(lng);
As you see we extracted the nested properties with an easy way using the closest parent autocompleteResponse.geometry.location
Using the same methodology we can access other values like this:
let { compound_code, global_code } = autocompleteResponse.plus_code; console.log(compound_code); console.log(global_code); // Iterating and object destruction for(var {long_name, short_name} of autocompleteResponse.address_components) { console.log("long name: " + long_name + " - short name: " + short_name); }