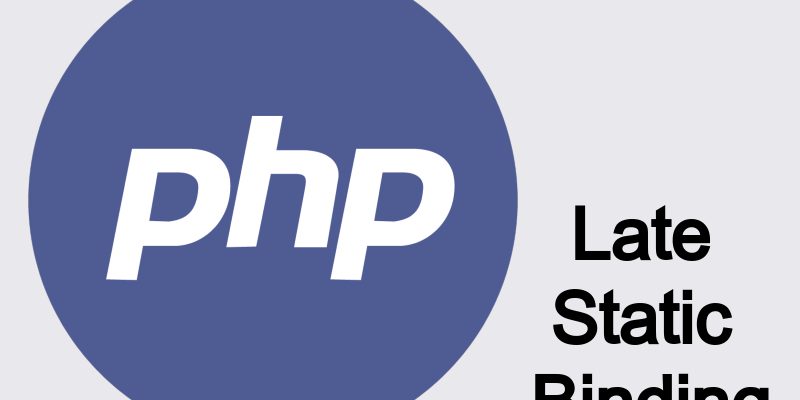
The late static binding feature introduced in PHP 5.3 and later which allows to reference child class methods inside the parent class using static keyword.
The late static binding feature is a feature in PHP object oriented programming, and in this feature we speak in context of class inheritance as this feature introduced to solve a problem when dealing with inheritance relationship between parent and child classes.
To understand the late static binding, let’s consider this example:
class ImageDriver { static function classname(){ return __CLASS__; } public static function displayName() { echo "The image driver is " . self::classname(); } } class ImagickDriver extends ImageDriver { static function classname(){ return __CLASS__; } }
In this simple example we have two classes that represent an image driver used in a web application. The base class ImageDriver contains a static method displayName() which displays the current driver name (class name) using the “self” keyword which invokes the classname() utility method.
Then i added another class ImagickDriver which inherit from ImageDriver and overrides the classname() method.
Now if we create an instance ImageDriver and invoke displayName() method:
echo ImageDriver::displayName() . "\n";
output
The image driver is ImageDriver
The output is expected, but if we try to create an instance of the child class:
echo ImagickDriver::displayName() . "\n";
output
The image driver is ImageDriver
You will see the same output which is strange, it’s supposed to output the current class name, and this problem that late static binding solve.
Using static keyword
Let’s improve the example to use the static keyword:
public static function displayName() { echo "The image driver is " . static::classname(); }
As you see i updated the displayName() method so instead of calling the classname() method using “self” we replaced it with the “static” keyword.
After running the example again it will display the correct class name:
The image driver is ImageDriver The image driver is ImagickDriver
From this according to php.net the “late static binding” work by storing the class named in the last “non-forwarding call”. In case of static methods, this is the class explicitly named (this is the one if the left of :: operator). In the case of non static method calls it’s the class of the object.
The term “Late Binding” comes from the fact that static:: will not be resolved using the class where the method is declared, but it will be computed at the runtime from the calling context as in the above example in ImagickDriver::displayname()
The term “Static Binding” is used mostly with static method calls but this is not limited as it can be used to non static method calls.
Let’s refactor the above example to be non static:
<?php class ImageDriver { function classname(){ return __CLASS__; } public function displayName() { echo "The image driver is " . static::classname(); } } class ImagickDriver extends ImageDriver { function classname(){ return __CLASS__; } } $imageDriver = new ImageDriver(); echo $imageDriver->displayName() . "\n"; $imagick = new ImagickDriver(); echo $imagick->displayName() . "\n";
output
The image driver is ImageDriver The image driver is ImagickDriver
Common Uses Of Late Static Binding
- Calling the right child class method.
- When dealing with shared functionality, so instead of repeating the code again and again in child classes we use the parent class method as the entry point to call the dedicated child class method.