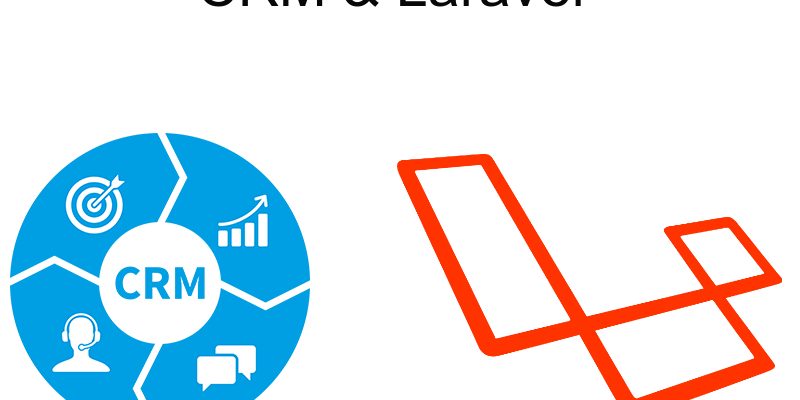
Today we will open the final chapter of “Implementing CRM system with laravel” and in this part we will add the final pieces to our system.
Series Topics:
- Part 1: Preparation
- Part 2: Database
- Part 3: Models & Relations
- Part 4: Preparing Login Page
- Part 5: Users Module
- Part 6: Roles & Permissions
- Part 7: Documents Module
- Part 8: Contacts Module
- Part 9: Tasks Module
- Part 10: Mailbox Module
- Part 11: Mailbox Module Complete
- Part 12: Calendar Module
- Part 13: Finishing
Updating Dashboard Homepage
Let’s update the dashboard homepage so we will show some quick links and some statistics according to whether the user is super admin or not. To do that we will some functions to Generals.php file
Add those functions at the end of app/Helpers/General.php
/** * getContacts * * * @param null $status * @return \Illuminate\Database\Eloquent\Collection|static[] */ function getContacts($status = null) { if(!$status) return \App\Models\Contact::all(); return \App\Models\Contact::join('contact_status', 'contact_status.id', '=', 'contact.status') ->where('contact_status.name', $status) ->get(); } /** * get Users * * * @return mixed */ function getUsers() { return \App\User::where('is_admin', 0)->where('is_active', 1)->get(); }
Next open resources/views/pages/home/index.blade.php and update it with this code
@extends('layout.app') @section('title', ' | Dashboard') @section('content') <!-- Content Header (Page header) --> <section class="content-header"> <h1> Dashboard <small>Control panel</small> </h1> <ol class="breadcrumb"> <li><a href="#"><i class="fa fa-dashboard"></i> Home</a></li> <li class="active">Dashboard</li> </ol> </section> <section class="content"> @if(\Auth::user()->is_admin == 1) <!-- Small boxes (Stat box) --> <div class="row"> @if(count(getContacts()) > 0) <div class="col-lg-3 col-xs-6"> <!-- small box --> <div class="small-box bg-aqua"> <div class="inner"> <h3>{{ count(getContacts()) }}</h3> <p>Contacts</p> </div> <div class="icon"> <i class="ion ion-bag"></i> </div> <a href="{{ url('admin/contacts') }}" class="small-box-footer">More info <i class="fa fa-arrow-circle-right"></i></a> </div> </div> <!-- ./col --> @endif @if(count(getContacts('Lead')) > 0) <div class="col-lg-3 col-xs-6"> <!-- small box --> <div class="small-box bg-green"> <div class="inner"> <h3>{{count(getContacts('Lead'))}}</h3> <p>Leads</p> </div> <div class="icon"> <i class="ion ion-stats-bars"></i> </div> <a href="{{ url('admin/contacts?status_name=Lead') }}" class="small-box-footer">More info <i class="fa fa-arrow-circle-right"></i></a> </div> </div> <!-- ./col --> @endif @if(count(getContacts('Opportunity')) > 0) <div class="col-lg-3 col-xs-6"> <!-- small box --> <div class="small-box bg-yellow"> <div class="inner"> <h3>{{count(getContacts('Opportunity'))}}</h3> <p>Opportunities</p> </div> <div class="icon"> <i class="ion ion-person-add"></i> </div> <a href="{{ url('admin/contacts?status_name=Opportunity') }}" class="small-box-footer">More info <i class="fa fa-arrow-circle-right"></i></a> </div> </div> <!-- ./col --> @endif @if(count(getContacts('Customer')) > 0) <div class="col-lg-3 col-xs-6"> <!-- small box --> <div class="small-box bg-red"> <div class="inner"> <h3>{{count(getContacts('Customer'))}}</h3> <p>Potential Customers</p> </div> <div class="icon"> <i class="ion ion-pie-graph"></i> </div> <a href="{{ url('admin/contacts?status_name=Customer') }}" class="small-box-footer">More info <i class="fa fa-arrow-circle-right"></i></a> </div> </div> <!-- ./col --> @endif </div> <div class="row"> <section class="col-lg-12 connectedSortable ui-sortable"> <!-- TO DO List --> <div class="box box-primary"> <div class="box-header"> <i class="ion ion-clipboard"></i> <h3 class="box-title">My Team</h3> </div> <!-- /.box-header --> <div class="box-body"> <table class="table table-responsive"> <thead> <tr> <th>Name</th> <th>Email</th> <th>Position</th> <th>Contacts</th> <th>Tasks</th> <th>Options</th> </tr> </thead> <tbody> @foreach(getUsers() as $user) <tr> <td>{{ $user->name }}</td> <td>{{ $user->email }}</td> <td>{{ $user->position_title }}</td> <td><a href="{{ url('admin/contacts?assigned_user_id=' . $user->id) }}">{{ $user->contacts->count() }}</a></td> <td><a href="{{ url('admin/tasks?assigned_user_id=' . $user->id) }}">{{ $user->tasks->count() }}</a></td> <td><a href="{{ url('admin/users/' . $user->id) }}" data-toggle="tooltip" title="view details"><i class="fa fa-camera"></i></a></td> </tr> @endforeach </tbody> </table> </div> </div> <!-- /.box --> </section> </div> @else <div class="row"> @if(user_can("list_contacts")) @if(\Auth::user()->contacts->count() > 0) <div class="col-lg-3 col-xs-6"> <!-- small box --> <div class="small-box bg-aqua"> <div class="inner"> <h3>{{ \Auth::user()->contacts->count() }}</h3> <p>My Contacts</p> </div> <div class="icon"> <i class="ion ion-bag"></i> </div> <a href="{{ url('admin/contacts') }}" class="small-box-footer">More info <i class="fa fa-arrow-circle-right"></i></a> </div> </div> <!-- ./col --> @endif @if(\Auth::user()->leads->count() > 0) <div class="col-lg-3 col-xs-6"> <!-- small box --> <div class="small-box bg-green"> <div class="inner"> <h3>{{ \Auth::user()->leads->count() }}</h3> <p>My Leads</p> </div> <div class="icon"> <i class="ion ion-stats-bars"></i> </div> <a href="{{ url('admin/contacts?status_name=Lead') }}" class="small-box-footer">More info <i class="fa fa-arrow-circle-right"></i></a> </div> </div> <!-- ./col --> @endif @if(\Auth::user()->opportunities->count() > 0) <div class="col-lg-3 col-xs-6"> <!-- small box --> <div class="small-box bg-yellow"> <div class="inner"> <h3>{{ \Auth::user()->opportunities->count() }}</h3> <p>My Opportunities</p> </div> <div class="icon"> <i class="ion ion-person-add"></i> </div> <a href="{{ url('admin/contacts?status_name=Opportunity') }}" class="small-box-footer">More info <i class="fa fa-arrow-circle-right"></i></a> </div> </div> <!-- ./col --> @endif @if(\Auth::user()->customers->count() > 0) <div class="col-lg-3 col-xs-6"> <!-- small box --> <div class="small-box bg-red"> <div class="inner"> <h3>{{ \Auth::user()->customers->count() }}</h3> <p>My Potential Customers</p> </div> <div class="icon"> <i class="ion ion-pie-graph"></i> </div> <a href="{{ url('admin/contacts?status_name=Customer') }}" class="small-box-footer">More info <i class="fa fa-arrow-circle-right"></i></a> </div> </div> <!-- ./col --> @endif @endif </div> @if(user_can("list_tasks")) <div class="row"> <section class="col-lg-12 connectedSortable ui-sortable"> <div class="box box-primary"> <div class="box-header"> <i class="ion ion-clipboard"></i> <h3 class="box-title">To do list</h3> </div> <div class="box-body"> <ul class="todo-list"> @foreach(\Auth::user()->tasks as $task) <li> <span class="handle ui-sortable-handle"> <i class="fa fa-ellipsis-v"></i> <i class="fa fa-ellipsis-v"></i> </span> <span class="text">{{ $task->name }}</span> @if($task->getStatus->name == 'Not Started') <small class="label label-warning">not started</small> @elseif($task->getStatus->name == 'Started') <small class="label label-info">started</small> @elseif($task->getStatus->name == 'Completed') <small class="label label-success">completed</small> @else <small class="label label-danger">cancelled</small> @endif </li> @endforeach </ul> </div> </div> </section> </div> @endif @endif </section> @stop
In this code i checked if the user is super admin. If it’s super admin i show a list of boxes that display the contacts count, leads count, opportunities and so on. Then i show a grid that displays the crm sales person.
If the user is not a super admin in other means it’s a sales person we display the same boxes but in this case we display the contacts assigned to this user. Also we display a to do list of tasks at the end.
At this point we finished the crm system and below is the repository url on bitbucket.
https://bitbucket.org/webmobtuts/crm/src/master/
Conclusion
In this series you have learned how to create a basic CRM system with laravel, we covered also some important topics related to laravel also such as authorization and how to deal with permissions and roles, saving them into database and assign roles to users. Also we covered some basic CRM modules such as contacts and tasks and mailbox. There are a lot to do with a CRM system, try to add more features to this system for example try to make a dynamic mailing template and storing them into database and so on.
I downloaded the source to see hot it looks like and it gave me a “The cookie name cannot be empty”
What is the issue here? I also seeded the database so the admin is in the user table
May be you need to set COOKIE_NAME to specific name in the project .env file
It doest work, need help
May be you haven’t done these steps:
– Remove composer.lock and then run composer install
– run php artisan key:generate
– create public/uploads directory and make it writable
– make writable /storage directory
– set SESSION_COOKIE to some name in project .env
@Wael, I downloaded the source code and it perfectly worked for me. Thanks a lot it save my time.
Appreciate you work, Keep posting such content.
Will you please post tutorial for OOPS concept with working example? It will be your great help 🙂
Regards
Anuj
Thanks, just keep following us for future tutorials
In ContactsController.php, the query returns wront ID.
Change:
return $contacts->get(‘contact.*’);
instead of return $contacts->get();
May be you are using different laravel version
Hi , I thank for this tutorial really useful.
I download the repository but am blocked at the level of the login.
each time I enter the user information’s its says These credentials do not match our records.
Can you help me resolve my problem?
Debug and check the login code in part 4
http://webmobtuts.com/backend-development/implementing-crm-system-with-laravel-part-4-preparing-login-page/
Also check the user is active and is_active flag is 1 in db