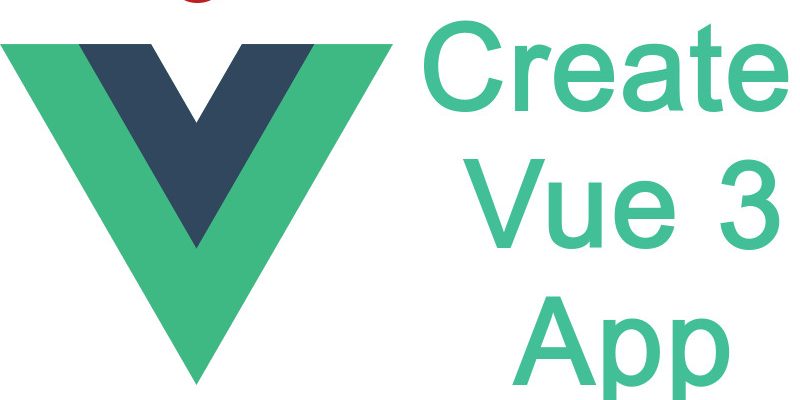
In this blog post i will describe three ways to initialize and create a Vue3 application using manual setup, cli or Vite as the process of creating Vuejs 3 apps is different from Vuejs 2.
There are three methods for creating Vue3 applications, which include manual setup, use of the vue-cli, or using vite. Let’s go over each method separately.
Manual Setup
Using this method we have to prepare the project dependencies from scratch. So let’s create a new folder project named “my-vue-app” with this structure:
- components/ directory
- package.json
- webpack.config.js
- app.js
- App.vue
- index.html
Open package.json and add this code:
{ "name": "my-vue-app", "version": "1.0.0", "description": "", "main": "app.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1", "dev": "webpack" }, "author": "", "license": "ISC", "dependencies": { "@vue/compiler-sfc": "^3.0.0-alpha.11", "css-loader": "^5.2.0", "vue": "^3.0.11", "vue-style-loader": "^4.1.3", "webpack": "^4.0.0" }, "devDependencies": { "vue-loader": "^16.0.0-alpha.3", "webpack-cli": "^4.6.0" } }
Then cd into the project root and run:
npm install
Here we are installing Vue3 the latest vue version. The @vue/compiler-sfc and vue-loader responsible for compiling and loading single file components. The vue-style-loader and css-loader responsible for loading the <style> and css files inside vue components. Finally webpack and webpack-cli required to bundle and build the project.
Next open webpack.config.js and this code:
const path = require('path'); const { VueLoaderPlugin } = require('vue-loader'); module.exports = { entry: './app.js', output: { path: path.resolve(__dirname, './dist'), filename: 'app.js', }, mode: 'development', module: { rules: [ { test: /\.vue$/, loader: 'vue-loader' }, { test: /\.css$/, use: [ 'vue-style-loader', 'css-loader' ] } ] }, plugins: [ new VueLoaderPlugin() ] }
If you are not familiar with webpack, webpack is a bundler tool which bundles and build Ecmascript, sass, css into understandable code to be run in browsers for example this Ecmascript 6 code:
import bar from './bar.js'; bar();
This code won’t work in most latest browsers, so to make it work in all the common browsers we need a way to convert this code into plain javascript to be run so webpack is used for these situations. There are also other bundlers like babel.
In the above configuration we tell webpack:
- The Entry file be app.js
- The output will be in dist/app.js, we don’t have to create the dist/ directory explicitly as webpack will create it automatically.
- Set the mode to “development”, for production deploy set it to “production”
- The module tells webpack about the rules to apply like applying vue-loader on .vue files.
After these config is set let’s update the main component App.vue.
App.vue
<template> <Home /> </template> <script> import Home from "./components/Home.vue"; export default { components: { Home } } </script>
In this component i invoked another child component <Home /> in the components/ directory, so let’s create it.
components/Home.vue
<template> <h2>I am a {{ title }}</h2> </template> <script> export default { data() { return { title: "Vue App" } } } </script> <style scoped> h2 { font-family: 'Times New Roman', Times, serif; font-size: 100px; font-style: italic; color: rgb(117, 22, 43); text-align: center; margin-top: 50px; } </style>
Now update the app.js by mounting the App component
app.js
import {createApp} from "vue"; import App from "./App.vue"; createApp(App).mount("#app");
This is the new syntax in Vue3 which inherited from React. In this case we imported the new createApp function, then i called createApp(App).mount(selector), this selector will be an html element in the index.html.
Then in the terminal run:
npm run dev
This command will generate the dist/ directory along with the created app.js. Now update the index.html file and load the app.js script.
index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8" /> <title>Vue app</title> </head> <body> <div id="app"></div> <script src="./dist/app.js"></script> </body> </html>
Now you can run the application in the browser and see the output. Note that you need to run it through http server to work properly, in my case i am using apache or you can install the webpack-dev-server.
Using vue-cli
If you don’t want to create the application from scratch, you can use the vue-cli tool to scaffold an application with zero configuation.
At first let’s install the vue-cli globally:
npm install -g @vue/cli // or yarn global add @vue/cli
After installation you can check the vue binary in command line by running:
vue --version
To create a project:
vue create my-app
This command will ask you some questions like “selecting a preset”, you can select the default present which comes with (babel, eslint) or you can pick the features manually. The features include Typescript, PWA, Router, Vuex, etc. Once finish it will start installing the features and it’s ready for launch.
Launch the app with:
npm run serve
This will fire a vue dev server on port 8080.
There is also a great tool included with vue-cli is the vue ui which enables to create and manage any vue app using GUI. To use it run this command:
vue ui
This command will open a browser window with GUI to create a vue app, it’s like the command line but in graphical interface. You can also view any created vue application and manage it’s dependencies. Visit the vue-cli docs in this link.
Using vite
The third method to create a vue app by using the vite tool, vite is a web development build tool that used to generate a ready to use app with less much effort.
To create a project with vite run those commands in terminal:
npm init vite-app my-app cd my-app npm install npm run dev
This will launch a vue app, typically on port 3000. To learn more about vite visit https://vitejs.dev.