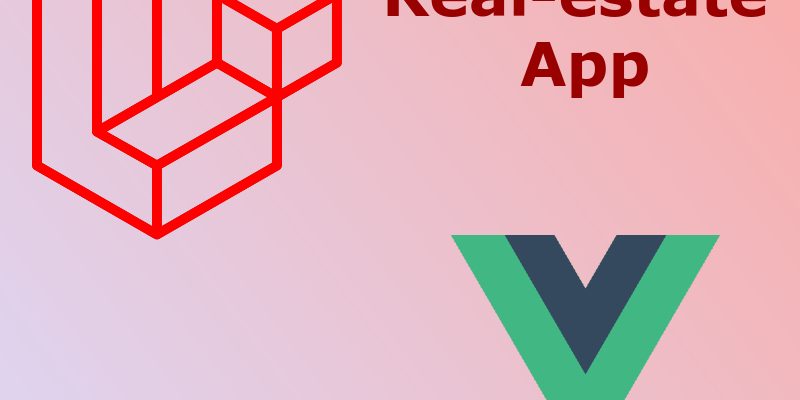
In the first part of this series we will prepare a skeleton Laravel application. Then we will download and install the html template we will be using in this tutorial.
Let’s start by creating a fresh laravel project, at the time of writing this article i am using the latest version of laravel which is laravel 9.
composer create-project laravel/laravel realestateapp --prefer-dist
After successful installation cd into the project and install the npm dependencies:
cd realestateapp npm install
Next install the Vue 3 dependencies:
npm install --save vue@next && npm install --save-dev vue-loader@next
After that configure webpack.mix.js to invoke the .vue() method like so:
webpack.mix.js
const mix = require('laravel-mix'); mix.js('resources/js/app.js', 'public/js') .vue() .postCss('resources/css/app.css', 'public/css', [ // ]);
Preparing Vue 3 app
We will continue by preparing a Vue 3 app in the Laravel project. So inside resources/js create a new directory called components/ and inside it just create a test component:
resources/js/components/Hello.vue
<template> {{title}} </template> <script> export default { name: "Hello", setup() { const title = "Hello world"; return { title } } } </script>
This is a test component to preview that the app is working fine, and we will remove it later. As you see i am using the setup() function from the Vue 3 composition api.
Now initialize the Vue 3 app inside app.js
resources/js/app.js
require('./bootstrap'); import { createApp } from 'vue'; import Hello from './components/Hello.vue'; const app = createApp({}); app.component('hello', Hello); app.mount('#app');
In this code we import the createApp named exported function from Vue 3 and then mounts it to the “#app” selector.
Then open the welcome.blade.php laravel view and update the <body/> content like shown below:
<body class="antialiased"> <div id="app"> <hello/> </div> <script src="{{ mix('js/app.js') }}"></script> </body>
Next compile the javascript assets using:
npm run watch
And finally launch the app:
php artisan serve
Now check http://localhost:8000
In the next step we will download and integrate the template.
Preparing The Template
I will use a ready made html template built using bootstrap, you can download it through this link.
After downloading the template extract it and copy the assets/ and bootstrap/ directory into a new directory public/template/
Rename welcome.blade.php to app.blade.php and update it like so:
app.blade.php
<!DOCTYPE html> <html lang="{{ str_replace('_', '-', app()->getLocale()) }}"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Real estate app</title> <link href='http://fonts.googleapis.com/css?family=Open+Sans:400,300,700,800' rel='stylesheet' type='text/css'> <link rel="stylesheet" href="{{asset('template')}}/assets/css/normalize.css"> <link rel="stylesheet" href="{{asset('template')}}/assets/css/font-awesome.min.css"> <link rel="stylesheet" href="{{asset('template')}}/assets/css/fontello.css"> <link href="{{asset('template')}}/assets/fonts/icon-7-stroke/css/pe-icon-7-stroke.css" rel="stylesheet"> <link href="{{asset('template')}}/assets/fonts/icon-7-stroke/css/helper.css" rel="stylesheet"> <link href="{{asset('template')}}/assets/css/animate.css" rel="stylesheet" media="screen"> <link rel="stylesheet" href="{{asset('template')}}/assets/css/bootstrap-select.min.css"> <link rel="stylesheet" href="{{asset('template')}}/bootstrap/css/bootstrap.min.css"> <link rel="stylesheet" href="{{asset('template')}}/assets/css/icheck.min_all.css"> <link rel="stylesheet" href="{{asset('template')}}/assets/css/price-range.css"> <link rel="stylesheet" href="{{asset('template')}}/assets/css/owl.carousel.css"> <link rel="stylesheet" href="{{asset('template')}}/assets/css/owl.theme.css"> <link rel="stylesheet" href="{{asset('template')}}/assets/css/owl.transitions.css"> <link rel="stylesheet" href="{{asset('template')}}/assets/css/wizard.css" /> <link rel="stylesheet" href="{{asset('template')}}/assets/css/lightslider.min.css" /> <link rel="stylesheet" href="{{asset('template')}}/assets/css/style.css"> <link rel="stylesheet" href="{{asset('template')}}/assets/css/responsive.css"> <link href="{{ mix('css/app.css') }}" type="text/css" rel="stylesheet" /> <script> window._base_url = '{{url('/')}}'; window._asset = '{{asset('')}}'; </script> </head> <body> <div id="app"> <app/> </div> <script src="{{asset('template')}}/assets/js/modernizr-2.6.2.min.js"></script> <script src="{{asset('template')}}/assets/js/jquery-1.10.2.min.js"></script> <script src="{{asset('template')}}/assets/js/bootstrap.min.js" type="text/javascript"></script> <script src="{{asset('template')}}/assets/js/bootstrap-select.min.js"></script> <script src="{{asset('template')}}/assets/js/bootstrap-hover-dropdown.js"></script> <script src="{{asset('template')}}/assets/js/easypiechart.min.js"></script> <script src="{{asset('template')}}/assets/js/jquery.easypiechart.min.js"></script> <script src="{{asset('template')}}/assets/js/owl.carousel.min.js"></script> <script src="{{asset('template')}}/assets/js/wow.js"></script> <script src="{{asset('template')}}/assets/js/icheck.min.js"></script> <script src="{{asset('template')}}/assets/js/price-range.js"></script> <script type="text/javascript" src="{{asset('template')}}/assets/js/lightslider.min.js"></script> <script src="{{asset('template')}}/assets/js/main.js"></script> <script src="{{ mix('js/app.js') }}"></script> </body> </html>
Update routes/web.php
<?php use Illuminate\Support\Facades\Route; Route::get('/{path?}', function () { return view('app'); })->where('path', '^(?!api).*$');
The app.blade.php view as the you might guess will hold the vuejs app, for this reason i updated the route as shown above using where() expression to tell laravel that this is a vue route not laravel route.
Now run
npm run dev
Inspect the browser console to check that the scripts and styles loaded correctly. If everything fine then we are ready to go to the next step.
Installing Vue Router and Setting The Pages
Below we will extract the required pages from the template we just downloaded and add to each Vue page as we see later.
Like many applications we will have different pages for our app. To make it easy to navigate to each page we need some sort of router.
So first install the Vue Router:
npm install vue-router@4
Next create a new directory called pages/ inside of resources/js/components/
Inside of the pages/ directory create these components as in this figure:
Using Vue router i have added the required routes in resources/js/routes.js
resources/js/routes.js
import {createRouter, createWebHistory} from "vue-router"; import Home from "./components/pages/Home"; import Properties from "./components/pages/Properties"; import Contact from "./components/pages/Contact"; import PropertySingle from "./components/pages/PropertySingle"; import Register from "./components/pages/Register"; import SubmitProperty from "./components/pages/SubmitProperty"; import EditProfile from "./components/pages/user/EditProfile"; import ChangePassword from "./components/pages/user/ChangePassword"; import MyProperties from "./components/pages/user/MyProperties"; import Favorites from "./components/pages/user/Favorites"; import EditProperty from "./components/pages/user/EditProperty"; const routes = [ {path: '/', component: Home}, {path: '/properties', component: Properties}, {path: '/register', component: Register}, {path: '/property/:id/:slug', component: PropertySingle}, {path: '/submit-property', component: SubmitProperty}, {path: '/contact', component: Contact}, {path: '/user/edit-profile', component: EditProfile}, {path: '/user/change-password', component: ChangePassword}, {path: '/user/my-properties', component: MyProperties}, {path: '/user/edit-property/:id', component: EditProperty}, {path: '/user/fav', component: Favorites}, ]; export default createRouter({ history: createWebHistory(), routes });
Also remove the <Hello /> component created earlier and add a new component <App />. This component serve as the root component for our app.
resources/js/components/App.vue
<template> <div id="preloader"> <div id="status"> </div> </div> <Menu /> <router-view></router-view> <Footer /> </template> <script> import Menu from "./partials/Menu"; import Footer from "./partials/Footer"; export default { name: "App", components: {Footer, Menu}, setup() { return { } } } </script>
The purpose of the <App /> component is to render the required page based on the current route using the <router-view /> component. As you see i have add two partial components <Menu /> and <Footer />.
Extract the menu nav from the template into the below Menu.vue like so:
resources/js/components/partials/Menu.vue
<template> <nav class="navbar navbar-default "> <div class="container"> <!-- Brand and toggle get grouped for better mobile display --> <div class="navbar-header"> <button type="button" class="navbar-toggle collapsed" data-toggle="collapse" data-target="#navigation"> <span class="sr-only">Toggle navigation</span> <span class="icon-bar"></span> <span class="icon-bar"></span> <span class="icon-bar"></span> </button> <router-link to="/" class="navbar-brand"><img src="/template/assets/img/logo.png" alt=""></router-link> </div> <!-- Collect the nav links, forms, and other content for toggling --> <div class="collapse navbar-collapse yamm" id="navigation"> <div class="button navbar-right"> <button class="navbar-btn nav-button wow bounceInRight login" @click="$router.push('/register')" data-wow-delay="0.4s">Login</button> <button class="navbar-btn nav-button wow fadeInRight" @click="$router.push('/submit-property')" data-wow-delay="0.5s">Submit</button> </div> <ul class="main-nav nav navbar-nav navbar-right"> <li class="ymm-sw " data-wow-delay="0.1s"> <router-link to="/">Home</router-link> </li> <li class="wow fadeInDown" data-wow-delay="0.1s"> <router-link to="/properties">Properties</router-link> </li> <li class="wow fadeInDown" data-wow-delay="0.4s"> <router-link to="/contact">Contact</router-link> </li> </ul> </div><!-- /.navbar-collapse --> </div><!-- /.container-fluid --> </nav> </template> <script> export default { name: "Menu" } </script> <style scoped> </style>
In the same manner extract the footer section from the template into the below Footer.vue like so:
resources/js/components/partials/Footer.vue
<template> <div class="footer-area"> <div class=" footer"> <div class="container"> <div class="row"> <div class="col-md-3 col-sm-6 wow fadeInRight animated"> <div class="single-footer"> <h4>About us </h4> <div class="footer-title-line"></div> <img src="assets/img/footer-logo.png" alt="" class="wow pulse" data-wow-delay="1s"> <p>Lorem ipsum dolor cum necessitatibus su quisquam molestias. Vel unde, blanditiis.</p> <ul class="footer-adress"> <li><i class="pe-7s-map-marker strong"> </i> 9089 your adress her</li> <li><i class="pe-7s-mail strong"> </i> email@yourcompany.com</li> <li><i class="pe-7s-call strong"> </i> +1 908 967 5906</li> </ul> </div> </div> <div class="col-md-3 col-sm-6 wow fadeInRight animated"> <div class="single-footer"> <h4>Quick links </h4> <div class="footer-title-line"></div> <ul class="footer-menu"> <li><router-link to="/properties">Properties</router-link> </li> <li><router-link to="/submit-property">Submit property </router-link></li> <li><router-link to="/contact">Contact us</router-link></li> <li><router-link to="register">Create account</router-link> </li> </ul> </div> </div> <div class="col-md-3 col-sm-6 wow fadeInRight animated"> <div class="single-footer news-letter"> <h4>Stay in touch</h4> <div class="footer-title-line"></div> <p>Lorem ipsum dolor sit amet, nulla suscipit similique quisquam molestias. Vel unde, blanditiis.</p> <form> <div class="input-group"> <input class="form-control" type="text" placeholder="E-mail ... "> <span class="input-group-btn"> <button class="btn btn-primary subscribe" type="button"><i class="pe-7s-paper-plane pe-2x"></i></button> </span> </div> <!-- /input-group --> </form> </div> </div> </div> </div> </div> <div class="footer-copy text-center"> <div class="container"> <div class="row"> <div class="pull-left"> <span> (C) <a href="http://www.KimaroTec.com">KimaroTheme</a> , All rights reserved {{ (new Date()).getFullYear()}} </span> </div> <div class="bottom-menu pull-right"> <ul> <li><router-link to="/" class="wow fadeInUp animated" data-wow-delay="0.2s">Home</router-link></li> <li><router-link to="/contact" class="wow fadeInUp animated" data-wow-delay="0.6s">Contact</router-link></li> </ul> </div> </div> </div> </div> </div> </template> <script> export default { name: "Footer" } </script> <style scoped> </style>
Extract the homepage content from the template into the below Home.vue:
Update resources/js/components/pages/Home.vue with the below code:
<template> <div class="slider-area"> <div class="slider"> <div id="bg-slider" class="owl-carousel owl-theme"> <div class="item"><img src="/template/assets/img/slide1/slider-image-1.jpg" alt="Mirror Edge"></div> <div class="item"><img src="/template/assets/img/slide1/slider-image-2.jpg" alt="The Last of us"></div> <div class="item"><img src="/template/assets/img/slide1/slider-image-4.jpg" alt="GTA V"></div> </div> </div> <div class="container slider-content"> <div class="row"> <div class="col-lg-8 col-lg-offset-2 col-md-10 col-md-offset-1 col-sm-12"> <h2>property Searching Just Got So Easy</h2> <p>Lorem ipsum dolor sit amet, consectetur adipisicing elit. Eligendi deserunt deleniti, ullam commodi sit ipsam laboriosam velit adipisci quibusdam aliquam teneturo!</p> <div class="search-form wow pulse" data-wow-delay="0.8s"> <form action="" class=" form-inline"> <button class="btn toggle-btn" type="button"><i class="fa fa-bars"></i></button> <div class="form-group"> <input type="text" class="form-control" placeholder="Key word"> </div> <div class="form-group"> <select id="lunchBegins" class="selectpicker" data-live-search="true" data-live-search-style="begins" title="Select your city"> <option>New york, CA</option> <option>Paris</option> <option>Casablanca</option> <option>Tokyo</option> <option>Marraekch</option> <option>kyoto , shibua</option> </select> </div> <div class="form-group"> <select id="basic" class="selectpicker show-tick form-control"> <option> -Status- </option> <option>Rent </option> <option>Boy</option> <option>used</option> </select> </div> <button class="btn search-btn" type="submit"><i class="fa fa-search"></i></button> <div style="display: none;" class="search-toggle"> <div class="search-row"> <div class="form-group mar-r-20"> <label for="price-range">Price range ($):</label> <input type="text" class="span2" value="" data-slider-min="0" data-slider-max="600" data-slider-step="5" data-slider-value="[0,450]" id="price-range" ><br /> <b class="pull-left color">2000$</b> <b class="pull-right color">100000$</b> </div> <!-- End of --> <div class="form-group mar-l-20"> <label for="property-geo">Property geo (m2) :</label> <input type="text" class="span2" value="" data-slider-min="0" data-slider-max="600" data-slider-step="5" data-slider-value="[50,450]" id="property-geo" ><br /> <b class="pull-left color">40m</b> <b class="pull-right color">12000m</b> </div> <!-- End of --> </div> <div class="search-row"> <div class="form-group mar-r-20"> <label for="price-range">Min baths :</label> <input type="text" class="span2" value="" data-slider-min="0" data-slider-max="600" data-slider-step="5" data-slider-value="[250,450]" id="min-baths" ><br /> <b class="pull-left color">1</b> <b class="pull-right color">120</b> </div> <!-- End of --> <div class="form-group mar-l-20"> <label for="property-geo">Min bed :</label> <input type="text" class="span2" value="" data-slider-min="0" data-slider-max="600" data-slider-step="5" data-slider-value="[250,450]" id="min-bed" ><br /> <b class="pull-left color">1</b> <b class="pull-right color">120</b> </div> <!-- End of --> </div> <br> <div class="search-row"> <div class="form-group"> <div class="checkbox"> <label> <input type="checkbox"> Fire Place(3100) </label> </div> </div> <!-- End of --> <div class="form-group"> <div class="checkbox"> <label> <input type="checkbox"> Dual Sinks(500) </label> </div> </div> <!-- End of --> <div class="form-group"> <div class="checkbox"> <label> <input type="checkbox"> Hurricane Shutters(99) </label> </div> </div> <!-- End of --> </div> <div class="search-row"> <div class="form-group"> <div class="checkbox"> <label> <input type="checkbox"> Swimming Pool(1190) </label> </div> </div> <!-- End of --> <div class="form-group"> <div class="checkbox"> <label> <input type="checkbox"> 2 Stories(4600) </label> </div> </div> <!-- End of --> <div class="form-group"> <div class="checkbox"> <label> <input type="checkbox"> Emergency Exit(200) </label> </div> </div> <!-- End of --> </div> <div class="search-row"> <div class="form-group"> <div class="checkbox"> <label> <input type="checkbox"> Laundry Room(10073) </label> </div> </div> <!-- End of --> <div class="form-group"> <div class="checkbox"> <label> <input type="checkbox"> Jog Path(1503) </label> </div> </div> <!-- End of --> <div class="form-group"> <div class="checkbox"> <label> <input type="checkbox"> 26' Ceilings(1200) </label> </div> </div> <!-- End of --> </div> </div> </form> </div> </div> </div> </div> </div> <!-- property area --> <div class="content-area recent-property" style="padding-bottom: 60px; background-color: rgb(252, 252, 252);"> <div class="container"> <div class="row"> <div class="col-md-12 padding-top-40 properties-page"> <div class="col-md-12 "> <div class="col-xs-10 page-subheader sorting pl0"> <ul class="sort-by-list"> <li class="active"> <a href="javascript:void(0);" class="order_by_date" data-orderby="property_date" data-order="ASC"> Property Date <i class="fa fa-sort-amount-asc"></i> </a> </li> <li class=""> <a href="javascript:void(0);" class="order_by_price" data-orderby="property_price" data-order="DESC"> Property Price <i class="fa fa-sort-numeric-desc"></i> </a> </li> </ul> <div class="items-per-page"> <label for="items_per_page"><b>Property per page :</b></label> <div class="sel"> <select id="items_per_page" name="per_page"> <option value="3">3</option> <option value="6">6</option> <option value="9">9</option> <option selected="selected" value="12">12</option> <option value="15">15</option> <option value="30">30</option> <option value="45">45</option> <option value="60">60</option> </select> </div> </div> </div> <div class="col-xs-2 layout-switcher"> <a class="layout-list" href="javascript:void(0);"> <i class="fa fa-th-list"></i> </a> <a class="layout-grid active" style="margin-left: 5px" href="javascript:void(0);"> <i class="fa fa-th"></i> </a> </div><!--/ .layout-switcher--> </div> <div class="col-md-12 "> <div id="list-type" class="proerty-th"> <div class="col-sm-6 col-md-3 p0"> <div class="box-two proerty-item"> <div class="item-thumb"> <a href="property-1.html" ><img src="/template/assets/img/demo/property-3.jpg"></a> </div> <div class="item-entry overflow"> <h5><a href="property-1.html"> Super nice villa </a></h5> <div class="dot-hr"></div> <span class="pull-left"><b> Area :</b> 120m </span> <span class="proerty-price pull-right"> $ 300,000</span> <p style="display: none;">Suspendisse ultricies Suspendisse ultricies Nulla quis dapibus nisl. Suspendisse ultricies commodo arcu nec pretium ...</p> <div class="property-icon"> <img src="/template/assets/img/icon/bed.png">(5)| <img src="/template/assets/img/icon/shawer.png">(2)| <img src="/template/assets/img/icon/cars.png">(1) </div> </div> </div> </div> <div class="col-sm-6 col-md-3 p0"> <div class="box-two proerty-item"> <div class="item-thumb"> <a href="property-1.html" ><img src="/template/assets/img/demo/property-2.jpg"></a> </div> <div class="item-entry overflow"> <h5><a href="property-1.html"> Super nice villa </a></h5> <div class="dot-hr"></div> <span class="pull-left"><b> Area :</b> 120m </span> <span class="proerty-price pull-right"> $ 300,000</span> <p style="display: none;">Suspendisse ultricies Suspendisse ultricies Nulla quis dapibus nisl. Suspendisse ultricies commodo arcu nec pretium ...</p> <div class="property-icon"> <img src="/template/assets/img/icon/bed.png">(5)| <img src="/template/assets/img/icon/shawer.png">(2)| <img src="/template/assets/img/icon/cars.png">(1) </div> </div> </div> </div> <div class="col-sm-6 col-md-3 p0"> <div class="box-two proerty-item"> <div class="item-thumb"> <a href="property-1.html" ><img src="/template/assets/img/demo/property-1.jpg"></a> </div> <div class="item-entry overflow"> <h5><a href="property-1.html"> Super nice villa </a></h5> <div class="dot-hr"></div> <span class="pull-left"><b> Area :</b> 120m </span> <span class="proerty-price pull-right"> $ 300,000</span> <p style="display: none;">Suspendisse ultricies Suspendisse ultricies Nulla quis dapibus nisl. Suspendisse ultricies commodo arcu nec pretium ...</p> <div class="property-icon"> <img src="/template/assets/img/icon/bed.png">(5)| <img src="/template/assets/img/icon/shawer.png">(2)| <img src="/template/assets/img/icon/cars.png">(1) </div> </div> </div> </div> <div class="col-sm-6 col-md-3 p0"> <div class="box-two proerty-item"> <div class="item-thumb"> <a href="property-1.html" ><img src="/template/assets/img/demo/property-3.jpg"></a> </div> <div class="item-entry overflow"> <h5><a href="property-1.html"> Super nice villa </a></h5> <div class="dot-hr"></div> <span class="pull-left"><b> Area :</b> 120m </span> <span class="proerty-price pull-right"> $ 300,000</span> <p style="display: none;">Suspendisse ultricies Suspendisse ultricies Nulla quis dapibus nisl. Suspendisse ultricies commodo arcu nec pretium ...</p> <div class="property-icon"> <img src="/template/assets/img/icon/bed.png">(5)| <img src="/template/assets/img/icon/shawer.png">(2)| <img src="/template/assets/img/icon/cars.png">(1) </div> </div> </div> </div> <div class="col-sm-6 col-md-3 p0"> <div class="box-two proerty-item"> <div class="item-thumb"> <a href="property-1.html" ><img src="/template/assets/img/demo/property-1.jpg"></a> </div> <div class="item-entry overflow"> <h5><a href="property-1.html"> Super nice villa </a></h5> <div class="dot-hr"></div> <span class="pull-left"><b> Area :</b> 120m </span> <span class="proerty-price pull-right"> $ 300,000</span> <p style="display: none;">Suspendisse ultricies Suspendisse ultricies Nulla quis dapibus nisl. Suspendisse ultricies commodo arcu nec pretium ...</p> <div class="property-icon"> <img src="/template/assets/img/icon/bed.png">(5)| <img src="/template/assets/img/icon/shawer.png">(2)| <img src="/template/assets/img/icon/cars.png">(1) </div> </div> </div> </div> <div class="col-sm-6 col-md-3 p0"> <div class="box-two proerty-item"> <div class="item-thumb"> <a href="property-1.html" ><img src="/template/assets/img/demo/property-2.jpg"></a> </div> <div class="item-entry overflow"> <h5><a href="property-1.html"> Super nice villa </a></h5> <div class="dot-hr"></div> <span class="pull-left"><b> Area :</b> 120m </span> <span class="proerty-price pull-right"> $ 300,000</span> <p style="display: none;">Suspendisse ultricies Suspendisse ultricies Nulla quis dapibus nisl. Suspendisse ultricies commodo arcu nec pretium ...</p> <div class="property-icon"> <img src="/template/assets/img/icon/bed.png">(5)| <img src="/template/assets/img/icon/shawer.png">(2)| <img src="/template/assets/img/icon/cars.png">(1) </div> </div> </div> </div> <div class="col-sm-6 col-md-3 p0"> <div class="box-two proerty-item"> <div class="item-thumb"> <a href="property-1.html" ><img src="/template/assets/img/demo/property-3.jpg"></a> </div> <div class="item-entry overflow"> <h5><a href="property-1.html"> Super nice villa </a></h5> <div class="dot-hr"></div> <span class="pull-left"><b> Area :</b> 120m </span> <span class="proerty-price pull-right"> $ 300,000</span> <p style="display: none;">Suspendisse ultricies Suspendisse ultricies Nulla quis dapibus nisl. Suspendisse ultricies commodo arcu nec pretium ...</p> <div class="property-icon"> <img src="/template/assets/img/icon/bed.png">(5)| <img src="/template/assets/img/icon/shawer.png">(2)| <img src="/template/assets/img/icon/cars.png">(1) </div> </div> </div> </div> <div class="col-sm-6 col-md-3 p0"> <div class="box-two proerty-item"> <div class="item-thumb"> <a href="property-1.html" ><img src="/template/assets/img/demo/property-2.jpg"></a> </div> <div class="item-entry overflow"> <h5><a href="property-1.html"> Super nice villa </a></h5> <div class="dot-hr"></div> <span class="pull-left"><b> Area :</b> 120m </span> <span class="proerty-price pull-right"> $ 300,000</span> <p style="display: none;">Suspendisse ultricies Suspendisse ultricies Nulla quis dapibus nisl. Suspendisse ultricies commodo arcu nec pretium ...</p> <div class="property-icon"> <img src="/template/assets/img/icon/bed.png">(5)| <img src="/template/assets/img/icon/shawer.png">(2)| <img src="/template/assets/img/icon/cars.png">(1) </div> </div> </div> </div> <div class="col-sm-6 col-md-3 p0"> <div class="box-two proerty-item"> <div class="item-thumb"> <a href="property-1.html" ><img src="/template/assets/img/demo/property-1.jpg"></a> </div> <div class="item-entry overflow"> <h5><a href="property-1.html"> Super nice villa </a></h5> <div class="dot-hr"></div> <span class="pull-left"><b> Area :</b> 120m </span> <span class="proerty-price pull-right"> $ 300,000</span> <p style="display: none;">Suspendisse ultricies Suspendisse ultricies Nulla quis dapibus nisl. Suspendisse ultricies commodo arcu nec pretium ...</p> <div class="property-icon"> <img src="/template/assets/img/icon/bed.png">(5)| <img src="/template/assets/img/icon/shawer.png">(2)| <img src="/template/assets/img/icon/cars.png">(1) </div> </div> </div> </div> <div class="col-sm-6 col-md-3 p0"> <div class="box-two proerty-item"> <div class="item-thumb"> <a href="property-1.html" ><img src="/template/assets/img/demo/property-3.jpg"></a> </div> <div class="item-entry overflow"> <h5><a href="property-1.html"> Super nice villa </a></h5> <div class="dot-hr"></div> <span class="pull-left"><b> Area :</b> 120m </span> <span class="proerty-price pull-right"> $ 300,000</span> <p style="display: none;">Suspendisse ultricies Suspendisse ultricies Nulla quis dapibus nisl. Suspendisse ultricies commodo arcu nec pretium ...</p> <div class="property-icon"> <img src="/template/assets/img/icon/bed.png">(5)| <img src="/template/assets/img/icon/shawer.png">(2)| <img src="/template/assets/img/icon/cars.png">(1) </div> </div> </div> </div> <div class="col-sm-6 col-md-3 p0"> <div class="box-two proerty-item"> <div class="item-thumb"> <a href="property-1.html" ><img src="/template/assets/img/demo/property-2.jpg"></a> </div> <div class="item-entry overflow"> <h5><a href="property-1.html"> Super nice villa </a></h5> <div class="dot-hr"></div> <span class="pull-left"><b> Area :</b> 120m </span> <span class="proerty-price pull-right"> $ 300,000</span> <p style="display: none;">Suspendisse ultricies Suspendisse ultricies Nulla quis dapibus nisl. Suspendisse ultricies commodo arcu nec pretium ...</p> <div class="property-icon"> <img src="/template/assets/img/icon/bed.png">(5)| <img src="/template/assets/img/icon/shawer.png">(2)| <img src="/template/assets/img/icon/cars.png">(1) </div> </div> </div> </div> <div class="col-sm-6 col-md-3 p0"> <div class="box-two proerty-item"> <div class="item-thumb"> <a href="property-1.html" ><img src="/template/assets/img/demo/property-1.jpg"></a> </div> <div class="item-entry overflow"> <h5><a href="property-1.html"> Super nice villa </a></h5> <div class="dot-hr"></div> <span class="pull-left"><b> Area :</b> 120m </span> <span class="proerty-price pull-right"> $ 300,000</span> <p style="display: none;">Suspendisse ultricies Suspendisse ultricies Nulla quis dapibus nisl. Suspendisse ultricies commodo arcu nec pretium ...</p> <div class="property-icon"> <img src="/template/assets/img/icon/bed.png">(5)| <img src="/template/assets/img/icon/shawer.png">(2)| <img src="/template/assets/img/icon/cars.png">(1) </div> </div> </div> </div> </div> </div> </div> </div> </div> </div> </template> <script> export default { name: "Home" } </script> <style scoped> .page-subheader h4 { background-color: #FDC600; display: inline-block; padding: 5px 10px; color: #fff; font-weight: bold; font-size: 21px; } </style>
Finally update the app.js
resources/js/app.js
require('./bootstrap'); import { createApp } from 'vue'; import App from './components/App.vue'; import router from "./routes"; const app = createApp(App); app.use(router); app.mount('#app');
After this setup run:
npm run watch
Check http://localhost:8000 you should see the homepage.
Fixing Home Slider
An issue usually happens when navigating between pages and returning to the homepage is that the slider not loaded correctly and a white section appears instead. To resolve this issue is to call the slider jquery script located in the template main.js file and to load that script in the mounted hook of the page like so:
export default { name: "Home", mounted() { $(document).ready(function () { $("#bg-slider").owlCarousel({ // initialize the home slider navigation: false, // Show next and prev buttons slideSpeed: 100, autoPlay: 5000, paginationSpeed: 100, singleItem: true, mouseDrag: false, transitionStyle: "fade" }); }); } }
Continue to part 2: Database>>>