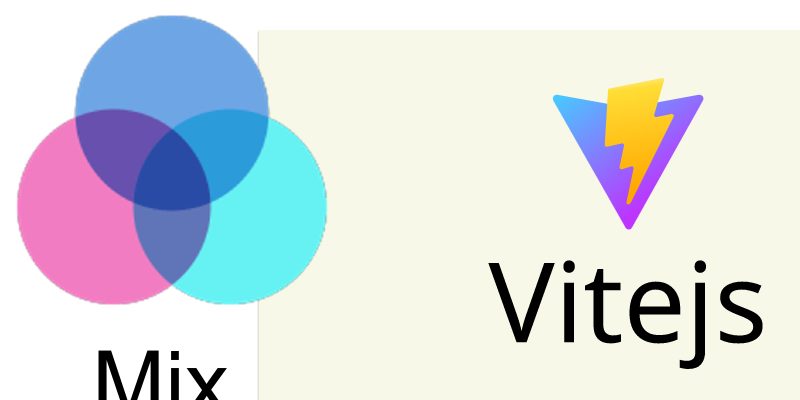
Latest versions of Laravel framework comes with a new frontend tooling which is the vite, in this post let’s see how to migrate from Webpack to using Vite.
Vite is the new frontend tooling and bundler for latest versions of laravel framework. The new library is the new generation frontend tooling and has a straightforward documentation and more easy to learn.
Compared to other tools, vite provides improved performance, fast browser updates, and many other advantages you can learn more on Vite website.
To migrate your existing laravel project to use Vite there are two methods:
- Automatic Migration Using Laravelshift service.
- Manual Migration.
First Method: Using Laravel Shift
The Laravelshift is a third party service which you can use it for free to migrate the laravel project to use vite. To use laravel shift your project should be located in git repository like Github. Just go to this link and follow the steps by clicking the “Run Shift” button.
Second Method: Manual Migration
The manual migration method as you might guess includes installing the npm Vite dependencies, updating env variables, editing Vue components and so on by yourself.
Installing Vite Dependencies:
npm install --save-dev vite laravel-vite-plugin
In addition to that if you are using Vuejs then you have to install the vite plugin for vue:
npm install @vitejs/plugin-vue
Remove laravel mix:
npm remove laravel-mix
rm webpack.mix.js
Update package.json scripts:
Open package.json and remove the scripts related to laravel mix and add these scripts:
"scripts": { "dev": "vite", "build": "vite build" },
Update env variables:
Open .env file and replace the env variables related to laravel mix with Vite:
Remove:
MIX_PUSHER_APP_KEY="${PUSHER_APP_KEY}" MIX_PUSHER_APP_CLUSTER="${PUSHER_APP_CLUSTER}"
With:
VITE_PUSHER_APP_KEY="${PUSHER_APP_KEY}" VITE_PUSHER_APP_CLUSTER="${PUSHER_APP_CLUSTER}"
By the way if you want to read the environment variables in javascript i.e in resources/js instead of using process.env there is a new syntax import.meta.env, for example:
import.meta.env.VITE_PUSHER_APP_KEY
Setup Vite config file:
Vite has a new config file similar to webpack.mix.js. So in your project root create a new file vite.config.js with this code:
import laravel from 'laravel-vite-plugin' import {defineConfig} from 'vite' export default defineConfig({ plugins: [ laravel([ 'resources/css/app.css', 'resources/js/app.js', ]), ], });
If you are using Vuejs and tailwindcss then modify vite.config.js like so:
import { defineConfig } from 'vite'; import laravel from 'laravel-vite-plugin'; import vue from '@vitejs/plugin-vue'; import path from "path"; import tailwindcss from "tailwindcss"; export default defineConfig({ plugins: [ laravel({ input: [ 'resources/css/app.css', 'resources/js/app.js', ], refresh: true }), vue({ template: { transformAssetUrls: { // The Vue plugin will re-write asset URLs, when referenced // in Single File Components, to point to the Laravel web // server. Setting this to `null` allows the Laravel plugin // to instead re-write asset URLs to point to the Vite // server instead. base: null, // The Vue plugin will parse absolute URLs and treat them // as absolute paths to files on disk. Setting this to // `false` will leave absolute URLs un-touched so they can // reference assets in the public directory as expected. includeAbsolute: false, }, }, }), tailwindcss() ], resolve: { alias: { '@': path.resolve(__dirname, './resources/js'), }, } });
Using Vite in blade templates:
Vite comes with @vite() blade directive to load the laravel assets, so in your laravel template in the <head /> tag add this code to load the assets:
@vite(['resources/css/app.css', 'resources/js/app.js'])
And remove any call to the mix() function.
Replace require with import:
You need to make another update also regarding scripts in resources/js/app.js and resources/js/bootstrap.js. Replace the require() syntax with the import as Vite support ES Modules only.
import _ from "lodash"; window._ = _; import axios from "axios"; window.axios = axios; window.axios.defaults.headers.common['X-Requested-With'] = 'XMLHttpRequest'; import Echo from 'laravel-echo'; import Pusher from "pusher-js"; window.Pusher = Pusher;
You have to do the same also if you are using Vue or React components.
Running Vite:
Now to run vite in the terminal :
npm run dev
And to build the assets for production deploy run:
npm run build