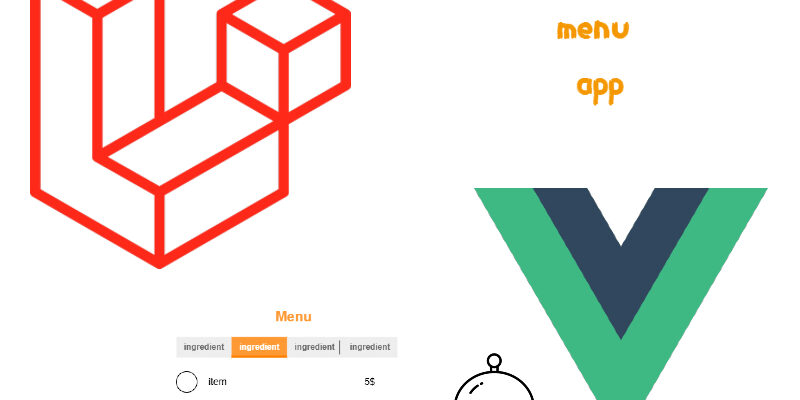
In this tutorial we will create a restaurant menu app using Laravel, Inertia and Vuejs 3, in which user can add the menu sections and menu items along with item description and price.
This tutorial is written using Laravel 12, you may encounter certain differences in packages setup and configurations from previous versions of laravel.
Creating Laravel Project
We will create a new laravel project for our app. You can use a laravel starter kit, however the starter kit contains many features like authorization and authentication and we don’t really need those features. So we will create a laravel project from scratch and install the necessary packages we just need:
composer create-project laravel/laravel laravel-vue-restaurant-menu
Install npm dependencies with npm install
After installing the npm dependencies let’s install the other required dependencies for our project, we will need tailwindcss, vue 3, inertiajs.
- Install Tailwind CSS and Flowbite using NPM:
npm install -D tailwindcss @tailwindcss/vite postcss autoprefixer flowbite
The flowbite package is a set of html components built on top tailwindcss like buttons, tables, forms, extra.
- Update app.css:
In resources/css/app.css add these lines:
@import 'tailwindcss'; @plugin "flowbite/plugin"; @source '../../vendor/laravel/framework/src/Illuminate/Pagination/resources/views/*.blade.php'; @source '../../storage/framework/views/*.php'; @source "../**/*.blade.php"; @source "../**/*.js"; @source "../**/*.vue"; @theme { --font-sans: 'Instrument Sans', ui-sans-serif, system-ui, sans-serif, 'Apple Color Emoji', 'Segoe UI Emoji', 'Segoe UI Symbol', 'Noto Color Emoji'; }
The @source directive to tell tailwind to scan the dedicated files and directories.
- Import Flowbite:
Import flowbite package inside resources/js/app.js so that we can use flowbite components:
import 'flowbite';
- Install inertiajs composer package:
composer require inertiajs/inertia-laravel
- Install inertiajs npm package along with vue:
npm install @inertiajs/vue3 vue @vitejs/plugin-vue
- Setup inertia middleware:
php artisan inertia:middleware
This command generates the HandleInertiaRequests
middleware class in app/Http/Middleware directory.
You need to register this middleware in bootstrap/app.php like so:
->withMiddleware(function (Middleware $middleware) { $middleware->web(append: [ App\Http\Middleware\HandleInertiaRequests::class, ]); })
- Update vite.config.js to include tailwindcss and vue:
vite.config.js
import { defineConfig } from 'vite' import tailwindcss from '@tailwindcss/vite'; // <------------------- import vue from '@vitejs/plugin-vue'; // <------------------- export default defineConfig({ plugins: [ tailwindcss(), // <------------------ vue(), // <------------------ // … ], })
- Create Layout File:
Create a new blade view that will act as the starting point to mount the vue inertia app:
resources/views/app.blade.php
<!doctype html> <html lang="{{ str_replace('_', '-', app()->getLocale()) }}"> <head> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Food Menu</title> @vite(['resources/css/app.css', 'resources/js/app.js']) @inertiaHead </head> <body> @inertia </body> </html>
- Initialize the inertia app:
Inside of resources/js/app.js add this code:
import './bootstrap'; import 'flowbite'; import { createApp, h } from 'vue'; import { createInertiaApp } from '@inertiajs/vue3'; createInertiaApp({ resolve: name => { const pages = import.meta.glob('./Pages/**/*.vue', { eager: true }) return pages[`./Pages/${name}.vue`] }, setup({ el, App, props, plugin }) { createApp({ render: () => h(App, props) }) .use(plugin) .mount(el) }, })
The resolve() callback receives a page name and loads the page component from the Pages directory.
Now start the build process using npm run dev
.
php artisan serve
. Now you will see the default welcome page of laravel, let’s display a vue page instead.<script setup> </script> <template> <h2>Restaurant Menu App</h2> </template>
Then update the default route to point to this page. Open routes/web.php and update as below:
<?php use Illuminate\Support\Facades\Route; Route::inertia('/', 'Home');
If you go to the browser you should see the text “Restaurant Menu App”.
Main Layout and Navbar
Let’s create a layout to wrap the application pages. Create a new directory Layout/ inside resources/js. Then add the Main layout like so:
resources/js/Layout/Main.vue
<script setup> import Navbar from "./Navbar.vue"; </script> <template> <main class="@container mx-20 max-md:mx-auto"> <header> <Navbar /> </header> <section class="p-4 pt-10"> <slot /> </section> </main> </template>
The Main layout file uses vue <slot />
element so that each page content will be replaced in this area. The <Navbar />
component contains the app logo and the navigation menu.
resources/js/Layout/Navbar.vue
<script setup> import { Link } from '@inertiajs/vue3' </script> <template> <nav class="bg-white border-gray-200 dark:bg-gray-900 dark:border-gray-700"> <div class="flex flex-wrap items-center justify-between p-4"> <Link href="/" class="flex items-center space-x-3 rtl:space-x-reverse"> <span class="self-center text-2xl font-semibold whitespace-nowrap text-orange-500 dark:text-white">Restaurant Menu</span> </Link> <button data-collapse-toggle="navbar-multi-level" type="button" class="inline-flex items-center p-2 w-10 h-10 justify-center text-sm text-gray-500 rounded-lg md:hidden hover:bg-gray-100 focus:outline-none focus:ring-2 focus:ring-gray-200 dark:text-gray-400 dark:hover:bg-gray-700 dark:focus:ring-gray-600" aria-controls="navbar-multi-level" aria-expanded="false"> <span class="sr-only">Open main menu</span> <svg class="w-5 h-5" aria-hidden="true" xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 17 14"> <path stroke="currentColor" stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M1 1h15M1 7h15M1 13h15"/> </svg> </button> <div class="hidden w-full md:block md:w-auto" id="navbar-multi-level"> <ul class="flex flex-col font-medium p-4 md:p-0 mt-4 border border-gray-100 rounded-lg bg-gray-50 md:space-x-8 rtl:space-x-reverse md:flex-row md:mt-0 md:border-0 md:bg-white dark:bg-gray-800 md:dark:bg-gray-900 dark:border-gray-700"> <li> <Link href="#" class="block py-2 px-3 text-white bg-blue-700 rounded-sm md:bg-transparent md:text-blue-700 md:p-0 md:dark:text-blue-500 dark:bg-blue-600 md:dark:bg-transparent" aria-current="page">Home</Link> </li> <li> <button id="dropdownNavbarLink" data-dropdown-toggle="dropdownNavbar" class="flex items-center justify-between w-full py-2 px-3 text-gray-900 hover:bg-gray-100 md:hover:bg-transparent md:border-0 md:hover:text-blue-700 md:p-0 md:w-auto dark:text-white md:dark:hover:text-blue-500 dark:focus:text-white dark:hover:bg-gray-700 md:dark:hover:bg-transparent">Manage <svg class="w-2.5 h-2.5 ms-2.5" aria-hidden="true" xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 10 6"> <path stroke="currentColor" stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="m1 1 4 4 4-4"/> </svg></button> <!-- Dropdown menu --> <div id="dropdownNavbar" class="z-10 hidden font-normal bg-white divide-y divide-gray-100 rounded-lg shadow-sm w-44 dark:bg-gray-700 dark:divide-gray-600"> <ul class="py-2 text-sm text-gray-700 dark:text-gray-200" aria-labelledby="dropdownLargeButton"> <li> <Link href="#" class="block px-4 py-2 hover:bg-gray-100 dark:hover:bg-gray-600 dark:hover:text-white">Menus</Link> </li> <li> <Link href="#" class="block px-4 py-2 hover:bg-gray-100 dark:hover:bg-gray-600 dark:hover:text-white">Sections</Link> </li> <li> <Link href="#" class="block px-4 py-2 hover:bg-gray-100 dark:hover:bg-gray-600 dark:hover:text-white">Menu Items</Link> </li> </ul> </div> </li> <li> <button data-dropdown-toggle="dropdownNavbar-all-menus" class="flex items-center justify-between w-full py-2 px-3 text-gray-900 hover:bg-gray-100 md:hover:bg-transparent md:border-0 md:hover:text-blue-700 md:p-0 md:w-auto dark:text-white md:dark:hover:text-blue-500 dark:focus:text-white dark:hover:bg-gray-700 md:dark:hover:bg-transparent">All Menus <svg class="w-2.5 h-2.5 ms-2.5" aria-hidden="true" xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 10 6"> <path stroke="currentColor" stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="m1 1 4 4 4-4"/> </svg> </button> <div id="dropdownNavbar-all-menus" class="z-10 hidden font-normal bg-white divide-y divide-gray-100 rounded-lg shadow-sm w-44 dark:bg-gray-700 dark:divide-gray-600"> <ul class="py-2 text-sm text-gray-700 dark:text-gray-200" aria-labelledby="dropdownLargeButton"> <li> <Link href="#" class="block px-4 py-2 hover:bg-gray-100 dark:hover:bg-gray-600 dark:hover:text-white">Menu 1</Link> </li> <li> <Link href="#" class="block px-4 py-2 hover:bg-gray-100 dark:hover:bg-gray-600 dark:hover:text-white">Menu 2</Link> </li> </ul> </div> </li> </ul> </div> </div> </nav> </template>
In the <Navbar />
component i added the code to display a navigation bar. This code taken from flowbite Navbar docs.
<Link />
inertia component at the script setup, then i replaced the anchor tags <a>
with the <Link>
inertia component in each li item.<script setup> import Main from '../Layout/Main.vue'; </script> <template> <Main> <h2>Restaurant Menu</h2> </Main> </template>
When running the application you will see the navbar as in this figure:
- Home: Displays the home page
- Manage: The dropdown menu that contains the administration links to create menu items, sections and menus.
- All Menus: The dropdown menu that display all create menu and a link to preview each menu.
Tables and Migrations
Update your project .env file and set the DB credentials:
.env
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3307 DB_DATABASE=laravel_vue_restaurant_menu DB_USERNAME=<username> DB_PASSWORD=<password>
Based to the above figure we will need these tables:
- Menus: Stores the menus.
- Sections: Stores food sections for each menu i.e burgers, meat, chicken, etc.
- Menu Items: Store menu items in each menu section.
- Menu_Section (Pivot): The intermediate table between Menu and Section as a many to many relationship.
- Section_Item (Pivot): The intermediate table between Section and Item as a many to many relationship.
Using laravel make:migration
command create these migrations:
php artisan make:migration create_menu_items_table php artisan make:migration create_sections_table php artisan make:migration create_menus_table php artisan make:migration create_menu_section_table php artisan make:migration create_section_item_table
Now open the migration files in database/migrations/ directory and update them as below:
*****_create_menu_items_table.php
<?php ..... ..... return new class extends Migration { /** * Run the migrations. */ public function up(): void { Schema::create('menu_items', function (Blueprint $table) { $table->id(); $table->string('title'); $table->string('price'); $table->string('image')->nullable(); $table->mediumText('description')->nullable(); $table->timestamps(); }); } .... .... };
*****_create_menu_sections_table.php
<?php ..... ..... return new class extends Migration { /** * Run the migrations. */ public function up(): void { Schema::create('sections', function (Blueprint $table) { $table->id(); $table->string('title'); $table->mediumText('description')->nullable(); $table->string('identifier', 100)->nullable()->comment('To uniquely identify the section if used in multiple menus'); $table->timestamps(); }); } .... .... };
*****_create_menus_table.php
<?php ..... ..... return new class extends Migration { /** * Run the migrations. */ public function up(): void { Schema::create('menus', function (Blueprint $table) { $table->id(); $table->string('title'); $table->mediumText('description')->nullable(); $table->timestamps(); }); } .... .... };
*****_create_menu_section_table.php
<?php ..... ..... return new class extends Migration { /** * Run the migrations. */ public function up(): void { Schema::create('menu_section', function (Blueprint $table) { $table->id(); $table->foreignId('menu_id')->constrained()->onDelete('cascade'); $table->foreignId('section_id')->constrained()->onDelete('cascade'); $table->timestamps(); }); } .... .... };
*****_create_section_item_table.php
<?php ..... ..... return new class extends Migration { /** * Run the migrations. */ public function up(): void { Schema::create('section_item', function (Blueprint $table) { $table->id(); $table->foreignId('section_id')->constrained()->onDelete('cascade'); $table->foreignId('item_id')->constrained('menu_items')->onDelete('cascade'); $table->timestamps(); }); } .... .... };
After updating each migration file, run the php artisan migrate
command in terminal to create the tables. You will be asked to create the database if not found, just hit `yes` and proceed.
Next create the models for these tables:
php artisan make:model Menu php artisan make:model Section php artisan make:model MenuItem
Open the created models and make the below updates:
app/Models/Menu.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Model; use Illuminate\Database\Eloquent\Relations\BelongsToMany; class Menu extends Model { /** * @var list<string> */ protected $fillable = [ "title", "description" ]; public function sections(): BelongsToMany { return $this->belongsToMany(Section::class); } }
I added the $fillable
property for mass assignment and declared the sections relation in sections() method. Let’s do the same for the other models.
app/Models/Section.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Model; use Illuminate\Database\Eloquent\Relations\BelongsToMany; class Section extends Model { /** * * @var list<string> */ protected $fillable = [ "title", "description", "identifier" ]; public function menus(): BelongsToMany { return $this->belongsToMany(Menu::class); } public function menuItems(): BelongsToMany { return $this->belongsToMany(MenuItem::class, 'section_item', 'section_id', 'item_id'); } }
app/Models/MenuItem.php
<?php namespace App\Models; use Illuminate\Database\Eloquent\Model; use Illuminate\Database\Eloquent\Relations\BelongsToMany; class MenuItem extends Model { protected $fillable = [ "title", "description", "price", "image" ]; protected $appends = [ 'image_url' ]; public function sections(): BelongsToMany { return $this->belongsToMany(Section::class, 'section_item', 'item_id', 'section_id'); } public function getImageUrlAttribute() { return $this->image ? url('/uploads/' . $this->image) : null; } }
As you see in the above models i added the relations needed between each model. In the MenuItem
model i added the getImageUrlAttribute()
method to return the image url which add a new attribute to the menu item responses and updated the $appends
property to include this attribute.
HandleInertiaRequestsMiddleware
The HandleInertiaRequestsMiddleware
is where you can add shared data that need to be accessed globally in the vue app, this is done in the share()
method:
public function share(Request $request): array { return array_merge(parent::share($request), [ 'flash' => [ 'success' => fn(): mixed => $request->session()->get('success'), 'error' => fn(): mixed => $request->session()->get('error') ] ]); }
In this case we are sharing the flash messages like success, error, etc when working with the controller actions later.
Helper Components
To maintain reusability i have created some helper components to be used in the vue app. These components will be located in resources/js/Components/ directory.
Components/FlashMessage.vue
<script setup> </script> <template> <div v-if="$page.props.flash.success" class="mt-2"> <div class="bg-green-100 border border-green-400 text-green-700 px-4 py-3 rounded relative" role="alert"> <strong class="font-bold">Success!</strong> <span class="block sm:inline mx-2">{{ $page.props.flash.success }}</span> </div> </div> <div v-if="$page.props.flash.error" class="mt-2"> <div class="bg-red-100 border border-red-400 text-red-700 px-4 py-3 rounded relative" role="alert"> <strong class="font-bold">Error!</strong> <span class="block sm:inline mx-2">{{ $page.props.flash.error }}</span> </div> </div> </template>
Components/InputError.vue
<script setup> defineProps({ errors: { type: Object, required: true }, field: { type: String, required: true } }); </script> <template> <div class="text-red-500 text-sm mt-2" v-if="errors[field]">{{ errors[field] }}</div> </template>
Components/Pagination.vue
<script setup> import { computed } from 'vue'; const props = defineProps({ currentPage: { type: Number, required: true }, totalPages: { type: Number, required: true }, maxVisibleButtons: { type: Number, default: 3 } }); const emit = defineEmits(['page-changed']); const pages = computed(() => { const range = []; const halfMaxVisibleButtons = Math.floor(props.maxVisibleButtons / 2); let start = Math.max(1, props.currentPage - halfMaxVisibleButtons); let end = Math.min(props.totalPages, start + props.maxVisibleButtons - 1); if (end - start + 1 < props.maxVisibleButtons) { start = Math.max(1, end - props.maxVisibleButtons + 1); } for (let i = start; i <= end; i++) { range.push(i); } return range; }); const changePage = (page) => { if (page !== props.currentPage && page >= 1 && page <= props.totalPages) { emit('page-changed', page); } }; </script> <template> <nav aria-label="Page navigation"> <ul class="inline-flex -space-x-px text-sm"> <!-- Previous Button --> <li> <a href="#" @click.prevent="changePage(currentPage - 1)" :class="{ 'cursor-not-allowed opacity-50': currentPage === 1 }" class="flex items-center justify-center px-3 h-8 ms-0 leading-tight text-gray-500 bg-white border border-e-0 border-gray-300 rounded-s-lg hover:bg-gray-100 hover:text-gray-700 dark:bg-gray-800 dark:border-gray-700 dark:text-gray-400 dark:hover:bg-gray-700 dark:hover:text-white">Previous</a> </li> <!-- Number Buttons --> <li v-for="page in pages" :key="page"> <a href="#" @click.prevent="changePage(page)" :class="`flex items-center justify-center px-3 h-8 leading-tight dark:bg-gray-800 dark:border-gray-700 dark:text-gray-400 dark:hover:bg-gray-700 dark:hover:text-white border border-gray-300 ${page === currentPage ? 'text-blue-600 bg-blue-50 hover:bg-blue-100 hover:text-blue-700':'text-gray-500 bg-white hover:bg-gray-100 hover:text-gray-700'}`" >{{ page }}</a> </li> <!-- Next Button --> <li> <a href="#" @click.prevent="changePage(currentPage + 1)" :class="{ 'cursor-not-allowed opacity-50': currentPage === totalPages }" class="flex items-center justify-center px-3 h-8 leading-tight text-gray-500 bg-white border border-gray-300 rounded-e-lg hover:bg-gray-100 hover:text-gray-700 dark:bg-gray-800 dark:border-gray-700 dark:text-gray-400 dark:hover:bg-gray-700 dark:hover:text-white">Next</a> </li> </ul> </nav> </template>
Components/Modal-Generic.vue
<script setup> import { onMounted, onUnmounted } from 'vue'; import { Modal } from 'flowbite'; let modal; defineProps({ title: { type: String, required: false }, hideHeader: { type: Boolean, required: false, default: false } }); const emits = defineEmits(["close"]); const closeModal = () => { emits("close"); modal.hide(); } onMounted(() => { const $modalElement = document.querySelector('#generic-modal', { closable: true, backdrop: 'dynamic', backdropClasses: 'modal-backdrop bg-gray-900/50 dark:bg-gray-900/80 fixed inset-0 z-40' }); if ($modalElement) { modal = new Modal($modalElement); modal.show(); } }) onUnmounted(() => { modal.hide(); }); </script> <template> <div id="generic-modal" tabindex="-1" class="fixed top-0 left-0 right-0 z-50 hidden w-full p-4 overflow-x-hidden overflow-y-auto md:inset-0 h-[calc(100%-1rem)] max-h-full"> <div class="relative w-full max-w-lg max-h-full"> <!-- Modal content --> <div class="relative bg-white rounded-lg shadow-sm dark:bg-gray-700"> <!-- Modal header --> <div v-if="!hideHeader" class="flex items-center justify-between p-4 md:p-5 border-b rounded-t dark:border-gray-600 border-gray-200"> <h3 class="text-lg font-semibold text-gray-900 dark:text-white" v-if="title"> {{ title }} </h3> <button type="button" @click="closeModal" class="text-gray-400 bg-transparent hover:bg-gray-200 hover:text-gray-900 rounded-lg text-sm w-8 h-8 ms-auto inline-flex justify-center items-center dark:hover:bg-gray-600 dark:hover:text-white"> <svg class="w-3 h-3" xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 14 14"> <path stroke="currentColor" stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="m1 1 6 6m0 0 6 6M7 7l6-6M7 7l-6 6"/> </svg> <span class="sr-only">Close modal</span> </button> </div> <!-- Modal body --> <slot /> </div> </div> </div> </template>
Components/ConfirmDialog.vue
<script setup> import ModalGeneric from './Modal-Generic.vue'; defineProps({ confirmText: { type: String } }); defineEmits(['close', 'confirm']); </script> <template> <ModalGeneric :hideHeader="true" @close="$emit('close')"> <div class="p-4 md:p-5 text-center"> <svg class="mx-auto mb-4 text-gray-400 w-12 h-12 dark:text-gray-200" aria-hidden="true" xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 20 20"> <path stroke="currentColor" stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M10 11V6m0 8h.01M19 10a9 9 0 1 1-18 0 9 9 0 0 1 18 0Z"/> </svg> <h3 class="mb-5 text-lg font-normal text-gray-500 dark:text-gray-400"> {{confirmText}} </h3> <button type="button" @click="$emit('confirm')" class="text-white bg-red-600 hover:bg-red-800 focus:ring-4 focus:outline-none focus:ring-red-300 dark:focus:ring-red-800 font-medium rounded-lg text-sm inline-flex items-center px-5 py-2.5 text-center"> Yes </button> <button type="button" @click="$emit('close')" class="py-2.5 px-5 ms-3 text-sm font-medium text-gray-900 focus:outline-none bg-white rounded-lg border border-gray-200 hover:bg-gray-100 hover:text-blue-700 focus:z-10 focus:ring-4 focus:ring-gray-100 dark:focus:ring-gray-700 dark:bg-gray-800 dark:text-gray-400 dark:border-gray-600 dark:hover:text-white dark:hover:bg-gray-700">No, cancel</button> </div> </ModalGeneric> </template>
The <FlashMessage />
helper component used to display laravel flash messages. Messages can be accessed using $page.props.flash
key as described above in the sharing data. And using tailwindcss to apply some css classes to each flash message.
The <InputError />
component displays validation errors under each html input. It takes errors and field as props and render a simple div with red color.
The <Pagination />
component display the pagination links according to laravel paginator. You can use this component in any of your vue apps and customize it as needed. We pass props currentPage
, totalPages
and maxVisibleButtons
. The component emit `page-changed
` event when clicking on each link.
The <Modal-Generic />
component display a flowbite modal and can be used in different vue pages if you need to display a modal. Using <slot />
as the modal body so we can pass any arbiritary html code. In the onMounted()
hook we initialize the modal using flowbite Modal class passing in the modal selector and display the modal with modal.show()
In the onUnmounted()
hook we hide the modal by calling modal.hide()
.
The <ConfirmDialog/>
component displays a nice confirmation message which can be used in cases like deleting entities. The component makes use of the <Modal-Generic />
component and takes a props confirmText
and emits the close
and confirm
custom events.