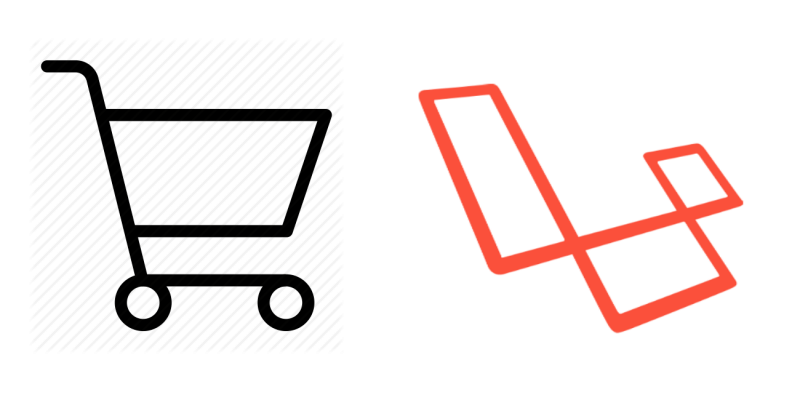
In this tutorial i will demonstrate how to create a shopping cart with php using laravel framework. we will be using laravel 5.5 but you can also use any other version.
Requirements:
- Apache or IIS with PHP >= 5.6.4 .
- Twitter bootstrap.
Â
Installation
In your local development path type this command to install laravel 5.5.
composer create-project laravel/laravel shopping-cart "5.5.*" --prefer-dist
After successful installation be sure that there is a “.env” file in the root path of the application. If not then take a copy of “.env.example” and rename it to “.env” then in type this command in the terminal.
php artisan key:generate
Now laravel successfully installed and you can be sure by visiting http://localhost/shopping-cart/public/.
Preparing Database
Every shopping cart must have a products table so we will create it right now.
Go to phpmyadmin and create a new database called “shopping_cart”. Then go to your .env file and set your database name and credentials like this:
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=shopping_cart DB_USERNAME=<your db username> DB_PASSWORD=<your db password>
Next we will be using laravel migration to create tables so ype this command in terminal.
php artisan make:model Product -m
The above command will create a Product model and -m for migration.
Now go to database>migrations and open up the migration we just created for products table.
<?php use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class CreateProductsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('products', function (Blueprint $table) { $table->increments('id'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('products'); } }
We will be adding some more fields to the up() function so add this code after $table->increments(‘id’)
$table->string("name", 255)->nullable(); $table->text("description")->nullable(); $table->string("photo", 255)->nullable(); $table->decimal("price", 6, 2);
Now migrate your tables by this command:
php artisan migrate
Now if you go to phpmyadmin. This is our database might look like after migration
Now let’s create a Seeder to populate our products table with some data to work with. Run this command to generate a seeder.
php artisan make:seed ProductsSeeder
Go to database>seeds>ProductsSeeder. Then inside run() function add this code.
DB::table('products')->insert([ 'name' => 'Samsung Galaxy S9', 'description' => 'A brand new, sealed Lilac Purple Verizon Global Unlocked Galaxy S9 by Samsung. This is an upgrade. Clean ESN and activation ready.', 'photo' => 'https://i.ebayimg.com/00/s/ODY0WDgwMA==/z/9S4AAOSwMZRanqb7/$_35.JPG?set_id=89040003C1', 'price' => 698.88 ]); DB::table('products')->insert([ 'name' => 'Apple iPhone X', 'description' => 'GSM & CDMA FACTORY UNLOCKED! WORKS WORLDWIDE! FACTORY UNLOCKED. iPhone x 64gb. iPhone 8 64gb. iPhone 8 64gb. iPhone X with iOS 11.', 'photo' => 'https://i.ebayimg.com/00/s/MTYwMFg5OTU=/z/9UAAAOSwFyhaFXZJ/$_35.JPG?set_id=89040003C1', 'price' => 983.00 ]); DB::table('products')->insert([ 'name' => 'Google Pixel 2 XL', 'description' => 'New condition • No returns, but backed by eBay Money back guarantee', 'photo' => 'https://i.ebayimg.com/00/s/MTYwMFg4MzA=/z/G2YAAOSwUJlZ4yQd/$_35.JPG?set_id=89040003C1', 'price' => 675.00 ]); DB::table('products')->insert([ 'name' => 'LG V10 H900', 'description' => 'NETWORK Technology GSM. Protection Corning Gorilla Glass 4. MISC Colors Space Black, Luxe White, Modern Beige, Ocean Blue, Opal Blue. SAR EU 0.59 W/kg (head).', 'photo' => 'https://i.ebayimg.com/00/s/NjQxWDQyNA==/z/VDoAAOSwgk1XF2oo/$_35.JPG?set_id=89040003C1', 'price' => 159.99 ]); DB::table('products')->insert([ 'name' => 'Huawei Elate', 'description' => 'Cricket Wireless - Huawei Elate. New Sealed Huawei Elate Smartphone.', 'photo' => 'https://ssli.ebayimg.com/images/g/aJ0AAOSw7zlaldY2/s-l640.jpg', 'price' => 68.00 ]); DB::table('products')->insert([ 'name' => 'HTC One M10', 'description' => 'The device is in good cosmetic condition and will show minor scratches and/or scuff marks.', 'photo' => 'https://i.ebayimg.com/images/g/u-kAAOSw9p9aXNyf/s-l500.jpg', 'price' => 129.99 ]);
Now run this command to seed your data.
php artisan db:seed --class=ProductsSeeder
Our products table now might look like.
Controller & Views
Let’s create a controller for our products and cart called “ProductsController”.
php artisan make:controller ProductsController
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Product; class ProductsController extends Controller { }
Now these actions in the above controller as shown:
public function index() { return view('products'); } public function cart() { return view('cart'); } public function addToCart($id) { }
In the above code we created two actions for our products and cart pages and one action for add to cart.
Next we will create three views with dummy data:
- layout.blade.php: this is the main layout view.
- products.blade.php: this is the view that will display our products.
- cart.blade.php: this is the cart view.
In the layout.blade.php copy and paste this code.
<!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>@yield('title')</title> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css"> <link rel="stylesheet" type="text/css" href="{{ asset('css/style.css') }}"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"></script> </head> <body> <div class="container"> <div class="row"> <div class="col-lg-12 col-sm-12 col-12 main-section"> <div class="dropdown"> <button type="button" class="btn btn-info" data-toggle="dropdown"> <i class="fa fa-shopping-cart" aria-hidden="true"></i> Cart <span class="badge badge-pill badge-danger">3</span> </button> <div class="dropdown-menu"> <div class="row total-header-section"> <div class="col-lg-6 col-sm-6 col-6"> <i class="fa fa-shopping-cart" aria-hidden="true"></i> <span class="badge badge-pill badge-danger">3</span> </div> <div class="col-lg-6 col-sm-6 col-6 total-section text-right"> <p>Total: <span class="text-info">$2,978.24</span></p> </div> </div> <div class="row cart-detail"> <div class="col-lg-4 col-sm-4 col-4 cart-detail-img"> <img src="https://images-na.ssl-images-amazon.com/images/I/811OyrCd5hL._SX425_.jpg"> </div> <div class="col-lg-8 col-sm-8 col-8 cart-detail-product"> <p>Sony DSC-RX100M..</p> <span class="price text-info"> $250.22</span> <span class="count"> Quantity:01</span> </div> </div> <div class="row cart-detail"> <div class="col-lg-4 col-sm-4 col-4 cart-detail-img"> <img src="https://cdn2.gsmarena.com/vv/pics/blu/blu-vivo-48-1.jpg"> </div> <div class="col-lg-8 col-sm-8 col-8 cart-detail-product"> <p>Vivo DSC-RX100M..</p> <span class="price text-info"> $500.40</span> <span class="count"> Quantity:01</span> </div> </div> <div class="row cart-detail"> <div class="col-lg-4 col-sm-4 col-4 cart-detail-img"> <img src="https://static.toiimg.com/thumb/msid-55980052,width-640,resizemode-4/55980052.jpg"> </div> <div class="col-lg-8 col-sm-8 col-8 cart-detail-product"> <p>Lenovo DSC-RX100M..</p> <span class="price text-info"> $445.78</span> <span class="count"> Quantity:01</span> </div> </div> <div class="row"> <div class="col-lg-12 col-sm-12 col-12 text-center checkout"> <a href="{{ url('cart') }}" class="btn btn-primary btn-block">View all</a> </div> </div> </div> </div> </div> </div> </div> <div class="container page"> @yield('content') </div> @yield('scripts') </body> </html>
As shown in the above code we have included bootstrap and jquery and the main style.css file and in the template header we displayed the cart icon along with sample dummy data.
products.blade.php
@extends('layout') @section('title', 'Products') @section('content') <div class="container products"> <div class="row"> <div class="col-xs-18 col-sm-6 col-md-3"> <div class="thumbnail"> <img src="http://placehold.it/500x300" alt=""> <div class="caption"> <h4>Product name</h4> <p>Lorem ipsum dolor sit amet, consectetur adipisicing elit. Facere, soluta, eligendi doloribus sunt minus amet sit debitis repellat. Consectetur, culpa itaque odio similique suscipit</p> <p><strong>Price: </strong> 567.7$</p> <p class="btn-holder"><a href="#" class="btn btn-warning btn-block text-center" role="button">Add to cart</a> </p> </div> </div> </div> <div class="col-xs-18 col-sm-6 col-md-3"> <div class="thumbnail"> <img src="http://placehold.it/500x300" alt=""> <div class="caption"> <h4>Product name</h4> <p>Lorem ipsum dolor sit amet, consectetur adipisicing elit. Facere, soluta, eligendi doloribus sunt minus amet sit debitis repellat. Consectetur, culpa itaque odio similique suscipit</p> <p><strong>Price: </strong> 567.7$</p> <p class="btn-holder"><a href="#" class="btn btn-warning btn-block text-center" role="button">Add to cart</a> </p> </div> </div> </div> <div class="col-xs-18 col-sm-6 col-md-3"> <div class="thumbnail"> <img src="http://placehold.it/500x300" alt=""> <div class="caption"> <h4>Product name</h4> <p>Lorem ipsum dolor sit amet, consectetur adipisicing elit. Facere, soluta, eligendi doloribus sunt minus amet sit debitis repellat. Consectetur, culpa itaque odio similique suscipit</p> <p><strong>Price: </strong> 567.7$</p> <p class="btn-holder"><a href="#" class="btn btn-warning btn-block text-center" role="button">Add to cart</a> </p> </div> </div> </div> <div class="col-xs-18 col-sm-6 col-md-3"> <div class="thumbnail"> <img src="http://placehold.it/500x300" alt=""> <div class="caption"> <h4>Product name</h4> <p>Lorem ipsum dolor sit amet, consectetur adipisicing elit. Facere, soluta, eligendi doloribus sunt minus amet sit debitis repellat. Consectetur, culpa itaque odio similique suscipit</p> <p><strong>Price: </strong> 567.7$</p> <p class="btn-holder"><a href="#" class="btn btn-warning btn-block text-center" role="button">Add to cart</a> </p> </div> </div> </div> </div><!-- End row --> </div> @endsection
cart.blade.php
@extends('layout') @section('title', 'Cart') @section('content') <table id="cart" class="table table-hover table-condensed"> <thead> <tr> <th style="width:50%">Product</th> <th style="width:10%">Price</th> <th style="width:8%">Quantity</th> <th style="width:22%" class="text-center">Subtotal</th> <th style="width:10%"></th> </tr> </thead> <tbody> <tr> <td data-th="Product"> <div class="row"> <div class="col-sm-3 hidden-xs"><img src="http://placehold.it/100x100" alt="..." class="img-responsive"/></div> <div class="col-sm-9"> <h4 class="nomargin">Product 1</h4> <p>Quis aute iure reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Lorem ipsum dolor sit amet.</p> </div> </div> </td> <td data-th="Price">$1.99</td> <td data-th="Quantity"> <input type="number" class="form-control text-center" value="1"> </td> <td data-th="Subtotal" class="text-center">1.99</td> <td class="actions" data-th=""> <button class="btn btn-info btn-sm"><i class="fa fa-refresh"></i></button> <button class="btn btn-danger btn-sm"><i class="fa fa-trash-o"></i></button> </td> </tr> </tbody> <tfoot> <tr class="visible-xs"> <td class="text-center"><strong>Total 1.99</strong></td> </tr> <tr> <td><a href="{{ url('/') }}" class="btn btn-warning"><i class="fa fa-angle-left"></i> Continue Shopping</a></td> <td colspan="2" class="hidden-xs"></td> <td class="hidden-xs text-center"><strong>Total $1.99</strong></td> </tr> </tfoot> </table> @endsection
style.css
.main-section{ background-color: #F8F8F8; } .dropdown{ float:right; padding-right: 30px; } .btn{ border:0px; margin:10px 0px; box-shadow:none !important; } .dropdown .dropdown-menu{ padding:20px; top:30px !important; width:350px !important; left:-110px !important; box-shadow:0px 5px 30px black; } .total-header-section{ border-bottom:1px solid #d2d2d2; } .total-section p{ margin-bottom:20px; } .cart-detail{ padding:15px 0px; } .cart-detail-img img{ width:100%; height:100%; padding-left:15px; } .cart-detail-product p{ margin:0px; color:#000; font-weight:500; } .cart-detail .price{ font-size:12px; margin-right:10px; font-weight:500; } .cart-detail .count{ color:#C2C2DC; } .checkout{ border-top:1px solid #d2d2d2; padding-top: 15px; } .checkout .btn-primary{ border-radius:50px; height:50px; } .dropdown-menu:before{ content: " "; position:absolute; top:-20px; right:50px; border:10px solid transparent; border-bottom-color:#fff; } .thumbnail { position: relative; padding: 0px; margin-bottom: 20px; } .thumbnail img { width: 100%; } .thumbnail .caption{ margin: 7px; } .page { margin-top: 50px; } .btn-holder{ text-align: center; } .table>tbody>tr>td, .table>tfoot>tr>td{ vertical-align: middle; } @media screen and (max-width: 600px) { table#cart tbody td .form-control{ width:20%; display: inline !important; } .actions .btn{ width:36%; margin:1.5em 0; } .actions .btn-info{ float:left; } .actions .btn-danger{ float:right; } table#cart thead { display: none; } table#cart tbody td { display: block; padding: .6rem; min-width:320px;} table#cart tbody tr td:first-child { background: #333; color: #fff; } table#cart tbody td:before { content: attr(data-th); font-weight: bold; display: inline-block; width: 8rem; } table#cart tfoot td{display:block; } table#cart tfoot td .btn{display:block;} }
Routes
At this point go to routes>web.php and add the following routes:
<?php /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::get('/', 'ProductsController@index'); Route::get('cart', 'ProductsController@cart'); Route::get('add-to-cart/{id}', 'ProductsController@addToCart');
Now visit http://localhost/shopping-cart/public/ and http://localhost/shopping-cart/public/cart you will see our products and cart page respectively.
Displaying Products
Go to ProductsController.php in the index function add the below code
public function index() { $products = Product::all(); return view('products', compact('products')); }
Here we fetch all products and sent them to the products view.
Now go to resources>views>products.blade.php and replace the dummy data with the below code
@extends('layout') @section('title', 'Products') @section('content') <div class="container products"> <div class="row"> @foreach($products as $product) <div class="col-xs-18 col-sm-6 col-md-3"> <div class="thumbnail"> <img src="{{ $product->photo }}" width="500" height="300"> <div class="caption"> <h4>{{ $product->name }}</h4> <p>{{ str_limit(strtolower($product->description), 50) }}</p> <p><strong>Price: </strong> {{ $product->price }}$</p> <p class="btn-holder"><a href="{{ url('add-to-cart/'.$product->id) }}" class="btn btn-warning btn-block text-center" role="button">Add to cart</a> </p> </div> </div> </div> @endforeach </div><!-- End row --> </div> @endsection
Great work now you can see the actual products by going to http://localhost/shopping-cart/public/
Processing the cart
Now its time to add logic for when user clicks on add to cart button. We will do this in the addToCart function in ProductsController.php and we will store the cart item using laravel session.
public function addToCart($id) { $product = Product::find($id); if(!$product) { abort(404); } $cart = session()->get('cart'); // if cart is empty then this the first product if(!$cart) { $cart = [ $id => [ "name" => $product->name, "quantity" => 1, "price" => $product->price, "photo" => $product->photo ] ]; session()->put('cart', $cart); return redirect()->back()->with('success', 'Product added to cart successfully!'); } // if cart not empty then check if this product exist then increment quantity if(isset($cart[$id])) { $cart[$id]['quantity']++; session()->put('cart', $cart); return redirect()->back()->with('success', 'Product added to cart successfully!'); } // if item not exist in cart then add to cart with quantity = 1 $cart[$id] = [ "name" => $product->name, "quantity" => 1, "price" => $product->price, "photo" => $product->photo ]; session()->put('cart', $cart); return redirect()->back()->with('success', 'Product added to cart successfully!'); }
In the above code first we fetch the product and checked if it exist or not then we check if there is a cart exist in the session. If cart is empty then we treated the item as the first product and insert it along with quantity and price. If cart not empty we checked if the product already exist and increment its quantity.
Displaying the cart contents
At this point we have a cart with products let’s display it in the cart view.
Go to resources>views>cart.blade.php and update the view with below code:
@extends('layout') @section('title', 'Cart') @section('content') <table id="cart" class="table table-hover table-condensed"> <thead> <tr> <th style="width:50%">Product</th> <th style="width:10%">Price</th> <th style="width:8%">Quantity</th> <th style="width:22%" class="text-center">Subtotal</th> <th style="width:10%"></th> </tr> </thead> <tbody> <?php $total = 0 ?> @if(session('cart')) @foreach(session('cart') as $id => $details) <?php $total += $details['price'] * $details['quantity'] ?> <tr> <td data-th="Product"> <div class="row"> <div class="col-sm-3 hidden-xs"><img src="{{ $details['photo'] }}" width="100" height="100" class="img-responsive"/></div> <div class="col-sm-9"> <h4 class="nomargin">{{ $details['name'] }}</h4> </div> </div> </td> <td data-th="Price">${{ $details['price'] }}</td> <td data-th="Quantity"> <input type="number" value="{{ $details['quantity'] }}" class="form-control quantity" /> </td> <td data-th="Subtotal" class="text-center">${{ $details['price'] * $details['quantity'] }}</td> <td class="actions" data-th=""> <button class="btn btn-info btn-sm update-cart" data-id="{{ $id }}"><i class="fa fa-refresh"></i></button> <button class="btn btn-danger btn-sm remove-from-cart" data-id="{{ $id }}"><i class="fa fa-trash-o"></i></button> </td> </tr> @endforeach @endif </tbody> <tfoot> <tr class="visible-xs"> <td class="text-center"><strong>Total {{ $total }}</strong></td> </tr> <tr> <td><a href="{{ url('/') }}" class="btn btn-warning"><i class="fa fa-angle-left"></i> Continue Shopping</a></td> <td colspan="2" class="hidden-xs"></td> <td class="hidden-xs text-center"><strong>Total ${{ $total }}</strong></td> </tr> </tfoot> </table> @endsection
And also update the main layout view layout.blade.php
<!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>@yield('title')</title> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css"> <link rel="stylesheet" type="text/css" href="{{ asset('css/style.css') }}"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"></script> </head> <body> <div class="container"> <div class="row"> <div class="col-lg-12 col-sm-12 col-12 main-section"> <div class="dropdown"> <button type="button" class="btn btn-info" data-toggle="dropdown"> <i class="fa fa-shopping-cart" aria-hidden="true"></i> Cart <span class="badge badge-pill badge-danger">{{ count((array) session('cart')) }}</span> </button> <div class="dropdown-menu"> <div class="row total-header-section"> <div class="col-lg-6 col-sm-6 col-6"> <i class="fa fa-shopping-cart" aria-hidden="true"></i> <span class="badge badge-pill badge-danger">{{ count((array) session('cart')) }}</span> </div> <?php $total = 0 ?> @foreach((array) session('cart') as $id => $details) <?php $total += $details['price'] * $details['quantity'] ?> @endforeach <div class="col-lg-6 col-sm-6 col-6 total-section text-right"> <p>Total: <span class="text-info">$ {{ $total }}</span></p> </div> </div> @if(session('cart')) @foreach(session('cart') as $id => $details) <div class="row cart-detail"> <div class="col-lg-4 col-sm-4 col-4 cart-detail-img"> <img src="{{ $details['photo'] }}" /> </div> <div class="col-lg-8 col-sm-8 col-8 cart-detail-product"> <p>{{ $details['name'] }}</p> <span class="price text-info"> ${{ $details['price'] }}</span> <span class="count"> Quantity:{{ $details['quantity'] }}</span> </div> </div> @endforeach @endif <div class="row"> <div class="col-lg-12 col-sm-12 col-12 text-center checkout"> <a href="{{ url('cart') }}" class="btn btn-primary btn-block">View all</a> </div> </div> </div> </div> </div> </div> </div> <div class="container page"> @yield('content') </div> @yield('scripts') </body> </html>
Updating & removing from the cart
Now to remove or update an item in the cart we will create two actions in the ProductsController as follows:
public function update(Request $request) { if($request->id and $request->quantity) { $cart = session()->get('cart'); $cart[$request->id]["quantity"] = $request->quantity; session()->put('cart', $cart); session()->flash('success', 'Cart updated successfully'); } } public function remove(Request $request) { if($request->id) { $cart = session()->get('cart'); if(isset($cart[$request->id])) { unset($cart[$request->id]); session()->put('cart', $cart); } session()->flash('success', 'Product removed successfully'); } }
Let’s create two other routes
Route::patch('update-cart', 'ProductsController@update'); Route::delete('remove-from-cart', 'ProductsController@remove');
Now go to resources>views>cart.blade.php and add this code at the bottom
@section('scripts') <script type="text/javascript"> $(".update-cart").click(function (e) { e.preventDefault(); var ele = $(this); $.ajax({ url: '{{ url('update-cart') }}', method: "patch", data: {_token: '{{ csrf_token() }}', id: ele.attr("data-id"), quantity: ele.parents("tr").find(".quantity").val()}, success: function (response) { window.location.reload(); } }); }); $(".remove-from-cart").click(function (e) { e.preventDefault(); var ele = $(this); if(confirm("Are you sure")) { $.ajax({ url: '{{ url('remove-from-cart') }}', method: "DELETE", data: {_token: '{{ csrf_token() }}', id: ele.attr("data-id")}, success: function (response) { window.location.reload(); } }); } }); </script> @endsection
As shown above we added two listeners for buttons update and remove and for each button just send ajax request to update and remove products.
And this is an updated version of the tutorial that uses ajax
https://bitbucket.org/webmobtuts/shopping-cart-tutorial-laravel/src/master/
Conclusion
You learned in this tutorial how to build a shopping cart with laravel. I decided to built this from scratch although there are ready made packages in laravel for shopping carts but to let you understand the basics of shopping cart. You can also extend this tutorial to add functionality of checkout, payments, and more
Obtive error: count(): Parameter must be an array or an object that implements Countable.
Código blade: {{ count(session(‘cart’)) }}
This error usually happens in php 7.2 make sure that you use a stable version of php 7
i got this error i’m using xampp on windows
is there any solution to solve this?
Open resources/views/layout.blade.php and modify those lines
line 26
Cart {{ session(‘cart’)!=null?count(session(‘cart’)):0 }}
line 31
{{ session(‘cart’)!=null?count(session(‘cart’)):0 }}
line 35 replace the first foreach with
@if(session(‘cart’) != null)
@foreach(session(‘cart’) as $id => $details)
@endforeach
@endif
line 36 replace the foreach with
@if(session(‘cart’) != null)
@foreach(session(‘cart’) as $id => $details)
{{ $details[‘name’] }}
${{ $details[‘price’] }} Quantity:{{ $details[‘quantity’] }}
@endforeach
@endif
how to implement in 7.3 php version
i think it will work in php 7.3 without problems
Thanks for the great tutorial, replace your layout.blade.php code with the following and it will fix your problem.
—————————————————————-
Change
{{ count(session(‘cart’)) }}
to
@if(session(‘cart’)!==null)
{{ count(session(‘cart’)) }}
@endif
Change
@foreach(session(‘cart’) as $id => $details)
@endforeach
to
@if(session(‘cart’)!==null)
@foreach(session(‘cart’) as $id => $details)
@endforeach
@endif
Funciona yo lo probe en la version de Php 7.3.12 con laravel 7. Las dos Versiones de Código de arriba. Saludos. Gracias…
Thanks
Open resources/views/layout.blade.php and modify those lines
line 26
Cart {{ session(‘cart’)!=null?count(session(‘cart’)):0 }}
line 31
{{ session(‘cart’)!=null?count(session(‘cart’)):0 }}
line 35 replace the first foreach with
@if(session(‘cart’) != null)
@foreach(session(‘cart’) as $id => $details)
@endforeach
@endif
line 36 replace the foreach with
@if(session(‘cart’) != null)
@foreach(session(‘cart’) as $id => $details)
{{ $details[‘name’] }}
${{ $details[‘price’] }} Quantity:{{ $details[‘quantity’] }}
@endforeach
@endif
i got it fixed, just add (array) before the session
{{ count(session(‘cart’)) }}
to
{{ count((array)session(‘cart’)) }}
No use same error was displaying. like as below:
count(): Parameter must be an array or an object that implements Countable
Try this fix add
(array) before session(‘cart’)
hola me sale este error al momento de usar el comando “php artisan migrate”
In Parser.php line 120:
dotenv values containing spaces must be surrounded by quotes.
show me the contents of .env file
Hola, como puedo hacer que la primera pagina sea el welcome, luego de registrarse, iniciar sesion, aparezcan los productos ??? Gracias
In files app/Http/Controllers/Auth/LoginController.php and app/Http/Controllers/Auth/RegisterController.php
change
protected $redirectTo = ‘/home’;
to
protected $redirectTo = ‘/’;
hello ,I got Invalid argument supplied for foreach() ,any solution ?
Open resources/views/layout.blade.php and modify those lines
line 26
Cart {{ session(‘cart’)!=null?count(session(‘cart’)):0 }}
line 31
{{ session(‘cart’)!=null?count(session(‘cart’)):0 }}
line 35 replace the first foreach with
@if(session(‘cart’) != null)
@foreach(session(‘cart’) as $id => $details)
@endforeach
@endif
line 36 replace the foreach with
@if(session(‘cart’) != null)
@foreach(session(‘cart’) as $id => $details)
{{ $details[‘name’] }}
${{ $details[‘price’] }} Quantity:{{ $details[‘quantity’] }}
@endforeach
@endif
Hi brother I fixed session cart like you but error foreach. Could you help please
can you put the line number where the error happens
Great tutorial! Thank you.
You welcome
Parse error: syntax error, unexpected ‘cart’ (T_STRING), expecting ‘,’ or ‘)’ (View: C:\xampp\htdocs\shopping-cart-demo\resources\views\layout.blade.php) (View: C:\xampp\htdocs\shopping-cart-demo\resources\views\layout.blade.php)
show me the code
Dear all I find the solution it is good in view/layout.blade.php in foreach you sould pass is code is working good
@if (is_array(session(‘cart’)))
@foreach(session(‘cart’) as $id => $details)
@endforeach
@endif
This can’t delete cart or update , it’s just saying “are you sure” then nothing changes why?
can you inspect the browser console?
Yes , same issue with me
Getting 500 error in console
Do you followed the full tutorial? because i didn’t see error 500 in the console
you just wanna include script JQUERY in layout.blade.php in
thanks yes of course i am kinda missing it
violation access error 767 bytes in windows
Syntax error or access violation: 1071 Specified key was too long; max key length is 767 bytes
This error usually happens in laravel related to mysql field length
the solution in this article
https://laravel-news.com/laravel-5-4-key-too-long-error
Class ‘App\Http\Controllers\Product’ not found
make sure to import Product model
in the top of the ProductsController
add
use App\Product;
$products = Product::all(); in ProductsController
Class ‘App\Http\Controllers\Product’ not found
Hay dude… Sorry, can you help me?
My @yield(‘script’) from cart.blade.php can’t work after remove-from-cart and update-cart onclick in button.
My cart can’t update quantity and can’t remove product from cart. Please help me…
Thankyou dude…
Ensure that you follow every step in the tutorial
Thanks alot. I appreciate every bit of ur code. More grace
You welcome
I would rather use existing package and app that is out there. I have use this one for one of my client.
https://github.com/avored/laravel-ecommerce
Yes of course i am just explaining the idea of shopping cart because people usually looking to build things from scratch
am getting the error
A non-numeric value encountered (View: C:\xampp\htdocs\Tafuta_restaurant\resources\views\restaurants\cart.blade.php)
Try this fix
@if (is_array(session(‘cart’))) @foreach(session(‘cart’) as $id => $details) @endforeach @endif
Hi, first of all thanks, your tutorial helped me a lot but I have a problem, I do not understand how the variable “quantity” works since it is not defined in the database and my cart is not storing it.
Could you explain to me how the variable “quantity” works and how do you use it?
Thank you.
First the quantity represent amount of specific product into the cart. When you add the products into the cart for the first time this quantity is 1 which means you have 1 quantity of each product in the cart but you can update this quantity from the cart page for example you have a cart which have two different products which are product1 and product2 and the quantity initially is 1. Next i wanted to increase the product1 amount to 3 and the product2 amount to 2. Then the final cart quantity = product1 * 3 + product2 * 2… Read more »
thanks for answerme. I’m doing a chatbot, customers write the quantity of products they require. I capture in a variable “$quantity” what the customer writes (number): foreach ($product as $key => $value) { $cart = [ $p => [ “code” => $value->code, “quantity” => $quantity, “price” => $value->price, “archImg” => $value->archImg ] ]; session()->put(‘cart’, $cart); $this->say(‘success 1’, ‘Product added to cart successfully!’); } } Then, when I want to add more products of the same type and increase the quantity, I don’t allow it: if(isset($cart[$p])) { $cart[$p][‘quantity’] + $quantity; session()->put(‘cart’, $cart); $this->say(‘success 2, Product added to cart successfully!’); } and… Read more »
Is this line correct?
$cart[$p][‘quantity’] + $quantity;
i think it should be
$cart[$p][‘quantity’] += $quantity;
besides you can debug $cart[$p] to check if the quantity has valid value
like var_dump($cart[$p]);
Thanks . That is great. however when I press add to cart the page was refreshing mean reload How can I make it without refreshing
You have to modify the code to use Ajax
how to make that
Didn’t you know how to use Jquery ajax?
https://api.jquery.com/jQuery.ajax
may I give the code then you modify it?
It may take some time from me as i have a lot of work to do right now
this a hearf **/ id) }}”>Add to Cart
/**
the rout and controller have done
you already make code ajax in cart page at remove and update button but you write success function as reload function that refresh whole page when you click are there others code or idea
I am just showing the process of manipulating the cart in regard to using ajax or php directly
$(“.remove-from-cart”).click(function (e) {
e.preventDefault();
var ele = $(this);
if(confirm(“Are you sure”)) {
$.ajax({
url: ‘{{ url(‘admin/remove-from-cart’) }}’,
method: “DELETE”,
data: {_token: ‘{{ csrf_token() }}’, id: ele.attr(“data-id”)},
success: function (response) {
window.location.reload();
}
});
}
I will modify it and i will reply to you as soon as possible
This is updated version of the tutorial that uses ajax
git clone https://webmobtuts@bitbucket.org/webmobtuts/shopping-cart-tutorial-laravel.git
How can I express my thank for you there is no way ….. big thanks for you and the god save you
Don’t mention it 😀
Thank you so much for this tutorial. After adding to cart the product photo does not display, it also does not display on view cart, what do i do please?
You mean when you click add to cart the cart still empty?
Sometimes you need to change the session cookie name in laravel go to .env and add this
SESSION_COOKIE=any name
No, it’s all working good. but it only displays product name, price, quantity, total but does not display product photo.
Am using image to call my product image instead of photo, so when i tried changing photo to image both in the blade and controller, i get the error “Undefined index: image”, but when i use photo i get no errors but photo does not display.
Be sure that you set the photo path correctly
Great tutorial! I am having trouble getting the cart totals (to enter into my database). How do I go about it?
What is the error?
how can i save each of my cart orders to a table in DB which can be viewed later by the user.
I am using the ajax version here
So instead of saving the cart into session you can make a simple table which contains those columns:
– id
– product_id
– quantity
public function storeCart(Request $request){
Cart::create($request->all());
return redirect()->route(‘/’);
}
– I created a function as above. When the ‘storeCart’ is triggered, product_id appears as NULL and the quantity is always 0.00 in the database
debug to see what there is in $request->all() i.e dd($request->all);
class Product extends Model
{
protected $fillable = [ ‘name’, ‘description’, ‘price’];
/**
* Get the post that owns the comment.
*/
public function carts()
{
return $this->hasMany(‘Cart’);
}
}
-This is my Product Model
class Cart extends Model
{
protected $fillable = [
‘quantity’,
];
/**
* Get the comments for the blog post.
*/
public function products(){
return $this->hasMany(‘App\Product’,’product_id’);
}
}
-This is my Cart Model
Try this:
class Cart extends Model
{
protected $fillable = [
'product_id',
'user_id',
'quantity'
];
public function product(){
return $this->belongsTo(‘App\Product’,’product_id’);
}
}
I can’t delete the first row of the cart
why!!!
I don’t know. I need help on that. When i add other items to the cart, i can delete them apart from the first item added. And also if i add an item twice, it does not increase it’s quantity, it only adds it a second time.
what’s the error? inspect the console for javascript errors
hi,when I change the names and data I get this error: Undefined index: image
I changed it to this:
$cart = [
$id => [
“descripcion” => $product->DESCRIPCION,
“cantidad” => 1,
“precio” => $product->PRECIO_COSTE,
“imagen” => $product->IMAGEN
]
];
and in the view just change the names to how I put them in the controller
you store it as “imagen” not “image” so you have to access it as “imagen”
unable to refresh or delete item from cart
Use of undefined constant product – assumed ‘product’ (this will throw an Error in a future version of PHP)
i have this error for my addToCart function, please help me
be sure to write $product not product
When I trigger add-to-cart button it shows url with product id but displays
Sorry, the page you are looking for could not be found.
clone it from the repo
https://bitbucket.org/webmobtuts/shopping-cart-tutorial-laravel/src/master/
thanks for this code
👍
No sirven las funciones de actualizar o remover salu2.
great !!!!!! your code help me thank.
May God bless you
Thanks
I had a problem when updating the product quantity with multiple product in cart. When I update the first product it has no problem, but when I update the other product, the product quantity update with the same number as the first product.
Strange! I tested it and works fine
same here.. only the first product will update.
How to resolve this
ErrorException (E_ERROR)
Undefined variable: products (View: C:\Users\DSA\shopping-cart\resources\views\products.blade.php)
Previous exceptions
Undefined variable: products (0)
It should be $products
Hi.
I have a problem with sessions in Laravel 7. They don’t persist across the code.
For example, if a have a method called addToCart and I create a session within it, no problem with that, I can access to the session data and show it in a view. But when I try to call the session in another method I have called index, then it gives me NULL. Also, when I try to show the session in another view, it’s not possible, it gives me NULL too.
Do you know what could be the problem?.
Thanks,
Ciro.
This is a common problem in Laravel, which may be caused by cookie name try to change the cookie name in config/session.php to some unique name:
‘cookie’ => ‘unique_name’,
Hello thanks for the tutorial
Is there a demo link?
No i didn’t create a demo for this just download the source
Thank you
This is an excellent post. This is really helpful and for my business websites.
Thanks for the tutorial! but when I logout then login back the item disappear.
Because we store the items in the session to store them permanently consider storing them into database
hello sir thank you for the tutorial but I have a little mouse button my product is not displayed
it run smoothly .. thanks……
have github?
https://bitbucket.org/webmobtuts/shopping-cart-tutorial-laravel
Hi i got this error Property [photo] does not exist on this collection instance. (View: C:\Laravel_Project\Project2\resources\views\products.blade.php), im using php 7.4.11
Seems like you haven’t added a photo field in your products table
Illegal string offset ‘quantity’
Which line and file?
“message”: “The GET method is not supported for this route. Supported methods: PATCH.”,
when i am trying to update cart get this error on console.
The update route should be like that
Route::patch(‘update-cart’, ‘ProductsController@update’);
amazing ????
Thanks
delete method is not working
i like but can u share some tutorial about add to cart using cookie
May be in future lesson
file style.css tạo ở đâu hay nằm ở đâu ạ
what if i have single button for Add to Cart and a dropdownlist for products and now select a product and add to cart with a single button which is out of foreach loop.Now my gets last product id and add it in cart. Any suggestion for this
What do you mean “out of foreach loop”?
Is this secure? I mean you are saving price in session. Is user able to change session variables? If it is , how can we handle it?
Yes it’s secure however if you intend to build a fully ecommerce website it’s better to use database to save shopping cart items. Big websites such as Amazon save shopping cart data into db.
Good Work