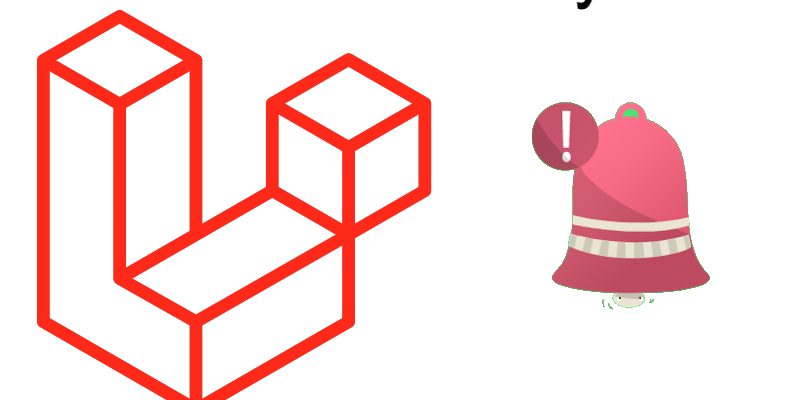
If you are looking for a way to display beautiful notifications in your laravel project, this package is for you /laravel-notify.
/laravel-notify package display a nice notification popup after every action you do in laravel controllers. The package notifications for success, errors, warnings and more.
Installation
Go to the github repo or run the following command:
composer require mckenziearts/laravel-notify
Register the service provider in config/app.php
. For Laravel versions 5.5 and beyond, this step can be skipped if package auto-discovery is enabled.
'providers' => [ ... Mckenziearts\Notify\LaravelNotifyServiceProvider::class ... ];
Publish the configuration file and assets:
php artisan vendor:publish --provider="Mckenziearts\Notify\LaravelNotifyServiceProvider"
Finally run this command to reload the composer dependencies:
composer dump-autoload
Â
Config
The config file located at config/notify.php. Here you customize many things. For example you can customize the dark mode to be used by updating the theme
 config, or add global variable NOTIFY_THEME
 on your .env file
'theme' => env('NOTIFY_THEME', 'dark'),
Also you can define custom user presets notifications as shown:
'preset-messages' => [ 'item-created' => [ 'message' => 'The item created successfully.', 'type' => 'success', 'model' => 'connect', 'title' => 'Item Created', ] ],
Usage
In your blade template in the head add the styles with:
@notifyCss
In the footer add the scripts with:
@notifyJs
Then in order to show the notification add the necessary component at the bottom:
For laravel < 7
@include('notify::components.notify')
For laravel >= 7 you can use the tag syntax:
<x:notify-messages />
Example layout.blade.php
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> @notifyCss </head> <body id="page-top"> @yield('content') @include('notify::messages')    // Laravel 7 or greater    <x:notify-messages /> @notifyJs </body> </html>
A typical scenario to fire the notification:
public function store() { notify()->success('Category created successfully!'); return redirect()->back(); }
Here we are using the helper function notify().
There are many types of notifications:
- toast:
notify()->success('Resource added successfully')
- connectify:
connectify('success', 'Connection Found', 'Success Message Here')
- drakify:
drakify('success') // for success alert or drakify('error') // for error alert
- smilify:
smilify('success', 'You are joined successfully')
- emotify:
emotify('success', 'Item updated with success')
If you want to make a shared notification that you can use across many modules without repetition, you can use a predefined preset in the config file like so:
notify()->preset('item-created')
To override the preset in certain places, pass an array that has the key of the attribute that you want to override:
notify()->preset('item-created', ['title' => 'Product created'])