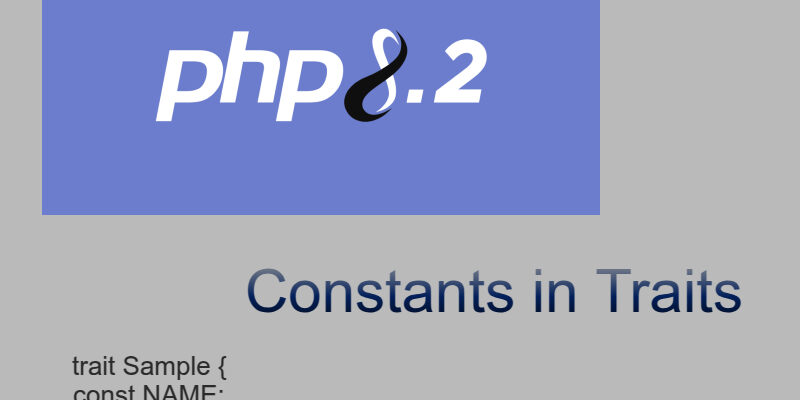
Using traits in PHP offers great benefits for code reuse. With the launch of PHP 8.2, developers have more powerful features at their fingertips. One notable enhancement is the ability to define constants in traits. This article will explain how to use constants within traits effectively, providing clear examples and best practices.
To define a constant in a trait, follow this syntax:
trait SampleTrait { const EXAMPLE_CONSTANT = 'Hello, World!'; }
In this example, EXAMPLE_CONSTANT
 is a constant that can be accessed wherever the trait is used.
Visibility and Scope
Constants within traits can have different visibility levels:
- Public:Â Accessible everywhere.
- Protected:Â Accessible in the trait and its subclasses.
- Private:Â Accessible only within the trait itself.
Here’s how to define them:
trait SampleTrait { public const PUB_CONSTANT = 'Public'; protected const PROT_CONSTANT = 'Protected'; private const PRIV_CONSTANT = 'Private'; }
class SampleClass { use SampleTrait; } echo SampleClass::PRIV_CONSTANT; // Uncaught Error, Cannot access private constant
Naming Best Practices
When naming constants, consider these guidelines:
- Use uppercase letters for constants.
- Separate words with underscores (_).
Accessing Constants
You can access constants defined in a trait directly in the methods of a class using that trait. Here’s how:
trait SampleTrait { const CONSTANT_VALUE = 100; public function showConstant() { return self::CONSTANT_VALUE; } } class SampleClass { use SampleTrait; } $instance = new SampleClass(); echo $instance->showConstant(); // Outputs: 100
Error Handling
Accessing undefined constants can throw errors. Use checks using PHP defined() function to check for constant:
if (defined('self::UNDEFINED_CONSTANT')) { echo self::UNDEFINED_CONSTANT; } else { echo 'Constant not defined.'; }
Overriding Trait Constants
Traits constants cannot be overridden directly in a class, which helps maintain their values. If customization is needed, consider using methods instead:
trait EquipmentTrait { const EQUIPMENT_TYPE = 'Basic'; public function getEquipmentType() { return static::EQUIPMENT_TYPE; } } class AdvancedEquipment { use EquipmentTrait; public function getEquipmentType() { return 'Advanced'; } } $equipment = new AdvancedEquipment(); echo $equipment->getEquipmentType(); // Outputs: Advanced
Example: Database Configuration
Using constants in a trait for database connections:
trait DatabaseConfig { const DB_NAME = 'my_database'; const DB_USER = 'root'; const DB_PASS = 'password'; } class Database { use DatabaseConfig; public function connect() { return "Connecting to " . self::DB_NAME . " as " . self::DB_USER; } } $db = new Database(); echo $db->connect();
Example: Defining UI Elements
Constants can define reusable UI elements, improving consistency:
trait UIConstants { const BUTTON_COLOR = 'blue'; public function getButtonColor() { return self::BUTTON_COLOR; } } class Button { use UIConstants; public function render() { return "Button color is " . $this->getButtonColor(); } } $button = new Button(); echo $button->render(); // Outputs: Button color is blue