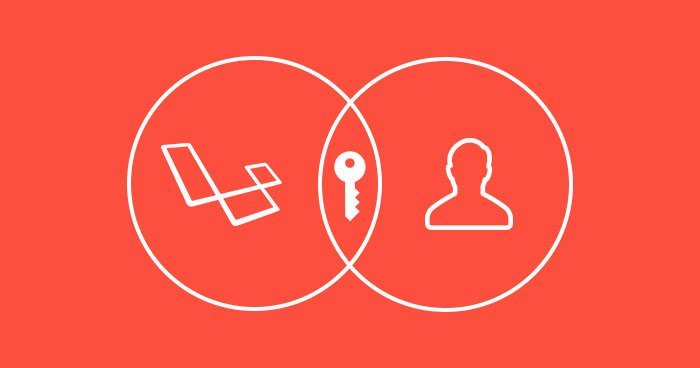
In this tutorial i will demonstrate how to create token based authentication with laravel 5.5. Token authentication normally used in when have some sort of Apis and a need a way to authenticate users when they interact with your apis.
Overview
The traditional process of interacting with a website is that you login from the login page. Next, you perform you desired actions and then log out. However, in the case of REST API, the process is quite different. The traditional procedure does not work in the case of RESTful APIs because the methods used on login page does not make any sense. You need to use api_token instead.
So you have to append the api_token with every api request and the request is authenticated. we will implement this in laravel with the help of the Passport library.
For demonstration purposes we will create a simple application in laravel in which can register and login.
Requirements
- Laravel 5.5
- PHP 7.0 or higher
- MySQL
Laravel Passport
composer require laravel/passport=~4.0
After install successfully Passport package in our Laravel application i need to set their Service Provider. so, open your config/app.php file and add following Line in the ‘providers’ array.
Laravel\Passport\PassportServiceProvider::class,
create the Database
Now go to mysql and create a new database then in the project root, you will find the .env and config/database.php files. Add the database credentials (username, DB name, and password) to setup the database and allow the Laravel app access it.
Run the migrations
After set providers array now run the migration command
Php artisan migrate
Install Laravel Passport
After Migrations of tables is successfully completed now run the install passport command
php artisan passport:install
Configuration of  Passport
After install of Passport , next move to next Three step to configure it .
First open app/User.php file and update with following code.
<?php namespace App; use Laravel\Passport\HasApiTokens; use Illuminate\Notifications\Notifiable; use Illuminate\Foundation\Auth\User as Authenticatable; class User extends Authenticatable { use HasApiTokens, Notifiable; /** * The attributes that are mass assignable. * * @var array */ protected $fillable = [ 'name', 'email', 'password', ]; /** * The attributes that should be hidden for arrays. * * @var array */ protected $hidden = [ 'password', 'remember_token', ]; }
Second go to the app/Providers/AuthServiceProvider.php  and update following code
<?php namespace App\Providers; use Laravel\Passport\Passport; use Illuminate\Support\Facades\Gate; use Illuminate\Foundation\Support\Providers\AuthServiceProvider as ServiceProvider; class AuthServiceProvider extends ServiceProvider { /** * The policy mappings for the application. * * @var array */ protected $policies = [ 'App\Model' => 'App\Policies\ModelPolicy', ]; /** * Register any authentication / authorization services. * * @return void */ public function boot() { $this->registerPolicies(); Passport::routes(); } }
Third update the config/auth.php with following code
'guards' => [ 'web' => [ 'driver' => 'session', 'provider' => 'users', ], 'api' => [ 'driver' => 'passport', 'provider' => 'users', ], ],
Run Laravel AuthÂ
php artisan make:auth
Set API Route
Go in routes folder and open api.php
Route::middleware('auth:api')->get('/user', function (Request $request) { return $request->user(); }); Route::post('login', 'Api\PassportController@login'); Route::post('register', 'Api\PassportController@register'); Route::group(['middleware' => 'auth:api'], function(){ Route::post('get-details', 'Api\PassportController@getDetails'); });
Create the Controller
php artisan make:controller Api/PassportController
Now go to app/Http/Controllers/Api and open PassportController.php
namespace App\Http\Controllers\Api; use Illuminate\Http\Request; use App\Http\Controllers\Controller; use App\User; use Illuminate\Support\Facades\Auth; use Validator; class PassportController extends Controller { public $successStatus = 200; /** * login api * * @return \Illuminate\Http\Response */ public function login(){ if(Auth::attempt(['email' => request('email'), 'password' => request('password')])){ $user = Auth::user(); $success['token'] = $user->createToken('MyApp')->accessToken; return response()->json(['success' => $success], $this->successStatus); } else{ return response()->json(['error'=>'Unauthorised'], 401); } } /** * Register api * * @return \Illuminate\Http\Response */ public function register(Request $request) { $validator = Validator::make($request->all(), [ 'name' => 'required', 'email' => 'required|email', 'password' => 'required', 'c_password' => 'required|same:password', ]); if ($validator->fails()) { return response()->json(['error'=>$validator->errors()], 401); } $input = $request->all(); $input['password'] = bcrypt($input['password']); $user = User::create($input); $success['token'] = $user->createToken('MyApp')->accessToken; $success['name'] = $user->name; return response()->json(['success'=>$success], $this->successStatus); } /** * details api * * @return \Illuminate\Http\Response */ public function getDetails() { $user = Auth::user(); return response()->json(['success' => $user], $this->successStatus); } }
Testing The App
Finally, run the php artisan serve command and check application
Next to test our apis i will use Postman as a restful client.