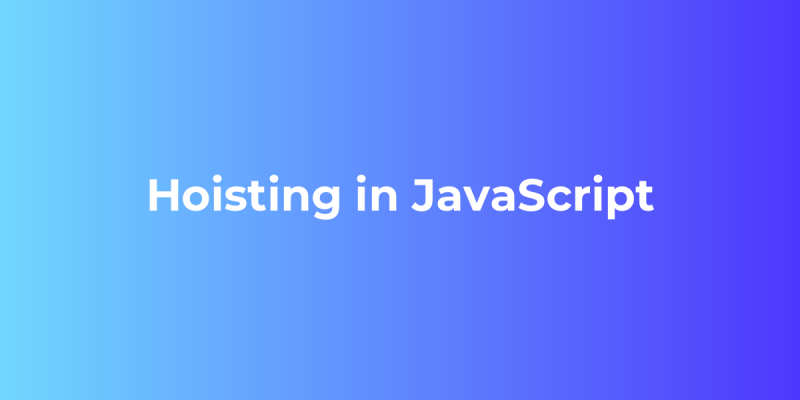
In this tutorial we look at a feature that you may already encountered a lot which Javascript Hoisting in other means calling code before actual code declaration.
You may have written javascript code before like this one:
sayMessage('Hello world'); function sayMessage(msg){ console.log(msg); }
If you run the above code it will works without any problems although you call the function before the function declaration and this interferes with the programming concepts which say that execution runs in sequence so this is hoisting in javascript.
So, what is happening here?
Most commonly, people will explain hoisting as declarations being moved to top of your code. While this is what appears to be happening, it’s important to understand exactly what is going on. You see, your code isn’t moving anywhere. It isn’t magically being moved to the top of the file. What’s actually happening is that your function and variable declarations are added to memory during the compile phase.
In the example above, because our function declaration was added to memory during the compile stage, we’re able to access and use it in our code above where it is typed.
Hoisting with variables
Hoisting with variables works in the same way as functions, look at this example:
var a = 3; console.log(a); // 3
However, what if we declare our variable at the bottom of our code?
a = 3; console.log(a); var a; // 3
As you can see, the above example logs 3
.
What about this example, where we declare and initialize our variable at the bottom of our code?
console.log(a); var a = 3; // undefined
Here, this example is the first time we get something unexpected. We expected 3
, but instead, undefined
is logged.
Why is this? It’s because JavaScript only hoists declarations. Initializations are not hoisted.
If we declare and initialize a variable, say var a = 3;
, only the var a;
portion (the declaration) is going to be hoisted. The a = 3;
(the initialization) is not hoisted and therefor not added to memory.
Remember, when we declare a variable but don’t initialize it, the variable is automatically set as undefined
. So lets look back at our final example. In the code below, only the var a;
will be hoisted:
console.log(a); var a = 3; // undefined
In fact, the above code yields the same result as writing it like this:
var a; console.log(a); a = 3; // undefined
Recommendations before using Hoisting
Because of hoisting, it’s considered a best practice to always declare your variables at the top of their respective scopes. This way there are no undesirable effects. You should also always try to initialize variables when you declare them. This will provide cleaner code and help avoid undefined
variables.