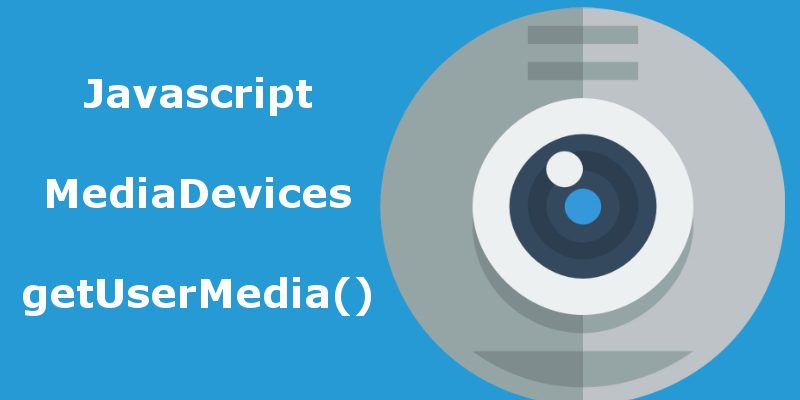
In this post i will show a simple snippet on how to access webcam in your browser using the javascript MediaDevices interface and getUserMedia() function.
People who tried to access webcams recently tended to install a third party flash plugin to add webcam support in browsers but as we know that flash plugins does not seem to provide the robust solution on how to customize things with code. Fortunately for this purpose a new Api added to Javascript to enable developers to access media devices which is the MediaDevices interface.
The MediaDevices interface incorporates some methods and apis to deal with media devices more easily such as webcams so for example you can takes photos, record videos, detecting when device attached or detached, get the list of media devices attached and more.
Retrieving the list of media devices
In this example we use the mediaDevices.enumerateDevices() function to get the list of media devices. This function return a promise which need to either resolved or rejected.
// check for mediaDevices.enumerateDevices() support if (!navigator.mediaDevices || !navigator.mediaDevices.enumerateDevices) { console.log("enumerateDevices() not supported."); return; } navigator.mediaDevices.enumerateDevices() .then(function(devices) { devices.forEach(function(device) { console.log(device.kind + ": " + device.label + " id = " + device.deviceId); }); }) .catch(function(err) { console.log(err.name + ": " + err.message); });
When running this script i suppose that your browser support the enumerateDevices() function it will show some output like this:
The resulting output show a list of input and output devices with each device have the deviceId, groupId, kind whether this an audio or video device and the label. In my case there is one videoinput which is my labtop camera.
No we have a camera let’s try to access that camera with javascript and take some photos. For this we will use another function in the MediaDevices interface which is the getUserMedia().
getUserMedia()
The getUserMedia() function requests a user permission to enable or disable the media device to be ready for use for example to allow or disallow the camera. The function return a promise and and once the user grants permission the Promise then() function is fired and the device is turned on. Else if the user disallow the request then the Promise catch() function is fired and the device turned off.
The function also take one parameter which an object that represent a constraints to apply. For example we can request that the taken photo to be of specific width and height so the constraint object will be {width: value, height: value}. There is a lot of constraints to apply you can find them in mozilla developer website.
Access webcam example:
In this example we will use an html <video/> element to access the webcam like shown below.
<html> <head> <meta charset="utf-8" /> <title>Webcam</title> <style> video { border: 3px solid #ccc; background: green; width: 400px; height: 400px } </style> </head> <body> <button>Start camera</button> <br/> <video></video> <script> if (!navigator.mediaDevices || !navigator.mediaDevices.getUserMedia) { console.log("getUserMedia() not supported."); return; } var btn = document.querySelector('button'); btn.onclick=function(e) { navigator.mediaDevices.getUserMedia({ audio: true, video: true }) .then(function(stream) { var video = document.querySelector('video'); if ("srcObject" in video) { video.srcObject = stream; } else { video.src = window.URL.createObjectURL(stream); } video.onloadedmetadata = function(e) { video.play(); }; }) .catch(function(err) { console.log(err.name + ": " + err.message); }); }; </script> </body> </html>
When running the script and click on the button the browser requests your permission if you click allow the camera will turn on and the stream will display in the video element. So let’s explain the code step by step.
First i added an html video element to display the stream coming from media device(camera). Then in the javascript i checked for navigator.mediaDevices.getUserMedia(), so we can proceed to the next step which is resolving the Promise returned by getUserMedia() function. The promise returned resolved to a MediaStream object which we can use to display in a video element as i did here:
var video = document.querySelector('video'); if ("srcObject" in video) { video.srcObject = stream; } else { // Avoid using this in new browsers, as it is going away. video.src = window.URL.createObjectURL(stream); }
Some browsers support the srcObject attribute in <video/> elements, for that reason i added this check otherwise if srcObject attribute not supported then it will fallback to the src attribute, but to display the stream in the src you have to make a little tweak using window.URL.createObjectURL(stream) which converts the stream to a url.
The parameter that i passed to getUserMedia() function is called a constraint object and this object identifies the resulting output so in the script i added this constraint {audio: true, video: true}, this constraint tells the media device to fetch the audio and video, now try to make audio: false you will hear nothing.
You can retrieve the supported useragent constraints using mediaDevices.getSupportedConstraints() function like in this snippet:
let supportedConstraints = navigator.mediaDevices.getSupportedConstraints(); console.log(supportedConstraints); // output autoGainControl: true ​ browserWindow: true ​ channelCount: true ​ deviceId: true ​ echoCancellation: true ​ facingMode: true ​ frameRate: true ​ height: true ​ mediaSource: true ​ noiseSuppression: true ​ scrollWithPage: true ​ viewportHeight: true ​ viewportOffsetX: true ​ viewportOffsetY: true ​ viewportWidth: true ​ width: true
Mobile phones front and back camera
For mobile phones there are a special constraint to use which is facingMode and can take one of these values “user” or “environment“. “user” is the front camera and “environment” is the back camera.
var constraints = { video: { facingMode: "user" } };
Note that to make the above code work on mobile phones you have to upload it to a remote server that supports “https” protocol.
To take photos from the webcam there are a tutorial here in the mdn website.