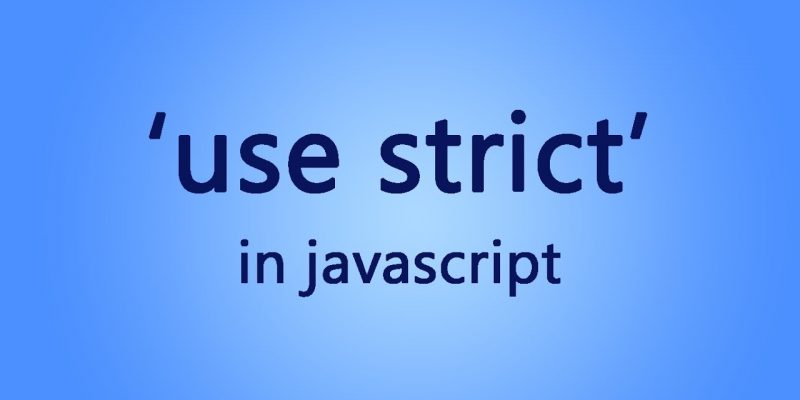
As we already know that javascript is a loosly typed language which means that you don’t need for example to define a variable before using it but recently a new EcmaScript 5 feature launched which can be used to do more syntax checking which is “Strict” mode.
“Strict” mode can easily statically-analyzable subset of EcmaScript which would be a good target for future versions of the language. Strict mode was also designed in the hope that developers who limit themselves to strict mode would make fewer mistakes and that the bugs they do make would manifest in more obvious ways.
Invoking strict mode
Strict mode applies to entire scripts or to individual functions. It doesn’t apply to block statements enclosed in {}
braces; attempting to apply it to such contexts does nothing.
Strict mode for scripts
To invoke strict mode for an entire script, put the exact statement "use strict";
(or 'use strict';
) before any other statements.
// Whole-script strict mode syntax 'use strict'; var v = "Hi! I'm a strict mode script!";
Strict mode for functions
Likewise, to invoke strict mode for a function, put the exact statement "use strict";
(or 'use strict';
) in the function’s body before any other statements.
function IamStrict() { // Function-level strict mode syntax 'use strict'; function nested() { return 'And so am I!'; } return "Hi! I'm a strict mode function! " + nested(); } function notStrict() { return "I'm not strict."; }
So How Javascript Behaves In Strict Mode
In “Strict” mode some rules must be followed otherwise the script will fail and these include:
Use of undeclared variable
In strict mode we cannot use an undeclared variable.
"use strict" a = 12; //this throws exception in strict mode. In non-strict mode a new property for window object is created.
delete operator
Delete operator is used to delete user defined object properties and array elements. If we try to delete anything other than user defined object properties or array elements we get an exception.
"use strict" var a = 78; window.b = 34; function print(){} window.c = function(){} delete a; //throws exception in strict mode. In non strict mode it returns false and program continues. 'a' is treated as a global variable not as an property of window object in both modes, so property operations are not allowed in variable a. delete window.a; //throws exception in strict mode. In non strict mode it returns false and program continues. delete window.b; //In both modes property b is deleted. delete Math.PI; //In strict mode it returns false as PI is pre defined. In strict mode exception is thrown. delete Object.prototype; //deleting an undeletable property in non-strict mode returns false. But in strict mode exception is thrown.
Multiple same declaration of property
In strict mode you cannot declare same object properties more than once. Otherwise it will throw exception.
var myObject = {property1:1, property2:2, property1:1}; //throws an exception.
Duplicate parameter names
In strict mode you cannot declare same parameter name more than once.
function print(value, value) {} //throws exception.
Octal numeric literals and escape characters are not allowed
Octal numeric literals and escape characters are not allowed in strict mode.
var testStrict = 010; // throws exception var testStrict = \010; // throws exception.
read-only and get-only Property
Writing to read-only and get-only properties of an object throws exception.
var testObjone = {}; Object.defineProperties(testObjone,"x",{value:0,writable:false}); testObjone.x = 3.14; // exception throws. var testObjtwo = {get x() {return 0} }; testObjtwo.x = 3.14; // exception thrown.
“eval” and “arguments”
We cannot use eval and arguments strings as variable, property or object names in strict mode.
var eval = 12; //In non-strict mode eval is overwritten. Exception thrown in strict mode. var arguments = 12;//Same behavior as above.
“with” statement
Use of “with” is not allowed in strict mode.
with (Math){x = sin(2)}; // throws an exception.
Reserved keywords
In strict mode we cannot use some words which are reserved for future version of JavaScript.
Reserved keywords are:
- implements
- interface
- package
- private
- protected
- public
- static
- yield
"use strict" var private = 12; //in non-strict mode this is allowed. In strict mode this throws an exception.
“this” keyword
“this” keyword inside global functions is undefined in strict mode.
"use strict" function checkforthis(){ console.log(this); //will print undefined. } checkforthis();
eval creating variables
eval function creates variables in the scope from which it was called. But in strict mode it doesn’t.
"use strict" eval("var q = 12"); console.log(q); //throws exception. q is not defined.
Benefits of strict mode
- Strict mode changes previously accepted “bad syntax” into real errors.
- In JavaScript mistyping a variable name creates a new global variable. In strict mode, this will throw an error, making it impossible to accidentally create a property of window variable.
- In normal JavaScript, a developer will not receive any error feedback assigning values to non-writable properties. And also any assignment to a non-writable property, a getter-only property, a non-existing property, a non-existing variable, or a non-existing object, will throw an error.