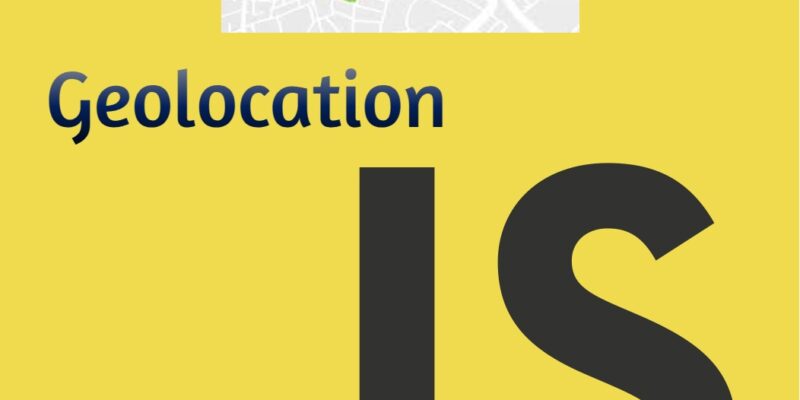
Geolocation brings countless benefits to web applications, enhancing user interaction and experience. With the rise of location-based services, understanding how to use the JavaScript Geolocation API becomes essential for developers.
The getCurrentPosition()
 Method
To obtain a user’s location, the getCurrentPosition()
method is provided. This method requests the current position of the device. Here’s a simple example:
navigator.geolocation.getCurrentPosition(successCallback, errorCallback); function successCallback(position) { console.log("Latitude: " + position.coords.latitude); console.log("Longitude: " + position.coords.longitude); } function errorCallback(error) { console.error("Error occurred. Error code: " + error.code); }
getCurrentPosition()
method accepts the successCallback
and errorCallback
arguments respectively. When the successCallback()
is executed you can get the user current position
object, as in this example we are retrieving the latitude and longitude of the user. You can learn more about the position object here.
The errorCallback
gets executed if an error happens while getting the user position.
There is also a similar function watchPosition()
the same as getCurrentPosition()
. However watchPosition()
executed every time the position updated perfect for situations to track user movement.
navigator.geolocation.watchPosition(success, error, options);
Handling Permissions and Errors
When accessing location data, browsers ask for user permission. It’s crucial to handle cases where the user denies access. Use the errorCallback
 function to manage these situations gracefully. Provide users with information about why the data is needed.
Working with Coordinates: Latitude, Longitude, and Accuracy
Once permission is granted, the API returns coordinates. The critical elements to note are:
- Latitude
- Longitude
- Accuracy
Let’s see a sample example creating a basic HTML structure for geolocation:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Geolocation Example</title> </head> <body> <h1>Your Location</h1> <button id="getLocation">Get Location</button> <p id="locationInfo"></p> <script src="script.js"></script> </body>5 </html>
In this html document we have a button to get the location data and display it in the div#locationInfo
.
The JavaScript Code: Requesting and Handling Location Data
script.js
document.getElementById("getLocation").addEventListener("click", function() { navigator.geolocation.getCurrentPosition(successCallback, errorCallback); }); function successCallback(position) { const locationInfo = document.getElementById("locationInfo"); locationInfo.textContent = `Latitude: ${position.coords.latitude}, Longitude: ${position.coords.longitude}`; } function errorCallback(error) { locationInfo.textContent = `Error occured while retreiving location ${error.message}`; }
A click event is attached to the button with id “getLocation
“, in the click handler function we call the getCurrentPosition()
as we learned previously. The successCallback()
prints the location info inside the div#locationInfo
, while the errorCallback()
prints an error message.
Improving Accuracy and Performance
You can pass a third argument to getCurrentPosition() which is the options object.
- Use theÂ
enableHighAccuracy
 option to get more precise data. - Adjust the timeout settings to allow users some leeway in how quickly they can share their location.
navigator.geolocation.getCurrentPosition(successCallback, errorCallback, { enableHighAccuracy: true, timeout: 5000 });
Geocoding and Reverse Geocoding: Converting Coordinates to Addresses
Geocoding allows developers to convert addresses into geographic coordinates, while reverse geocoding converts coordinates back into readable addresses. You can use services like Google Maps API for these tasks.
Let’s see a simple example while integrating geolocation data with google maps:
function initMap() { const map = new google.maps.Map(document.getElementById("map"), { center: {lat: 0, lng: 0}, zoom: 2 }); navigator.geolocation.getCurrentPosition(function(position) { const pos = { lat: position.coords.latitude, lng: position.coords.longitude, }; const marker = new google.maps.Marker({ position: pos, map: map, }); map.setCenter(pos); }); } initMap();