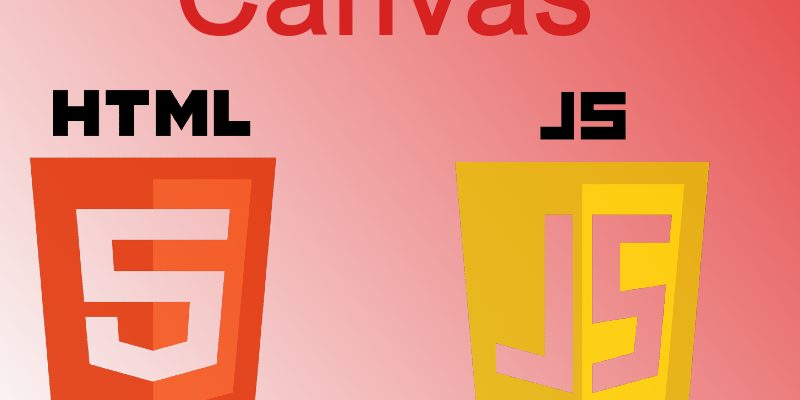
Canvas provides us with functions for displaying stroked and filled text as well as images, so in this article we will learn how to use these functions.
Drawing Text
Just like drawing stroked and filled rectangles we can draw stroked and filled text using the below functions:
- strokeText(text, x, y, [, maxWidth]): for drawing stroked text.
- fillText(text, x, y, [, maxWidth]): for drawing filled text.
Both functions accept the text to draw, the (x, y) coordinates and optional max width for drawing stretched or condensed text.
Stroked Text example
context.font = "30px arial"; context.strokeText("Html Canvas", 100, 50); context.font = "50px arial"; context.strokeText("Html5", 50, 150); context.strokeText("CSS3", 210, 150); context.strokeText("ES6", 150, 250);
Filled Text example
context.font = "30px arial"; context.fillStyle = "green"; context.fillText("Html Canvas", 100, 50); context.font = "50px arial"; context.fillText("Html5", 50, 150); context.fillText("CSS3", 210, 150); context.fillText("ES6", 150, 250);
To control the width of the text you can provide the max width parameter but be careful that this may led to loss the text quality:
context.fillText("Html Canvas", 100, 50, 100);
Text Styling
There are some properties that can control the text styling which are:
- font = value This property determine the css font family, we already saw in the examples above.
- textAlign= [start|end|left|right|center] Specifies the text alignment, default value is start.
context.textAlign = "left";
- textBaseline= [top|middle|bottom|hanging|alphabetic] Specifies the text baseline aligning or vertical alignment, default value is alphabetic.
context.textBaseline = "bottom";
Example moving text in circular path:
<canvas width="500" height="500" id="myCanvas"></canvas> <script> var context = document.getElementById("myCanvas").getContext("2d"); var x = 100; var y = 50; var angle = 0; var radius = 2; context.font = "30px arial"; setInterval(() => { context.clearRect(0, 0, 500, 500); context.strokeText("Html Canvas", x, y); x = x + radius * Math.cos(angle * (Math.PI / 180)); y = y + radius * Math.sin(angle * (Math.PI / 180)); angle++; }, 20); </script>
This example animates text using strokeText() along with clearRect() function. The clearRect() function used to clear the canvas, this function used mostly with canvas animations. To move the object in a circle all you have to do is updating the x, y coordinates of the object inside the setInterval() function as shown above. The formula to update the x, y coordinates:
x = x + radius * Math.cos(angle * (Math.PI / 180)); y = y + radius * Math.sin(angle * (Math.PI / 180));
Displaying Images in Canvas
To display images in canvas we can use the drawImage(image, x, y, width, height) function. The function accept an image instance, the (x,y) position, width and height. There are two ways to display the image into the canvas:
- The first method is rendering the image by referencing an html <img /> tag using document.getElementById().
- The second method if you don’t have an html <img /> tag and have only the image path you can create the image programmatically by using javascript Image() constructor.
Using document.getElementById():
context.drawImage(document.getElementById('source'), 0, 0, 210, 220);
Using an image instance from the Image() constructor:
var img = new Image(); // Create new img element img.addEventListener('load', function() { context.drawImage(img, 0, 0, 210, 220); }, false); img.src = 'image.png';
In this way you must listen for the load event on the image, then inside the load callback i invoked context.drawImage() and this an important point, if you attempt to call drawImage() directly it will not load the image.
It’s also possible to use image data uri’s like so:
img.src = "data:image/jpeg;base64/";
Capturing Media
In addition to displaying images from static image tags canvas powerful apis can be used to capture photos at particular time frames from javascript HTMLVideoElement or <video> tags.
Let’s see this example where we have a <video> element and button when clicked it will capture the current played frame:
<canvas width="400" height="400" id="myCanvas"></canvas> <video id="vid" style="width:500px;max-width:100%" controls=""> <source src="video.mp4" type="video/mp4"> Your browser does not support HTML5 video. </video> <button type="button" id="capture">Capture</button> <script> var context = document.getElementById("myCanvas").getContext("2d"); var video = document.getElementById("vid"); var btn = document.getElementById("capture"); btn.onclick = function() { context.drawImage(video, 0, 0, 400, 400); } </script>
As you see in this code i defined an html <video> element and a button. Then in javascript we get access to the video element using getElementById(). When clicking the button i listened on the onclick event and then i called context.drawImage() passing in the video instance.