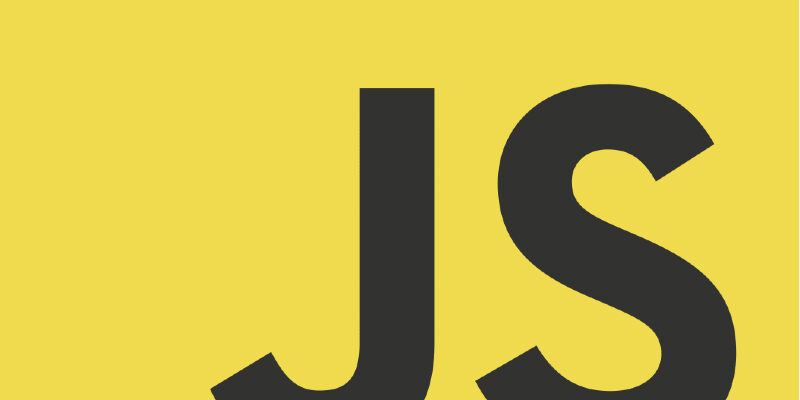
Media queries are a key part of responsive design in which it let’s us build responsive websites in css. However we can also manipulate media queries in Javascript using window.matchMedia() function.
Media queries in CSS let you apply styles based on device features. These include its width, height, or orientation.
CSS media queries use the @media
rule. With it, you can target styles to certain screen sizes or device types. For example, @media screen and (max-width: 768px)
applies styles only to screens smaller than 768 pixels wide. They ensure your website looks good on any device.
Javascript window.matchMedia()
window.matchMedia()
lets JavaScript check the status of media queries. With it, your website can react to screen size changes. it accepts a media query string and returns a MediaQueryList
object.
Basic usage:
const mediaQuery = window.matchMedia('(max-width: 768px)'); if (mediaQuery.matches) { // Do something if the media query matches console.log('Screen is smaller than 768px'); }
In this code if mediaQuery.matches
is true which means the screen is smaller than 768px. Note how we passed the media query string like we do in css.
You can think of window.matchMedia() like detecting window.clientWidth property.
The MediaQueryList
Object
The MediaQueryList
object has two key properties:
media
: The media query string.matches
: A boolean value. It shows if the media query currently matches.
Reacting to Media Query Changes: The MediaQueryList
Object
The MediaQueryList
object lets you listen for changes in media query status. By using the addEventListener
we can detect changes of screen size and implement particular logic.
const mediaQuery = window.matchMedia('(max-width: 768px)'); mediaQuery.addEventListener('change', (event) => { if (event.matches) { // Do something when the media query matches console.log('Media query matched!'); } else { // Do something when the media query no longer matches console.log('Media query no longer matches!'); } });
We accessed the matches
property in the event handler and determined the media query’s current state.
With this technique we can implement different logic using window.matchMedia, let’s see some examples below.
Dynamically Loading Content Based on Screen Size
Load different images based on the media query for mobile and desktop:
const mediaQuery = window.matchMedia('(max-width: 768px)'); function loadContent(matches) { if (matches) { // Load mobile-specific content document.getElementById('content').innerHTML = '<img src="mobile.png">'; } else { // Load desktop-specific content document.getElementById('content').innerHTML = '<img src="desktop.png">'; } } loadContent(mediaQuery.matches); mediaQuery.addEventListener('change', (event) => { loadContent(event.matches); });
Adjusting Navigation Menus for Mobile Devices
Toggle a mobile navigation menu based on screen size:
<style> #nav { background: #000; color: #fff; font-family: Arial, sans-serif; } #nav ul { list-style-type: none; margin-top: 5px; margin-bottom: 5px; } #nav ul li { display: inline-block; margin-inline-start: 5px; padding: 5px 3px; } #nav ul li a { display:block; color: #fff; text-decoration: none; } .mobile-menu ul li { display: block !important; } </style> <div id="nav"> <ul> <li> <a href="">Home</a> </li> <li> <a href="">About</a> </li> <li> <a href="">Services</a> </li> <li> <a href="">Contact</a> </li> </ul> </div> <script> const mediaQuery = window.matchMedia('(max-width: 768px)'); const nav = document.getElementById('nav'); function toggleMenu(matches) { if (matches) { nav.classList.add('mobile-menu'); } else { nav.classList.remove('mobile-menu'); } } toggleMenu(mediaQuery.matches); mediaQuery.addEventListener('change', (event) => { toggleMenu(event.matches); }); </script>
You can use JavaScript to add or remove CSS classes and apply custom css styles to those classes so that it looks different on mobile and desktop.
Using JavaScript Media Queries in React
Here’s how to use window.matchMedia()
in React components:
import React, { useState, useEffect } from 'react'; function MyComponent() { const [isMobile, setIsMobile] = useState(window.matchMedia('(max-width: 768px)').matches); useEffect(() => { const mediaQuery = window.matchMedia('(max-width: 768px)'); const handleChange = (event) => setIsMobile(event.matches); mediaQuery.addEventListener('change', handleChange); return () => mediaQuery.removeEventListener('change', handleChange); }, []); return ( <div> {isMobile ? ( <p>Mobile view</p> ) : ( <p>Desktop view</p> )} </div> ); } export default MyComponent;