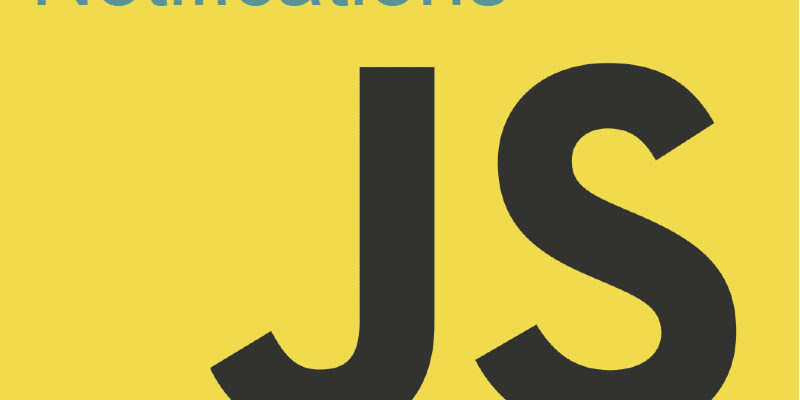
User engagement is crucial for web applications. Notifications can boost this interaction by keeping users informed in real-time. When users receive updates directly, they feel more connected to the app.
JavaScript notifications provide an instant way to communicate with users. They can relay important messages without interrupting the user experience. This flexibility allows businesses to engage effectively without being annoying.
Notifications rely on APIs that browsers support. The Javascript Notification API is used to configure and display desktop notifications to the user.
The Notification API: Permissions and Functionality
The Notification API allows developers to create notifications programmatically. Users must grant permission for notifications to appear. It’s a simple but crucial step in the process.
Always ask for permission at a natural moment. Avoid asking immediately when users enter the site. Instead, wait until a user takes an action that shows intent, like signing up for a newsletter.
Checking Notification Support:
if (!("Notification" in window)) { alert("Notifications not supported in this browser"); } else { // Notifications supported }
Once the notifications is supported, the next step is to show the notification to the user by checking for Notification.permission
key.
The Notification.permission
return a string with these values:
denied
— The user refuses to have notifications displayed.granted
— The user accepts having notifications displayed.default
— The user choice is unknown and therefore the browser will act as if the value were denied.
Basic Example:
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title>Js Notifications</title> </head> <body> <button id="show-notifications">Show notification</button> <script> var show_notification = document.querySelector("#show-notifications"); show_notification.addEventListener("click", function() { if (!("Notification" in window)) { alert("This browser does not support desktop notification"); } else { if (Notification.permission === "granted") { new Notification("Hello, World!"); } else if (Notification.permission !== "denied") { Notification.requestPermission().then(permission => { if (permission === "granted") { new Notification("Hello, World!"); } }); } } }); </script> </body> </html>
In this code we have a button that triggers notifications. So i registered an event listener for button click. Next we check for Notification availability in the browser using this line:
if (!("Notification" in window))
If notifications is supported then we show the notification by checking for Notification.permission
. If the permission is granted then we allowed to show the permission, otherwise we attempt to request the permission using Notification.requestPermission()
function.
The Notification.requestPermission()
requests permission from the user for the current origin to display notifications. The method returns a Promise
that fulfills with a string indicating whether permission was granted or denied:
Notification.requestPermission().then(permission => { if (permission === "granted") { new Notification("Hello, World!"); } });
When running this script you may not see the notification permission popup, just make sure the permissions is not blocked in the icon beside the address bar
Customizing Appearance: Icons, Titles, and Body Text
You can make your notifications stand out with customization. This can be done using the options argument. Set the icon, title, and body text as shown below:
const options = { body: "This is the message body", icon: "logo_big.png", image: "logo_big.png", badge: "logo_96x96.png", dir: "rtl", // Can be rtl or ltr silent: true, // for silent notifications (no sound) requireInteraction: true // notification should remain active until the user clicks or dismisses it }; new Notification("Custom Title", options);
Handling User Interaction: Click Events and Dismissal
Notifications can respond to user actions. Use event listeners to track when users interact. Here’s an example:
const notification = new Notification("Hello!", options); notification.onclick = function() { window.open("https://google.com"); };
Also you can use The show
event which fires when a Notification
is displayed:
notification.onshow = function() { console.log("Notification appeared"); }
The close
event of the Notification
interface fires when a Notification
is closed:
notification.onclose = function() { console.log("Notification closed"); }